Avoiding Spring Test Context Caching Pitfalls
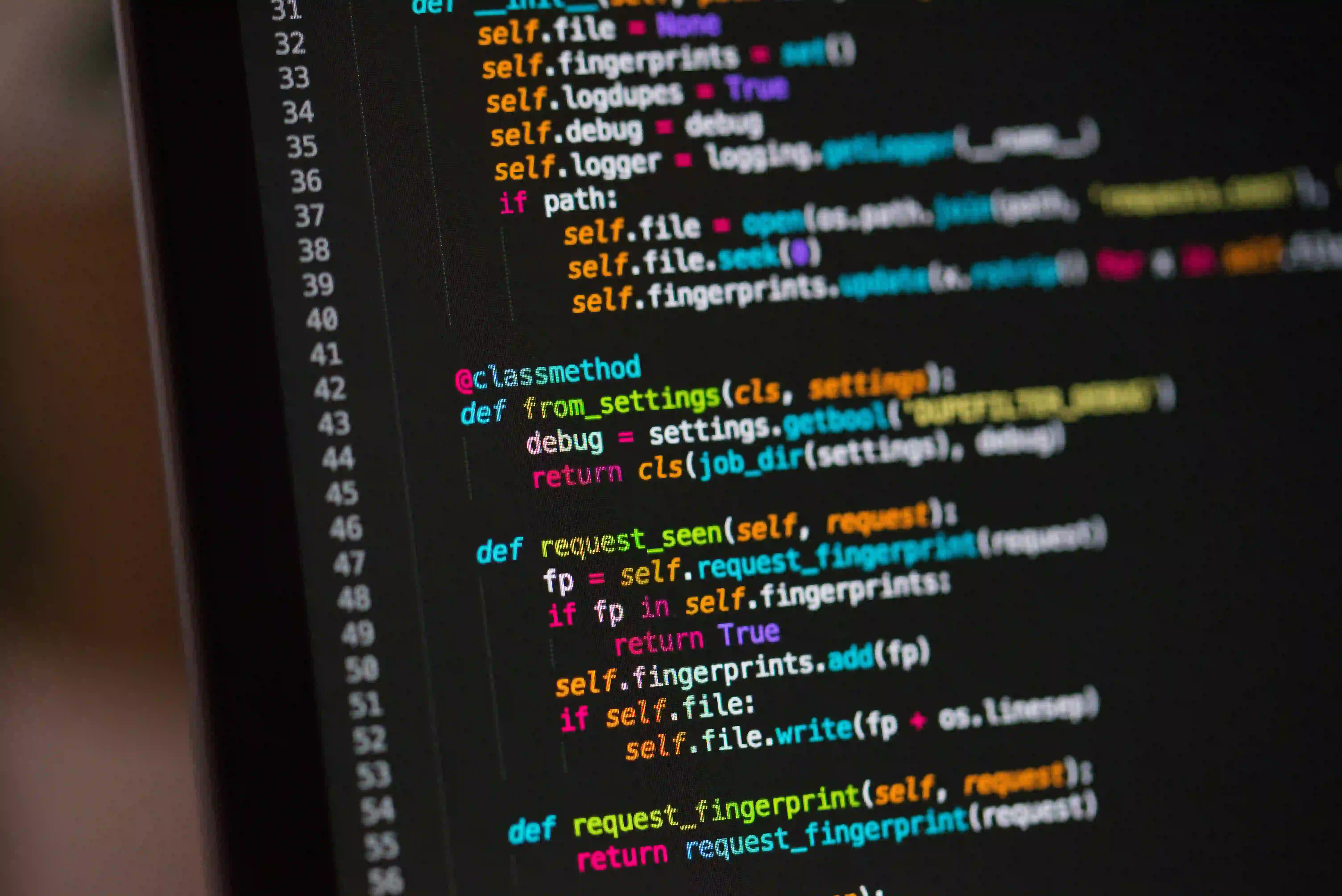
Avoiding Spring Test Context Caching Pitfalls
Setting the Stage
Testing plays a crucial role in ensuring the reliability and functionality of Spring applications. In the context of Spring, integration tests are instrumental in verifying that various components, such as controllers, services, and repositories, work seamlessly together. The Spring Test Context framework simplifies the process of writing and executing integration tests by managing the application context and providing utilities for testing Spring components.
However, within the context of Spring integration tests, the caching of test contexts, while a significant feature for improving test performance, can also be a double-edged sword. This article delves into the nuances of managing test context caching in Spring applications, highlighting common pitfalls and best practices to avoid them.
What is Test Context Caching?
In Spring, the test context caching mechanism is designed to improve the performance of integration tests by reusing application contexts across multiple test methods and classes. When a test class is run, the framework consults the cache to check if the corresponding application context already exists. If so, the existing context is reused, saving the overhead of creating it from scratch. This approach significantly reduces the time required to set up the application context for each test class, ultimately speeding up the execution of integration tests.
The test context caching mechanism essentially aims to strike a balance between the benefits of reusing application contexts and ensuring the isolation and independence of individual tests.
Why Manage Test Context Cache?
While test context caching offers substantial performance benefits, it can lead to unexpected outcomes if not managed effectively. One of the primary concerns is the potential for unintended context modifications, where changes made to the application state in one test method inadvertently affect the behavior of another. This can introduce false positives or negatives and compromise the reliability of the tests.
Moreover, inefficient context usage, such as overly broad context configurations, may diminish the advantages of caching by increasing the overhead associated with maintaining and reusing the application contexts. Additionally, a misinterpretation of the conditions that trigger the creation of new cached contexts can further exacerbate issues related to test isolation and performance.
Common Pitfalls and How to Avoid Them
Unintended Context Modifications
Consider the following scenario:
@SpringBootTest
class UserRegistrationServiceIntegrationTest {
@Autowired
private UserRepository userRepository;
@Test
void testUserRegistration() {
User user = new User("JohnDoe", "john@example.com");
userRepository.save(user);
// ...perform registration tests
}
@Test
void testUserDeletionAfterRegistrationFailure() {
// ...perform tests involving user deletion
}
}
In the example above, the UserRepository
bean is autowired into both test methods. If the testUserRegistration
method modifies the state of the repository by saving a user, it could inadvertently affect the behavior of the subsequent testUserDeletionAfterRegistrationFailure
method. To avoid this, the state changes made during the setup or execution of a test should be isolated to ensure each test method operates on a clean slate.
Inefficient Context Usage
When defining the application context for integration tests, it’s crucial to aim for minimal configurations tailored to the specific needs of the test scenarios. For instance, a test class focusing solely on service layer functionality should not include unnecessary controller or repository beans in its context configuration. By designing lean and purpose-specific context configurations, the benefits of caching can be maximized.
Misunderstanding Context Caching Keys
In the context of Spring integration tests, the caching of application contexts is influenced by factors such as the configuration of the test class, the environment, and the active profiles. Changes in these factors impact the caching decisions, and a deep understanding of how Spring determines the reuse or creation of cached contexts is vital for effectively managing test context caching.
Best Practices for Managing Test Context Cache
Clear Context Cache Space
The @DirtiesContext
annotation can be utilized to explicitly mark test methods or classes that require the context to be cleared or dirtied. While this annotation ensures a clean application context for the annotated tests, it comes with a trade-off in terms of performance, as the context creation overhead is reintroduced. Therefore, careful consideration must be given to its usage to maintain a balance between test isolation and performance.
Optimize Test Configuration
By adopting modular and reusable context configurations, test suites can substantially benefit from the caching mechanism. This allows the caching of specific context parts, minimizing the overhead of unnecessary context creation. Furthermore, advanced features such as @ContextConfiguration
can be employed to define custom context loaders and resource locations, enhancing the efficiency of the test configurations.
Understand Your Testing Needs
It's essential to understand that not all tests require an extensive cache of application contexts. Tailoring the caching strategies to the specific needs of the application and the team can lead to an optimal balance between performance and reliability. Conclusively, developers should perform a cost-benefit analysis to choose context caching strategies that align with their testing requirements.
Closing the Chapter
Effectively managing test context caching in Spring applications is critical for ensuring the reliability and efficiency of integration tests. By being mindful of unintended context modifications, optimizing test configurations, and comprehending the intricacies of caching mechanisms, developers can navigate the potential pitfalls associated with test context caching. Through clear directives and a deliberate approach, testing in Spring applications can be streamlined and scaled without compromising the integrity of the test suite.
In conclusion, the delicate balance between reusability and isolation in test context caching demands a deep and nuanced understanding of Spring’s testing mechanisms. By adhering to best practices and staying mindful of potential pitfalls, developers can harness the full potential of test context caching while safeguarding the reliability and effectiveness of their integration tests.
Further Reading
- Official Documentation: Spring Framework - Testing
- Book: "Testing Spring Boot Applications" by Andy Wilkinson and Phil Webb
- Tutorial: "Mastering Spring Framework 5" on Udemy
As you explore the realm of testing in Spring, refer to the official documentation for comprehensive insights into testing strategies and best practices. Additionally, books such as "Testing Spring Boot Applications" by Andy Wilkinson and Phil Webb offer in-depth explorations of testing methodologies in Spring. If you prefer online tutorials, "Mastering Spring Framework 5" hosted on Udemy provides valuable perspectives on testing in Spring applications.
With a foundational understanding of test context caching and the tools at your disposal, you can enhance your Spring testing expertise and fortify the reliability of your application through thoughtful testing strategies.