RxJava & Java 8: Solving Java EE 7 Testing Woes
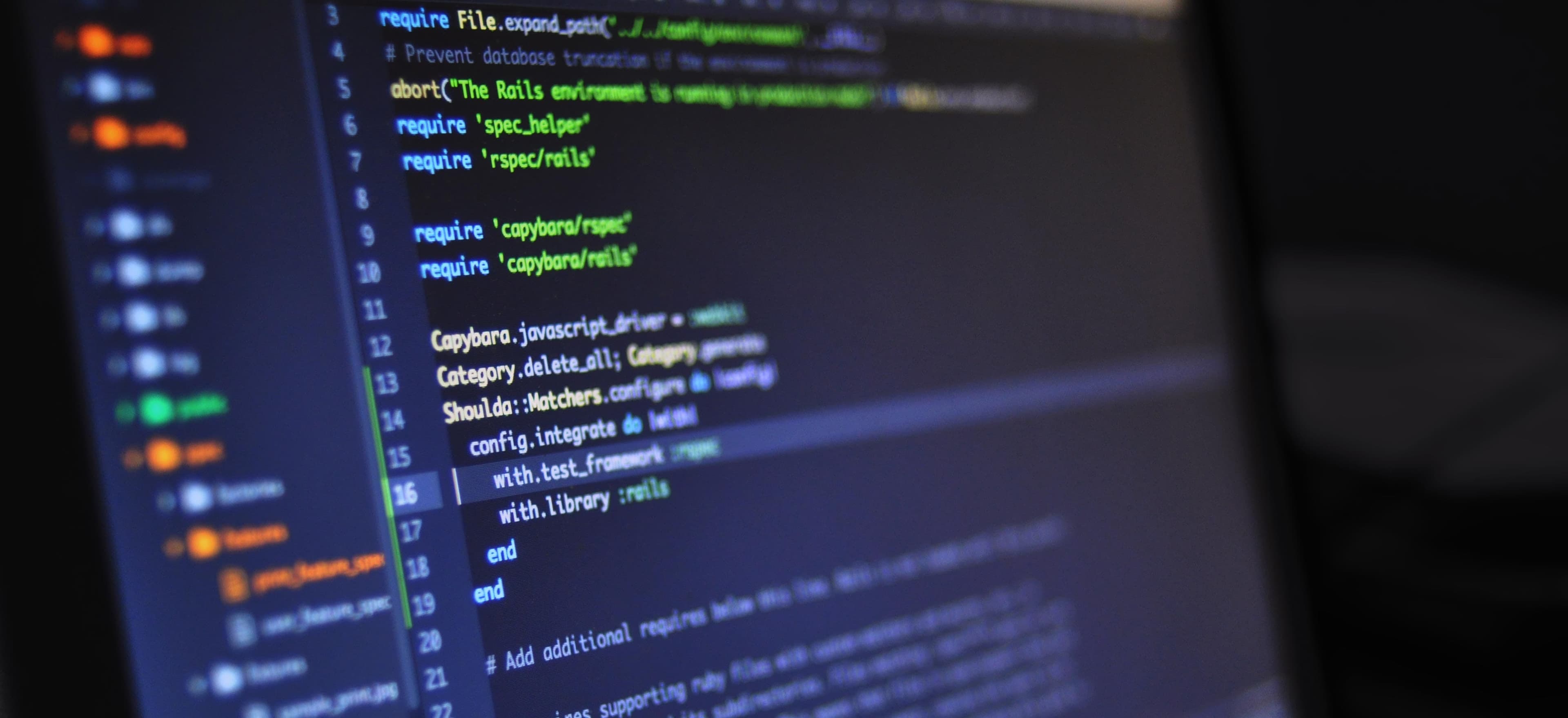
- Published on
RxJava & Java 8: Solving Java EE 7 Testing Woes
Introduction
Java EE 7 has long been a foundational technology for enterprise-grade applications, providing a robust set of APIs for building scalable and secure systems. However, with the increasing complexity of modern applications, testing Java EE 7 applications has become a daunting task for many developers. Asynchronous operations, integration test complexities, and mocking dependencies present significant challenges in the testing phase.
In this article, we will explore how RxJava, with its reactive programming paradigm, can serve as a potent solution for testing Java EE 7 applications. Additionally, we'll delve into the complementary nature of RxJava with Java 8 features, and how the two combined can streamline and simplify the testing process.
Understanding the Challenges with Java EE 7 Testing
Testing Java EE 7 applications presents multiple challenges for developers. Integration test complexity arises from the need to simulate a complete environment for testing components that rely on various Java EE 7 APIs. Moreover, mocking dependencies within the container-managed environment can be tricky and often requires the use of third-party libraries like Mockito.
The introduction of asynchronous operations in Java EE 7 also adds another layer of complexity to testing. Writing test scenarios for asynchronous behavior can be convoluted and error-prone, especially when dealing with complex sequences of events and multiple asynchronous calls.
Why RxJava?
RxJava, a reactive programming library for the JVM, offers a powerful paradigm for dealing with asynchronous operations. It provides a set of tools and abstractions for composing asynchronous and event-based programs using observable sequences. This makes it an ideal candidate for simplifying asynchronous programming and testing in Java applications, including those built on Java EE 7 technologies.
By leveraging RxJava, developers can write test scenarios that mirror complex asynchronous behavior in a clear and concise manner. The reactive nature of RxJava also enhances the readability and maintainability of test code, making it easier to understand and maintain test suites over time.
Integrating RxJava with Java EE 7 Applications
Integrating RxJava with Java EE 7 applications is relatively straightforward. Begin by adding the RxJava library as a dependency in the project configuration. Once integrated, developers can utilize RxJava's Observable
class to handle asynchronous operations, thus simplifying the testing of asynchronous code paths in Java EE 7 applications.
Code Snippet: Asynchronous Testing with RxJava
Let's take a look at an example code snippet that demonstrates testing an asynchronous operation with RxJava. In this scenario, we have an asynchronous method performAsyncOperation()
that returns an Observable
. We will write a test case to ensure that the method emits the expected value.
// Service class with an asynchronous method
public class AsyncService {
public Observable<Integer> performAsyncOperation() {
return Observable.create(emitter -> {
// Simulating an asynchronous operation
new Thread(() -> {
// Perform some asynchronous task
int result = performTask();
emitter.onNext(result); // Emit the result
emitter.onComplete(); // Complete the observable
}).start();
});
}
}
// Test class for asynchronous operation using RxJava
public class AsyncServiceTest {
@Test
public void testPerformAsyncOperation() {
AsyncService asyncService = new AsyncService();
TestObserver<Integer> testObserver = asyncService.performAsyncOperation()
.test(); // Create a test observer for the observable
testObserver.awaitTerminalEvent(); // Wait for the observable to complete
testObserver.assertResult(expectedResult); // Assert the emitted result
}
}
In this example, the use of RxJava's Observable
allows for a clear and concise representation of an asynchronous operation, simplifying the testing process. Additionally, Java 8's lambdas and Stream API can be utilized within the test cases, further enhancing the expressiveness and efficiency of the testing code.
Overcoming Mocking Challenges with Java 8
Java 8 introduces functional interfaces and lambda expressions, which provide a more succinct and expressive way to represent anonymous function implementations. When combined with testing frameworks like Mockito, Java 8 features enable simpler and more readable mocking in test scenarios.
For instance, using lambda expressions, developers can define behavior for mock objects in a more concise and declarative manner, enhancing the readability of test cases.
Code Snippet: Simplified Mocking with Java 8
Consider the following code snippet demonstrating simplified mocking using Java 8 features in a test scenario using Mockito:
// Mocking with Java 8 lambda expression
@Test
public void testSomeServiceMethod() {
SomeService mockService = mock(SomeService.class);
// Using Java 8 lambda to define mock behavior
when(mockService.someMethod(anyString())).thenAnswer(invocation -> "Mocked response");
// Invoke the method being tested
String result = testedComponent.methodUnderTest();
// Verify the method was called with specific arguments
verify(mockService).someMethod(expectedArgument);
}
In this snippet, the use of Java 8's lambda expression to define mock behavior with Mockito makes the test scenario more readable and easier to understand, especially when compared to the traditional anonymous inner classes used in earlier versions of Java.
Best Practices for Testing Java EE 7 Applications with RxJava and Java 8
When testing Java EE 7 applications with RxJava and Java 8, it's essential to follow certain best practices to ensure effective and efficient testing. Some key best practices include:
- Organizing test code in a clear and modular manner to facilitate maintenance and readability.
- Handling error scenarios and edge cases when dealing with asynchronous operations to ensure robust test coverage.
- Optimizing test execution time by leveraging parallel execution and efficient resource utilization.
By adhering to these best practices, developers can maximize the benefits of using RxJava and Java 8 for testing Java EE 7 applications.
Conclusion
In conclusion, RxJava and Java 8 provide powerful tools and paradigms for addressing the testing challenges faced by developers working with Java EE 7 applications. The reactive programming model of RxJava simplifies the testing of asynchronous operations, while Java 8 features enhance the readability and expressiveness of test scenarios, especially in mocking dependencies.
By incorporating these technologies and best practices, developers can streamline the testing process, leading to more robust and maintainable Java EE 7 applications. We encourage readers to explore the integration of RxJava and Java 8 in their testing strategies, leveraging the synergy between these technologies to achieve more efficient and effective testing outcomes.
Incorporate Relevant Links
Through this article, we've explored the intersection of RxJava and Java 8 in the context of testing Java EE 7 applications, providing real-world examples and best practices to equip intermediate Java developers with actionable insights to elevate their testing strategies.
Checkout our other articles