Boosting Web Apps: Mastering Async Servlets in Servlet 3
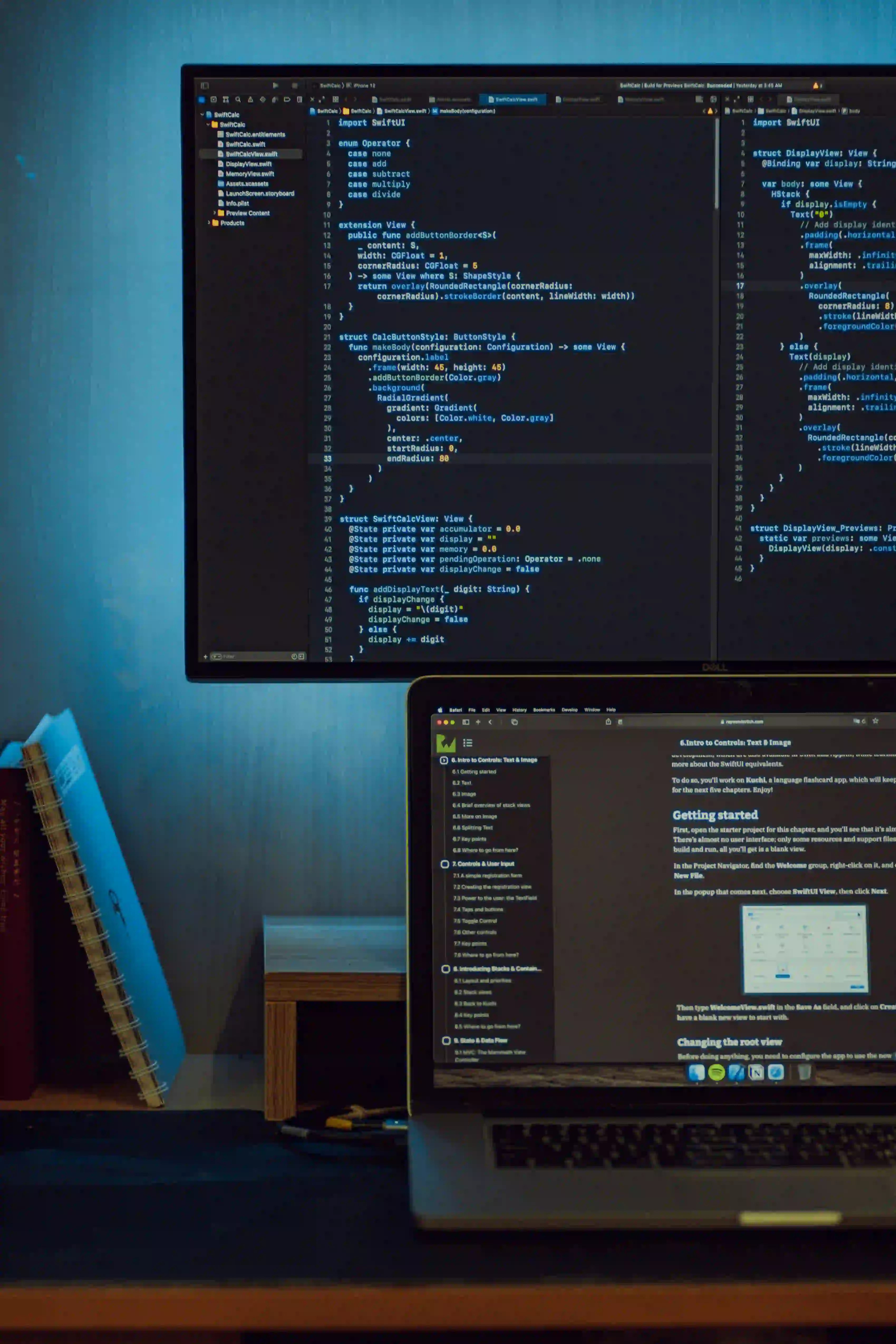
Mastering Async Servlets in Java Servlet 3
In today's world of web applications, performance is crucial. Users expect fast and responsive web services, which can be a challenge for developers. One way to improve the performance of web applications is by using asynchronous processing. In this blog post, we'll explore how to master async servlets in Java Servlet 3 to boost the performance of your web applications.
What are Async Servlets?
Traditional servlets in Java follow a synchronous request-response model, where each request is processed sequentially. This means that while a request is being processed, the server thread is blocked, waiting for the response to be generated. With async servlets, however, the server can process a request in a non-blocking manner, allowing the server thread to be freed up to handle other requests.
Why Use Async Servlets?
Using async servlets can significantly improve the performance and scalability of web applications. By allowing server resources to be used more efficiently, async servlets can result in better responsiveness and throughput, especially under heavy loads. This can lead to better user experience and lower infrastructure costs.
How to Use Async Servlets in Java Servlet 3
Java Servlet 3 introduced the capability for asynchronous processing, making it easier for developers to implement async servlets. Let's dive into the steps to master async servlets in Java Servlet 3.
1. Initializing Async Context
To initiate async processing in a servlet, we need to obtain the AsyncContext
from the ServletRequest
. This can be done by calling startAsync
on the ServletRequest
and obtaining the AsyncContext
from it. Here's an example:
@WebServlet("/asyncServlet")
public class AsyncServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response){
AsyncContext asyncContext = request.startAsync();
// Further processing using asyncContext
}
}
2. Processing in Async Context
Once the AsyncContext
is obtained, we can perform the processing asynchronously. This can involve tasks such as making asynchronous calls to external services, performing time-consuming computations, or streaming large data sets. The asynchronous processing should be performed within the AsyncContext
scope.
asyncContext.start(() -> {
// Asynchronous processing
});
3. Completion of Async Processing
After the asynchronous processing is completed, we need to complete the async context to send the response back to the client. This can be done by calling the complete
method on the AsyncContext
.
asyncContext.complete();
Best Practices for Async Servlets
When working with async servlets, there are several best practices to keep in mind:
-
Set Timeout for Async Requests: Always set a timeout for async requests to avoid potential resource leaks. This can be done using
asyncContext.setTimeout()
. -
Error Handling: Handle errors and exceptions gracefully when performing asynchronous processing.
-
Avoid Blocking Calls: Make sure that the asynchronous processing does not involve any blocking calls that can negate the benefits of async servlets.
-
Monitor and Tune: Monitor the performance of async servlets and tune the thread pool settings for optimal utilization of resources.
Wrapping Up
Mastering async servlets in Java Servlet 3 can significantly boost the performance of web applications. By leveraging asynchronous processing, developers can improve responsiveness and scalability, resulting in a better user experience. Asynchronous servlets are a powerful tool in the developer's arsenal for creating high-performance web applications.
In conclusion, understanding and applying async servlets in Java Servlet 3 can take your web application to the next level in terms of performance and scalability.
To learn more about async servlets and Java Servlet 3, you can refer to the official Java Servlet Documentation for in-depth information.
So, go ahead, master async servlets, and supercharge the performance of your web applications!