Overcoming Legacy Challenges with Modern CI/CD Tools
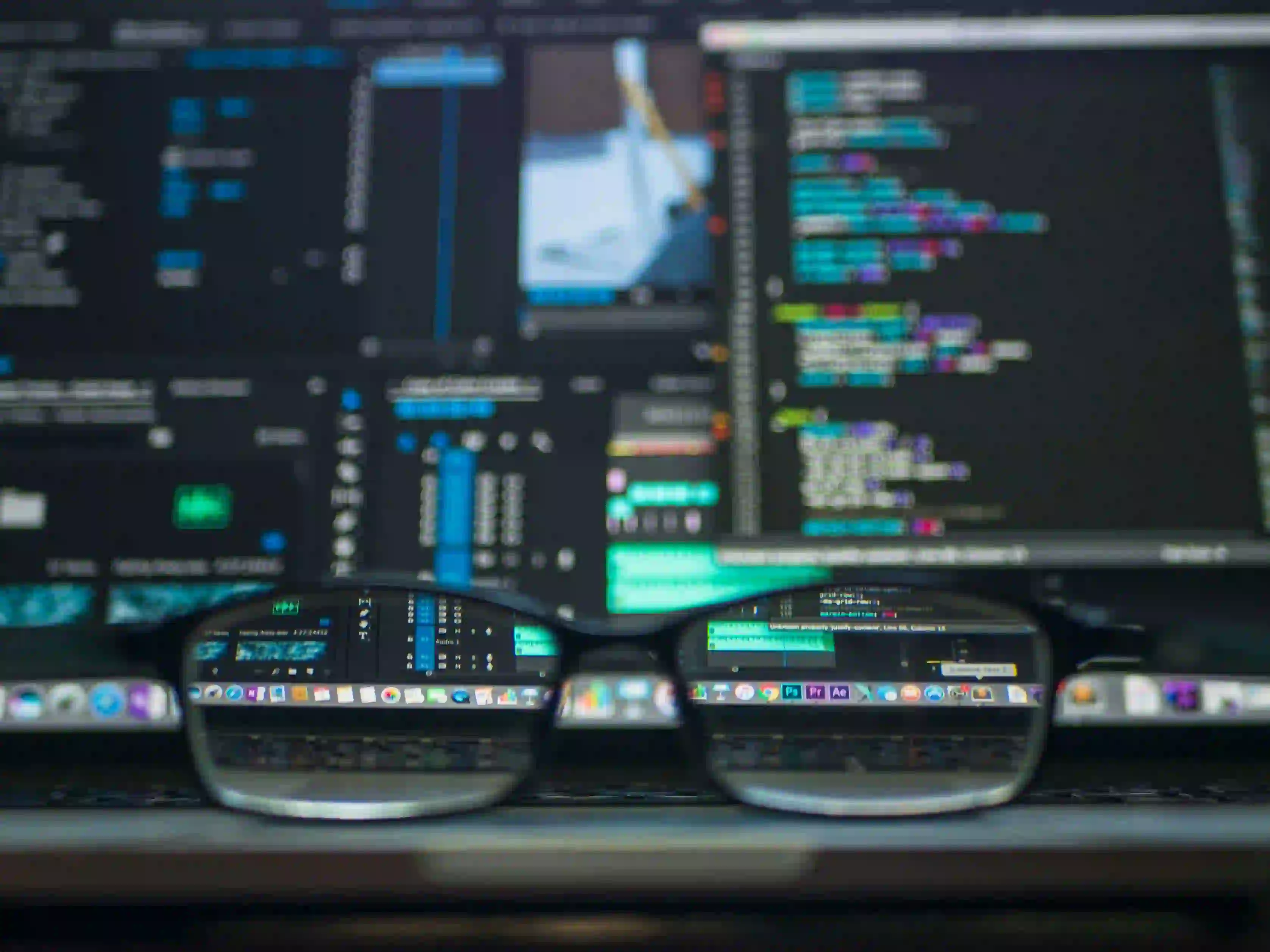
Overcoming Legacy Challenges with Modern CI/CD Tools
In the world of software development, maintaining and updating legacy systems can be a significant challenge. Legacy systems are often monolithic, tightly coupled, and lack modern development practices. However, with the right approach and tools, it is possible to overcome these challenges. In this article, we will discuss how modern CI/CD (Continuous Integration/Continuous Deployment) tools can help in overcoming legacy system challenges, and how Java, as a popular programming language in enterprise environments, can benefit from these practices.
Understanding Legacy Challenges
Legacy systems are often associated with outdated technologies, complex codebases, and lack of automated testing and deployment processes. These systems were not built with modern development practices in mind, making it difficult to update, test, and deploy changes without introducing regression issues.
The Role of CI/CD in Modernizing Legacy Systems
CI/CD practices have become a cornerstone of modern software development, enabling teams to automate the building, testing, and deployment of code changes. For legacy systems, the introduction of CI/CD practices can bring several key benefits:
-
Automation of Testing: Legacy systems often lack comprehensive test coverage, making it hard to make changes without breaking existing functionality. CI/CD tools enable automated testing, ensuring that new changes do not introduce regressions.
-
Streamlined Deployment Process: Manual deployment processes are error-prone and time-consuming. CI/CD pipelines automate the deployment process, reducing the risk of human error and accelerating the release process.
-
Incremental Updates: Legacy systems are often monolithic, making it challenging to roll out incremental updates. CI/CD encourages the practice of small, frequent releases, making it easier to manage and test the impact of changes.
Java and CI/CD
Java remains a widely used language in the enterprise, with many legacy systems and applications built on the Java platform. By integrating modern CI/CD practices with Java development, teams can effectively modernize and maintain their existing Java-based systems.
Java Build Automation with Gradle
One of the key components of CI/CD is build automation. Gradle, a popular build automation tool for Java, offers robust support for managing dependencies, building, and testing Java projects. Let's take a look at a simple build.gradle
file for a Java project:
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'com.google.guava:guava:30.1-jre'
testImplementation 'junit:junit:4.12'
}
test {
useJUnitPlatform()
}
In this example, we're using Gradle to define project dependencies (Guava and JUnit, in this case) and configuring the test task to use JUnit 5. This simple build.gradle
file demonstrates how Gradle streamlines the build and test process for Java projects, a crucial aspect of CI/CD.
Automated Testing with JUnit
Automated testing is a fundamental element of CI/CD. JUnit, the de-facto standard for unit testing in Java, enables developers to write and execute automated tests to ensure the correctness of their code. Here's a simple example of a JUnit test class:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyMathTest {
@Test
public void testAddition() {
MyMath math = new MyMath();
assertEquals(4, math.add(2, 2));
}
}
In this example, we're using JUnit to define a test case for a simple addition method. The assertEquals
method asserts that the expected result matches the actual result of the addition operation. By incorporating JUnit tests into the CI/CD pipeline, teams can gain confidence in the quality of their code and prevent regressions in legacy systems.
Continuous Deployment with Jenkins
Jenkins, an open-source automation server, plays a crucial role in enabling continuous deployment for Java applications. By defining Jenkins pipelines, teams can automate the entire deployment process, from building the application to deploying it to a production environment. Here's an example of a Jenkins pipeline for a Java application:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'gradle build'
}
}
stage('Test') {
steps {
sh 'gradle test'
}
}
stage('Deploy') {
steps {
sh 'ansible deploy.yml'
}
}
}
}
In this pipeline, we define three stages: build, test, and deploy. Each stage contains steps that automate the corresponding phase of the CI/CD process. Jenkins provides the flexibility to integrate with other tools and services, making it a powerful ally in modernizing the deployment process for legacy Java applications.
In Conclusion, Here is What Matters
Modern CI/CD tools have revolutionized the way software is developed, tested, and deployed. By embracing CI/CD practices and integrating them with Java development, teams can effectively overcome the challenges posed by legacy systems. The examples provided demonstrate how tools like Gradle, JUnit, and Jenkins can be leveraged to automate build processes, testing, and deployment for Java applications, ultimately leading to more reliable and maintainable systems.
In conclusion, modern CI/CD tools not only address the pain points of legacy systems but also pave the way for continuous improvement and agility in software development, ensuring that Java-based applications remain robust and efficient in today's fast-paced digital landscape.
By adopting modern CI/CD practices and leveraging Java's versatility, development teams can breathe new life into legacy systems, ultimately positioning them for long-term success in an ever-evolving technological landscape.
Additional Resources:
- The Benefits of Continuous Integration and Continuous Deployment
- Introduction to Jenkins Pipeline