Mockito Verification Woes: Solving Common Pitfalls
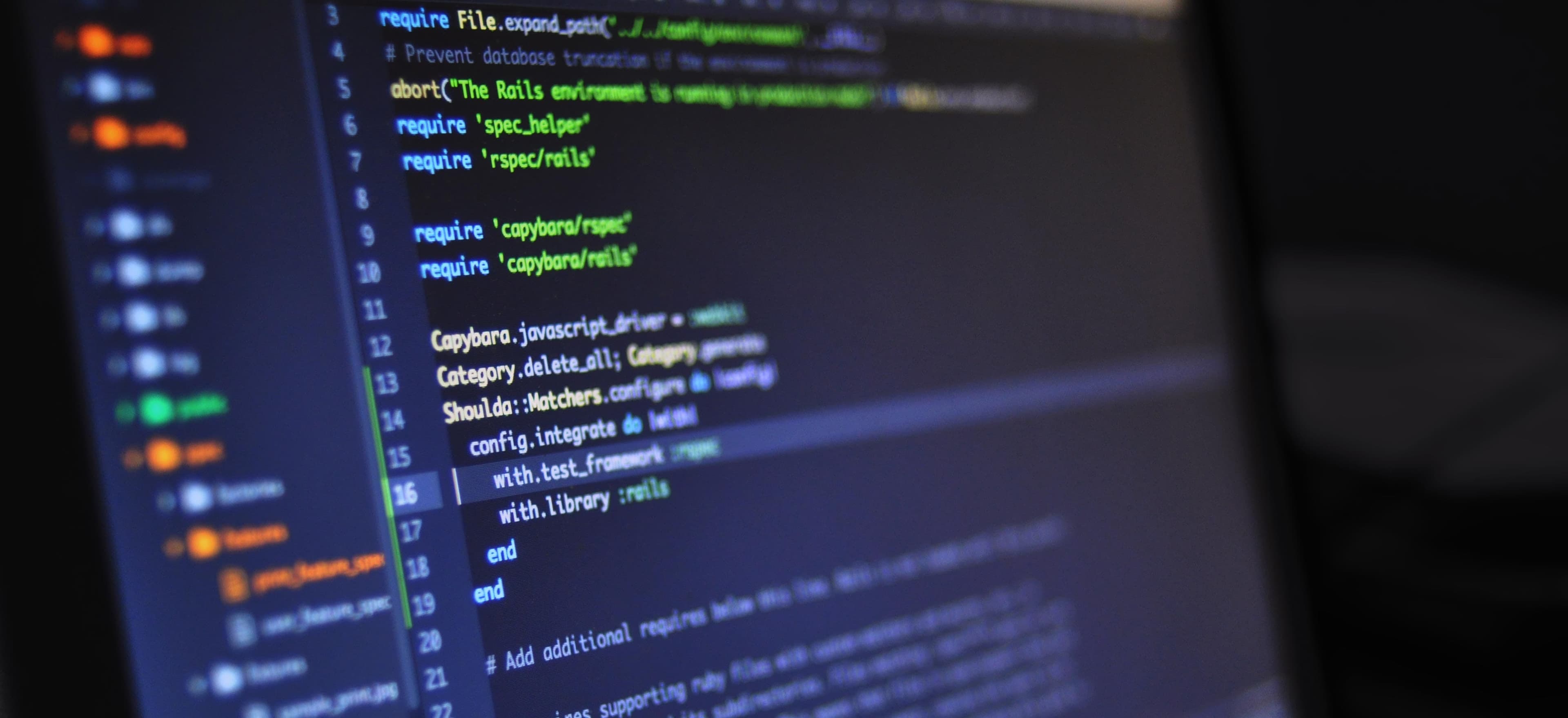
- Published on
Mockito Verification Woes: Solving Common Pitfalls
Mockito is a powerful Java framework for creating and using mock objects in unit tests. It allows developers to isolate the code under test by replacing dependencies with mock objects. One of the key features of Mockito is its ability to verify interactions between the code under test and its dependencies. However, verification in Mockito can sometimes be tricky, leading to common pitfalls that can frustrate developers. In this post, we'll explore some of these pitfalls and discuss how to solve them effectively.
Pitfall 1: Verifying the Wrong Method Call
One common mistake when using Mockito is verifying the wrong method call on a mock object. This can happen when the code under test invokes multiple methods on a dependency, and the developer inadvertently verifies the wrong method. To avoid this pitfall, it's essential to carefully identify the specific method call that needs to be verified.
// Incorrect verification
verify(mockDependency).doSomething();
// Correct verification
verify(mockDependency).doSomethingElse();
Why: Verifying the correct method call ensures that the expected interaction between the code under test and its dependency is properly validated.
Pitfall 2: Verifying the Wrong Number of Invocations
Another common issue is verifying the wrong number of invocations of a method on a mock object. Mockito allows developers to specify the exact number of times a method should be invoked, and failing to do so can lead to inaccurate verification results.
// Incorrect verification
verify(mockDependency, times(2)).doSomething();
// Correct verification
verify(mockDependency, times(1)).doSomething();
Why: Verifying the correct number of invocations ensures that the code under test is interacting with its dependencies as expected, preventing false positives in the test results.
Pitfall 3: Verifying Order of Method Calls
Verifying the order of method calls on mock objects is another area where developers can run into trouble. In complex scenarios with multiple dependencies and method invocations, it's crucial to ensure that the order of interactions is verified correctly.
// Incorrect verification
InOrder inOrder = inOrder(mockDependency1, mockDependency2);
inOrder.verify(mockDependency2).doSomething();
inOrder.verify(mockDependency1).doSomething();
// Correct verification
InOrder inOrder = inOrder(mockDependency1, mockDependency2);
inOrder.verify(mockDependency1).doSomething();
inOrder.verify(mockDependency2).doSomething();
Why: Verifying the order of method calls is essential for testing scenarios where the sequence of interactions between the code under test and its dependencies is significant.
Pitfall 4: Verifying Interaction with Real Objects
Mockito also allows developers to create partial mocks, which are mock objects that delegate to the real implementation for certain methods. When verifying interactions on partial mocks, it's crucial to ensure that the correct behavior is being validated.
// Incorrect verification on partial mock
verify(partialMockObject).doSomething();
// Correct verification on partial mock
verify(partialMockObject, times(1)).doSomething();
Why: Verifying interactions on partial mocks ensures that the expected behavior of the real implementations is properly validated, providing accurate test results.
Pitfall 5: Verifying Static Methods and Final Classes
Mockito has limitations when it comes to mocking static methods and final classes. It's essential to be aware of these limitations and use alternative approaches, such as PowerMock, when dealing with such scenarios.
Why: Understanding the limitations of Mockito and using appropriate alternatives prevents frustration and enables developers to effectively test code that relies on static methods and final classes.
Wrapping Up
In this post, we've explored some common pitfalls related to verifying interactions with mock objects using Mockito. By being mindful of these pitfalls and applying the correct verification techniques, developers can harness the full power of Mockito for effective unit testing. As a result, they can ensure that their code under test interacts with its dependencies as intended, leading to more robust and reliable software applications.
Remember, Mockito is a fantastic tool for unit testing, but it's essential to use it correctly to reap its full benefits and avoid potential pitfalls along the way.
For more in-depth understanding, check out this official Mockito documentation. Additionally, you can explore advanced verification strategies in the Mockito documentation to further enhance your unit testing skills using Mockito.
Happy testing!
Checkout our other articles