Scaling Issues with Netty and Karyon2 in Microservices
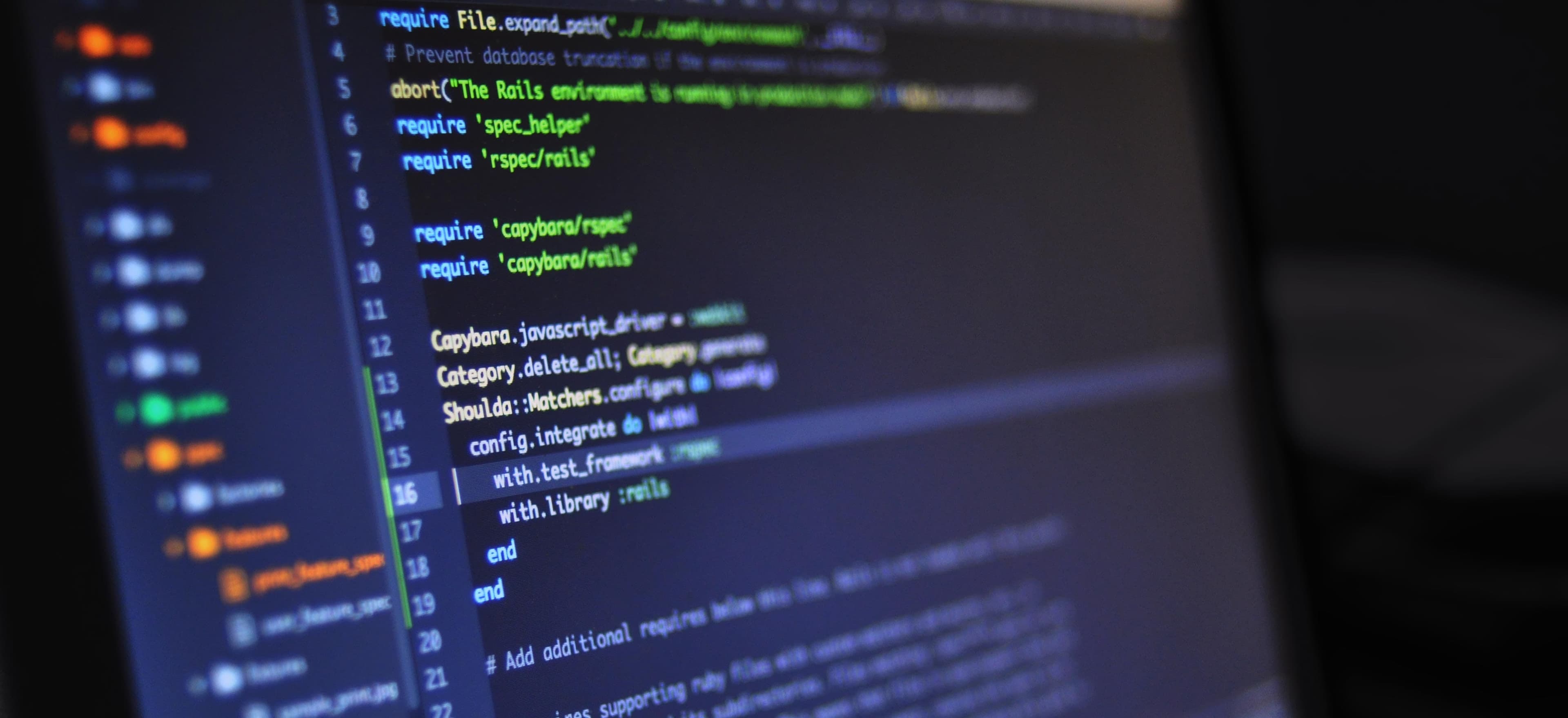
- Published on
Addressing Scaling Issues with Netty and Karyon2 in Microservices
Microservices have become a popular architectural style for building and deploying applications. In this approach, an application is composed of small, independently deployable services that work together. The microservices architecture offers benefits such as improved agility, scalability, and resilience. However, scaling microservices can present challenges, especially when dealing with communication between services.
In this blog post, we will focus on addressing scaling issues related to Netty and Karyon2 in microservices. Netty is a high-performance, non-blocking networking library for building low-level network applications in Java, while Karyon2 is a framework for building microservices using the Netflix stack. We will explore common scaling issues associated with Netty and Karyon2 in the context of microservices, and discuss strategies for mitigating these challenges.
Understanding the Scaling Issues
When dealing with microservices, one of the primary challenges is handling increased traffic and load as the number of service instances grows. Netty, being a powerful networking library, is commonly used in microservices for its performance and scalability. However, as the number of Netty-based microservices instances increases, managing communication between them can become complex and resource-intensive.
Karyon2, on the other hand, is built on top of Netty and provides a set of abstractions and utilities for building microservices. While Karyon2 simplifies the development of microservices, it's essential to address scaling issues that may arise when deploying and managing a large number of Karyon2-based microservices.
Mitigating Scaling Issues with Netty and Karyon2
1. Connection Pooling
One of the key strategies for mitigating scaling issues in Netty and Karyon2-based microservices is to implement connection pooling. Connection pooling allows services to reuse established network connections, reducing the overhead of creating new connections for each request. By implementing connection pooling with Netty, microservices can efficiently manage a large number of concurrent connections with minimal resource consumption.
Example of Connection Pooling with Netty:
ChannelPoolMap<InetSocketAddress, SimpleChannelPool> poolMap = new AbstractChannelPoolMap<InetSocketAddress, SimpleChannelPool>() {
@Override
protected SimpleChannelPool newPool(InetSocketAddress key) {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(new NioEventLoopGroup());
bootstrap.channel(NioSocketChannel.class);
bootstrap.remoteAddress(key);
return new FixedChannelPool(bootstrap, new SimpleChannelPoolHandler(), 10);
}
};
In this example, a ChannelPoolMap
is used to manage a pool of network connections to a specific remote address. By reusing the connections from the pool, the microservice can effectively handle a large number of concurrent requests.
2. Load Balancing
Another important aspect of mitigating scaling issues in microservices is implementing load balancing. Load balancing distributes incoming requests across multiple service instances, preventing any single instance from being overwhelmed with traffic. With Netty and Karyon2, implementing load balancing ensures that the workload is evenly distributed, improving the overall performance and scalability of the microservices architecture.
Example of Load Balancing with Karyon2:
public class MyHttpModule extends KaryonHttpModule {
@Override
protected void configureServer() {
bindRouter().toInstance(new MyRouter());
interceptTo(MyInterceptor.class);
loadBalancer(MyLoadBalancer.class);
}
}
In this example, the MyHttpModule
class configures the Karyon2 server with a custom load balancer, ensuring that incoming requests are evenly distributed across multiple instances of the microservice.
3. Asynchronous Communication
Adopting asynchronous communication patterns is crucial for handling scaling issues in Netty and Karyon2-based microservices. By leveraging asynchronous messaging and event-driven architectures, microservices can effectively manage high levels of concurrency and parallelism. Netty's non-blocking I/O model inherently supports asynchronous communication, enabling microservices to handle a large number of simultaneous connections without blocking threads.
Example of Asynchronous Communication with Netty:
Channel channel = // obtain a reference to the network channel
channel.writeAndFlush("Hello, World!");
In this example, the writeAndFlush
method sends a message asynchronously over the network channel, allowing the microservice to continue processing other tasks without waiting for the message to be sent.
A Final Look
Scaling Netty and Karyon2-based microservices involves addressing the challenges of managing communication and workload distribution as the number of service instances grows. By implementing strategies such as connection pooling, load balancing, and asynchronous communication, microservices can effectively handle scaling issues while maintaining high performance and resilience.
In conclusion, while Netty and Karyon2 offer powerful capabilities for building and deploying microservices, careful consideration of scaling issues is crucial for ensuring the success and stability of a microservices architecture. By addressing these challenges proactively, developers can build scalable and robust microservices that meet the demands of modern, cloud-native applications.
For further insight into scaling microservices and addressing performance challenges, consider exploring the Netflix Tech Blog for valuable resources and best practices.
Remember, achieving reliable, scalable microservices is an ongoing process, and staying updated with the latest trends and practices is vital in this rapidly evolving landscape.
Checkout our other articles