Tackling Microservice Complexity for Java Developers
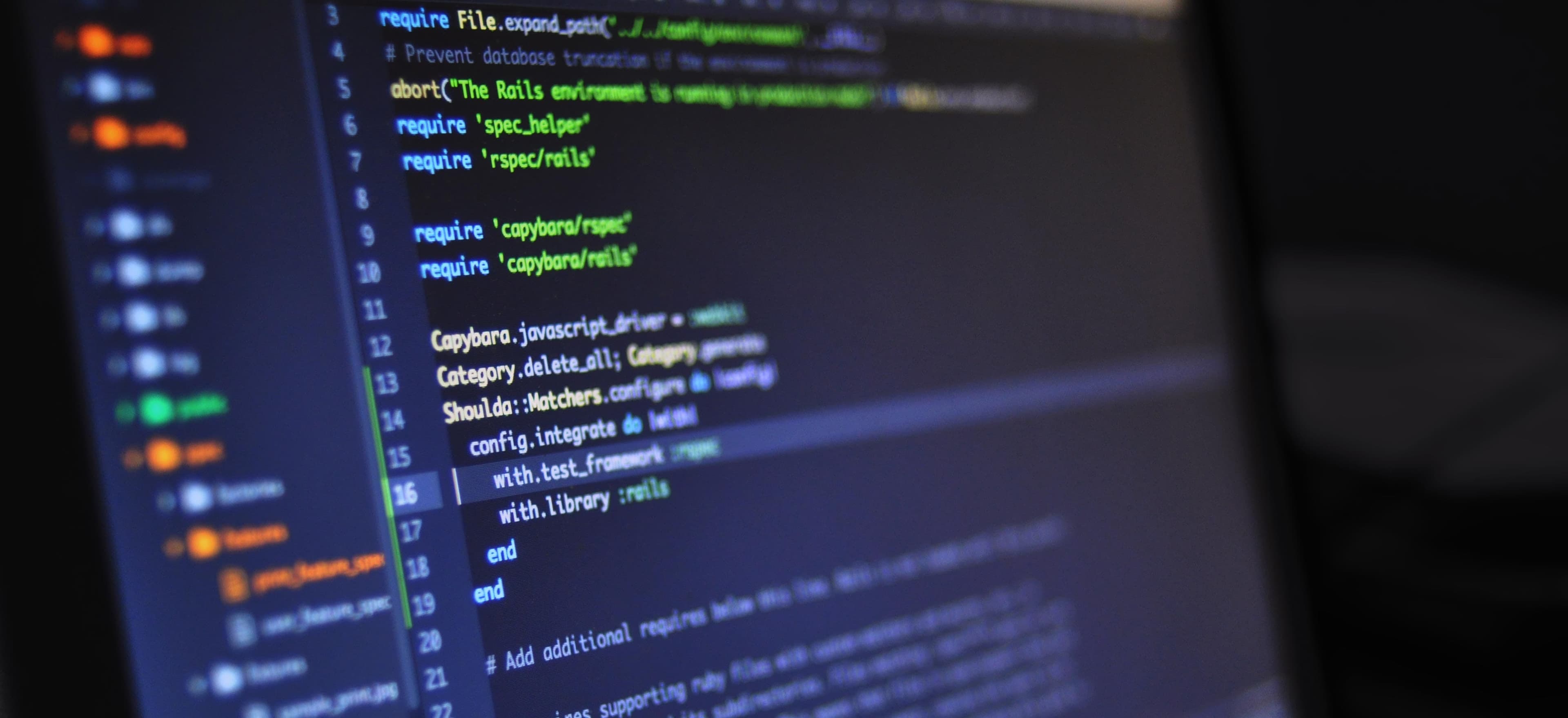
- Published on
Tackling Microservice Complexity for Java Developers
Microservices have become a popular architectural style for building and deploying applications. With the rise of microservices, Java developers are faced with the challenge of managing the complexity that comes with a distributed system. In this blog post, we will explore how Java developers can effectively tackle the complexities of microservices architecture.
Understanding Microservices
Before diving into the complexities, let's first understand what microservices are. Microservices is an architectural style that structures an application as a collection of loosely coupled services, which are independently deployable and scalable.
Embracing Asynchronous Communication
One of the biggest challenges in microservices architecture is managing inter-service communication. With synchronous communication, services can become tightly coupled, leading to cascading failures and increased complexity. Java developers can tackle this complexity by embracing asynchronous communication using message brokers like Apache Kafka or RabbitMQ.
Here's an example of asynchronous communication using Apache Kafka in Java:
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
producer.send(new ProducerRecord<String, String>("my-topic", "key", "value"));
producer.close();
In this example, we use Apache Kafka to publish messages to a topic asynchronously. This approach decouples services and reduces the complexity of synchronous communication.
Service Discovery and Load Balancing
Another complexity in microservices architecture is service discovery and load balancing. As the number of microservices grows, it becomes challenging to manage their addresses and balance the traffic. Java developers can tackle this complexity by utilizing service discovery tools like Netflix Eureka and load balancers like Ribbon.
Below is an example of service discovery using Netflix Eureka in Java:
@RestController
class ServiceInstanceRestController {
@Autowired
private DiscoveryClient discoveryClient;
@GetMapping("/service-instances/{applicationName}")
public List<ServiceInstance> serviceInstancesByApplicationName(
@PathVariable String applicationName) {
return this.discoveryClient.getInstances(applicationName);
}
}
In this example, we use Netflix Eureka to discover service instances by application name. This enables dynamic and scalable communication between microservices, reducing the complexity of managing service addresses.
Fault Tolerance and Resilience
Microservices are prone to failures, and Java developers need to tackle this complexity by building fault-tolerant and resilient services. Tools like Hystrix can help Java developers isolate failures, prevent cascading failures, and provide fallback options.
Here's an example of using Hystrix for fault tolerance in Java:
@HystrixCommand(fallbackMethod = "fallback")
public String getRemoteData() {
// Call remote service
}
public String fallback() {
// Fallback logic
}
In this example, we use Hystrix to wrap a remote service call, providing a fallback method in case of failure. This enhances the resilience of microservices and reduces the complexity of handling failures.
Monitoring and Logging
Effective monitoring and logging are essential for understanding the behavior and performance of microservices. Java developers can tackle this complexity by using tools like Prometheus for monitoring and ELK stack for logging.
// Example of using Micrometer for monitoring
registry.counter("requests", "endpoint", "/api/endpoint");
// Example of logging using Logback
logger.info("Request received: {}", request);
In this example, we use Micrometer to monitor the number of requests to an endpoint and Logback for logging request information. This provides visibility into the microservices and reduces the complexity of monitoring and troubleshooting.
Wrapping Up
Microservices architecture presents various complexities for Java developers, but with the right tools and practices, these complexities can be effectively tackled. By embracing asynchronous communication, utilizing service discovery and load balancing, building fault-tolerant services, and implementing effective monitoring and logging, Java developers can navigate the complexities of microservices architecture with confidence.
To delve deeper into these concepts, check out this guide to building microservices in Java, which provides comprehensive insights into Java-based microservices development.
Remember, tackling complexity is all about adopting the right mindset and leveraging the appropriate tools to build robust and scalable microservices.
Checkout our other articles