Seamless Integration: Juggling JUnit 4, 5, & TestNG Tests!
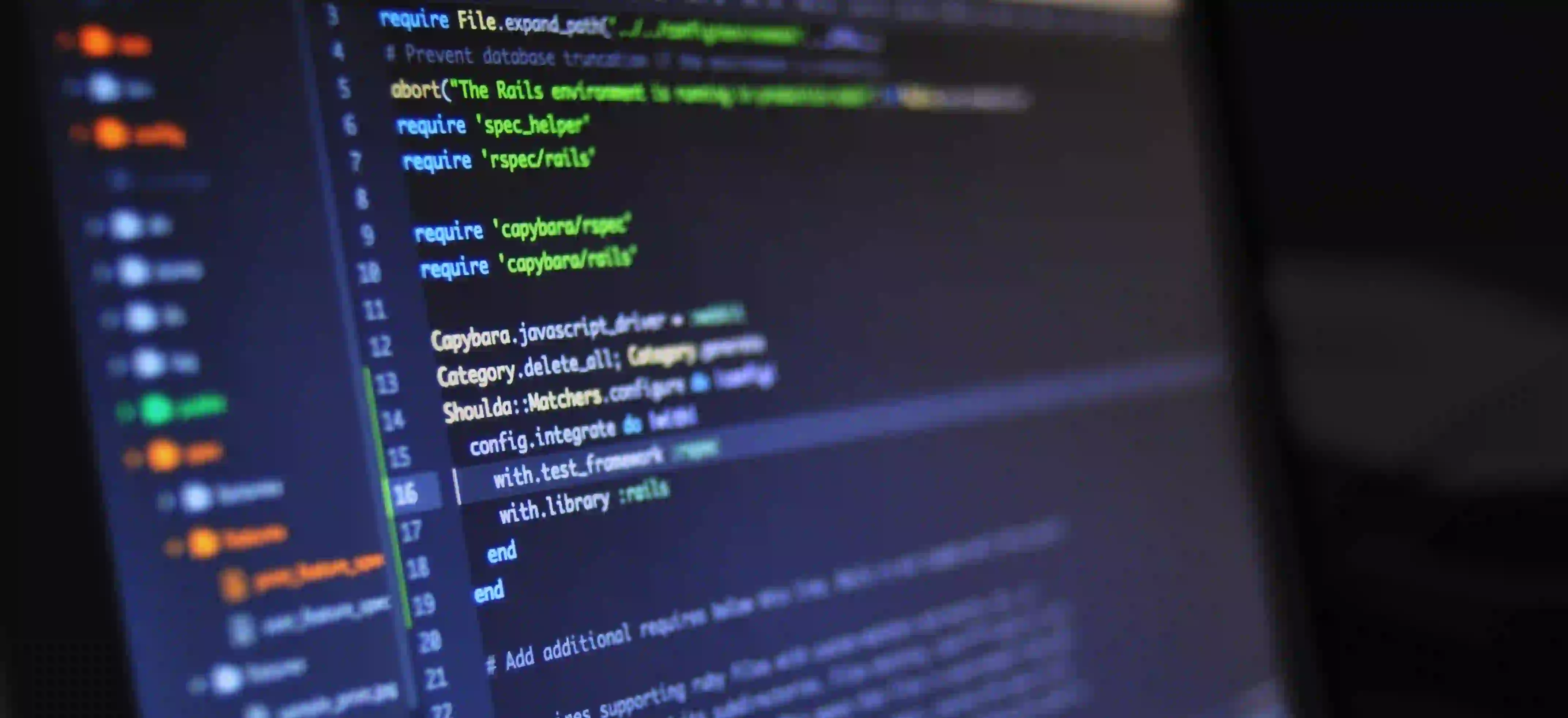
Seamless Integration: Juggling JUnit 4, JUnit 5, & TestNG Tests!
Setting the Stage
Unit testing plays a vital role in software development by ensuring that individual units or components of the software function as expected. The evolution of testing frameworks has seen significant advancements, from JUnit 4 to the more recent JUnit 5, and the existence of TestNG as an alternative option. It's not uncommon for a project to inherit legacy code written in JUnit 4, decide to leverage specific features of JUnit 5, and have team members who prefer TestNG. In such scenarios, the need to seamlessly integrate JUnit 4, JUnit 5, and TestNG becomes evident.
Why Mix Different Testing Frameworks?
The decision to mix different testing frameworks can be influenced by various factors. Legacy code, for instance, may already be written using JUnit 4, making it impractical to migrate all tests to JUnit 5 or TestNG immediately. JUnit 5 offers new features and enhancements over JUnit 4, such as nested tests, @DisplayName
for better readability, and improved extension support. TestNG, on the other hand, provides features not available in JUnit, such as native support for data-driven testing and better handling of parameterized tests.
In addition to feature disparities, team preferences and familiarity with specific frameworks can also influence the decision to mix different testing frameworks. Hence, integrations often arise organically from the diverse needs and capabilities within a development team.
Setting Up the Environment
Maven Dependencies
To integrate JUnit 4, JUnit 5, and TestNG seamlessly in a single project using Maven, you need to include specific dependencies.
JUnit 4 Maven Dependency
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
JUnit 5 Maven Dependencies
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
TestNG Maven Dependency
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
In addition to dependencies, configure the surefire-plugin
in the pom.xml
file to enable the seamless execution of tests from all three frameworks.
Gradle Setup
For those preferring Gradle over Maven, a similar setup can be achieved using the following Gradle configuration in the build.gradle
file:
JUnit 4 Gradle Dependency
testImplementation 'junit:junit:4.12'
JUnit 5 Gradle Dependencies
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.7.0'
testRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine:5.7.0'
TestNG Gradle Dependency
testImplementation 'org.testng:testng:7.4.0'
Writing Tests with JUnit 4, JUnit 5, and TestNG
Now, let's dive into how tests are written with each of these frameworks to understand the differences in syntax and features.
JUnit 4 Example
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class JUnit4Tests {
@Test
public void testAddition() {
assertEquals(5, 2 + 3);
}
}
JUnit 5 Example
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
@DisplayName("JUnit 5 Tests")
public class JUnit5Tests {
@Test
@DisplayName("Addition Test")
public void testAddition() {
assertEquals(5, 2 + 3);
}
}
TestNG Example
import org.testng.annotations.Test;
import static org.testng.Assert.assertEquals;
public class TestNGTests {
@Test
public void testAddition() {
assertEquals(5, 2 + 3);
}
}
Running Your Tests
Running tests from all three frameworks together is possible using Maven or Gradle commands.
Maven Command
Use the following Maven command to run all tests:
mvn clean test
Gradle Command
For Gradle, run the tests using the following command:
./gradlew test
Dealing with Common Issues
When integrating different testing frameworks, common issues may arise, including conflicting versions, test discovery discrepancies, and execution order discrepancies. One approach to address version conflicts is to utilize specific version ranges or to manage dependencies carefully to avoid conflicts. For execution order discrepancies, relying on specific annotations or naming conventions can help enforce a predictable order.
Best Practices
To manage a multi-framework test environment successfully, it's best to limit the use of multiple frameworks when possible. This simplifies the test suite and minimizes potential issues from framework interactions. Additionally, keeping dependencies up to date, maintaining consistent naming and organization of test classes, and documenting the integration approach can help in standardizing the testing process.
To Wrap Things Up
The seamless integration of JUnit 4, JUnit 5, and TestNG in a project can address various needs and preferences within a development team. Understanding the setup, writing tests across frameworks, running the tests, and dealing with potential issues contributes to a robust testing environment. Experimenting with each framework's features and sharing experiences across different testing platforms can lead to comprehensive test coverage and improved overall software quality.
Additional Resources
For further exploration, here are some official documentation and resources:
- JUnit 4: https://junit.org/junit4/
- JUnit 5: https://junit.org/junit5/
- TestNG: https://testng.org/
We encourage readers to share their own experiences and insights regarding integrating JUnit 4, JUnit 5, and TestNG. Feel free to engage in the comments section below.