Overcome Authentication Hurdles in Java with Twitter4J
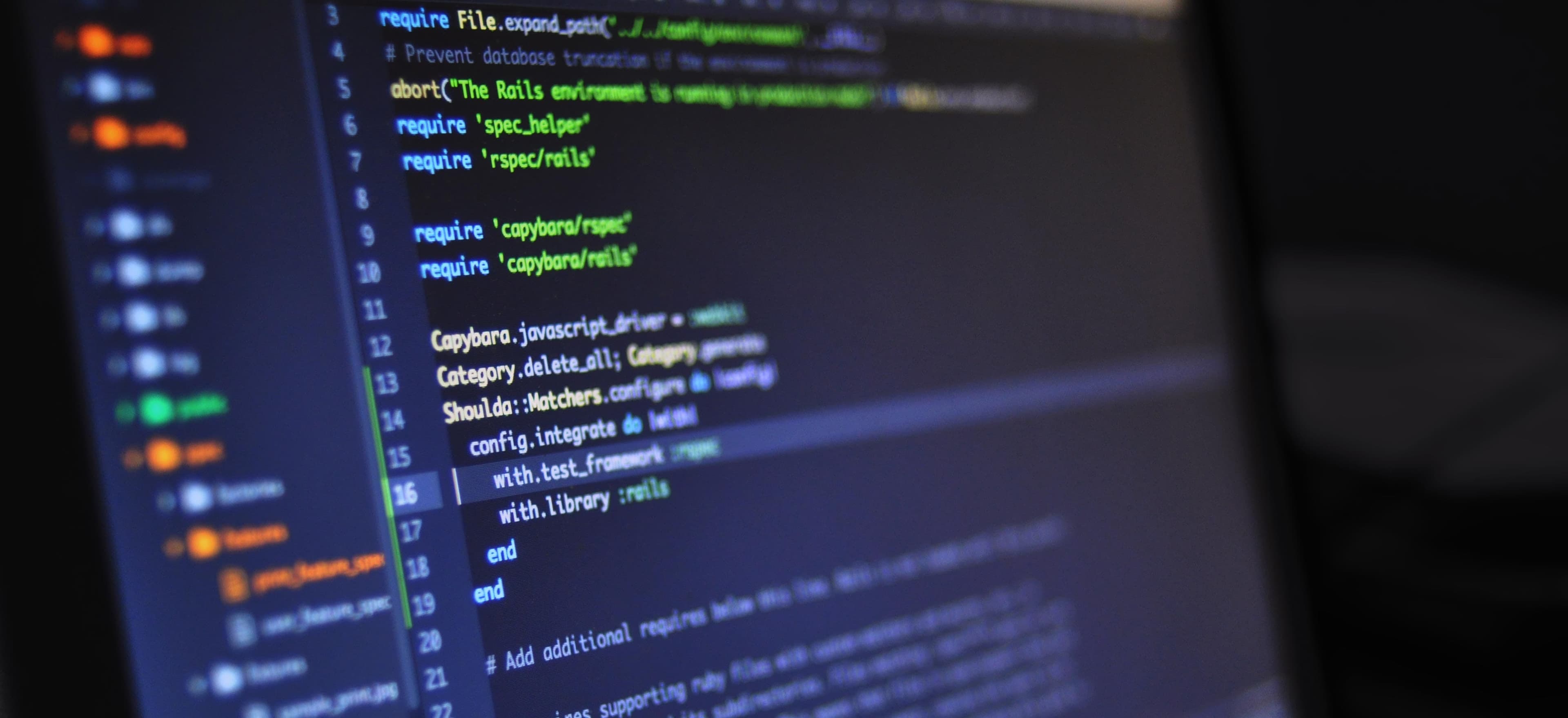
- Published on
Overcome Authentication Hurdles in Java with Twitter4J: A Comprehensive Guide
In the realm of Java development, integrating social media functionality into applications can significantly enhance user engagement and broaden the application's reach. Twitter, being one of the most popular social media platforms, offers vast opportunities for developers to tap into. However, the process of incorporating Twitter’s API for authentication and data access in Java can appear daunting, especially for newcomers. This is where Twitter4J shines as a beacon of hope. In this comprehensive guide, we'll dive deep into overcoming authentication hurdles in Java with Twitter4J, ensuring your journey is as smooth as possible.
What is Twitter4J?
Twitter4J is an unofficial Java library for the Twitter API. It's open-source, fully featured, and provides an easy-to-use interface for integrating Twitter services into your Java applications. What makes Twitter4J particularly appealing is its simplicity in handling OAuth authentication, which is a major stumbling block for many developers.
Setting Up Your Development Environment
Before we jump into the intricacies of Twitter4J and OAuth authentication, ensure you have the following:
- Java Development Kit (JDK) installed on your system.
- An IDE of your choice (Eclipse, IntelliJ IDEA, etc.)
- Maven or Gradle for dependency management.
To add Twitter4J to your project, include the following dependency in your Maven pom.xml
:
<dependency>
<groupId>org.twitter4j</groupId>
<artifactId>twitter4j-core</artifactId>
<version>[4.0.7,]</version>
</dependency>
Or if you're using Gradle, add this to your build.gradle
:
implementation 'org.twitter4j:twitter4j-core:[4.0.7,]'
Understanding OAuth
OAuth stands as a secure authorization protocol, allowing applications to authenticate against Twitter without handling users' passwords directly. Twitter4J simplifies the OAuth process significantly, but understanding the flow is crucial:
- Register your application with Twitter Developer to obtain API keys.
- Use these keys to request a temporary token.
- Direct the user to authenticate this token.
- Exchange the authenticated token for an access token.
Register Your Application
First and foremost, visit the Twitter Developer portal to create your app. Upon registration, you will receive four key pieces of information critical for authentication:
- Consumer API Key
- Consumer API Secret Key
- Access Token
- Access Token Secret
Keep these details secure as they are the cornerstone of interacting with Twitter’s API.
Crafting Your First Authentication
With Twitter4J, initiating OAuth authentication is straightforward. Here’s how you can authenticate and post a tweet:
import twitter4j.Twitter;
import twitter4j.TwitterFactory;
import twitter4j.auth.AccessToken;
public class TweetPoster {
public static void main(String[] args) {
try {
// Initialize Twitter4J
Twitter twitter = TwitterFactory.getSingleton();
// Set your consumer keys and access tokens
twitter.setOAuthConsumer("<Your Consumer API Key>", "<Your Consumer API Secret Key>");
AccessToken accessToken = new AccessToken("<Your Access Token>", "<Your Access Token Secret>");
// Authenticate
twitter.setOAuthAccessToken(accessToken);
// Post a Tweet
String tweetMessage = "Hello, Twitter World!";
twitter.updateStatus(tweetMessage);
System.out.println("Successfully posted: " + tweetMessage);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Code Works
- Simplicity: Twitter4J abstracts the complexity of directly dealing with the Twitter API's OAuth process.
- Security: It securely handles authentication using the OAuth tokens and secrets you provide, ensuring your app communicates safely with Twitter's services.
- Efficiency: You can post a tweet with just a few lines of code, making it highly efficient for developers.
Navigating Twitter's Rate Limiting
While Twitter4J makes authentication and interaction straightforward, it's important to be mindful of Twitter’s rate limiting. These limits are in place to ensure fair usage and protect against spam. Fortunately, Twitter4J offers ways to monitor and manage your API usage to stay within these bounds.
import twitter4j.RateLimitStatus;
import twitter4j.Twitter;
import twitter4j.TwitterFactory;
public class RateLimitChecker {
public static void main(String[] args) {
try {
Twitter twitter = TwitterFactory.getSingleton();
Map<String, RateLimitStatus> rateLimitStatus = twitter.getRateLimitStatus();
for (String endpoint : rateLimitStatus.keySet()) {
RateLimitStatus status = rateLimitStatus.get(endpoint);
System.out.println("Endpoint: " + endpoint);
System.out.println("Limit: " + status.getLimit());
System.out.println("Remaining: " + status.getRemaining());
System.out.println("Reset Time in Seconds: " + status.getSecondsUntilReset());
System.out.println("------");
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Insights Into the Rate Limit Checker
- Transparency: It offers a clear view of your current rate limit status across different endpoints, empowering you to make informed decisions about API usage.
- Preventive Measure: By understanding your limits, you can design your application to prevent hitting these limits unexpectedly, thus ensuring a smoother user experience.
The Bottom Line
Integrating Twitter functionality into your Java applications doesn't have to be an uphill battle. With Twitter4J, you can smoothly navigate through OAuth authentication hurdles, enriching your applications with social media interactions. Whether it's posting tweets, reading timelines, or handling rate limits, Twitter4J offers a comprehensive yet straightforward approach.
Remember: Always respect user privacy and Twitter's guidelines to create positive and meaningful interactions.
For more advanced uses and detailed documentation, refer to the official Twitter4J documentation and keep exploring what you can achieve with this powerful library. Happy coding!
While the focus today has been primarily on getting started with posting a tweet and understanding rate limits, Twitter4J's capabilities extend much further. From streaming live tweets to analyzing tweet sentiments, the potential use cases are vast and varied. As you grow more comfortable with the basics, don't hesitate to experiment and expand your application's social media prowess.