Mastering Apache Flink: Solving Stream Processing Challenges
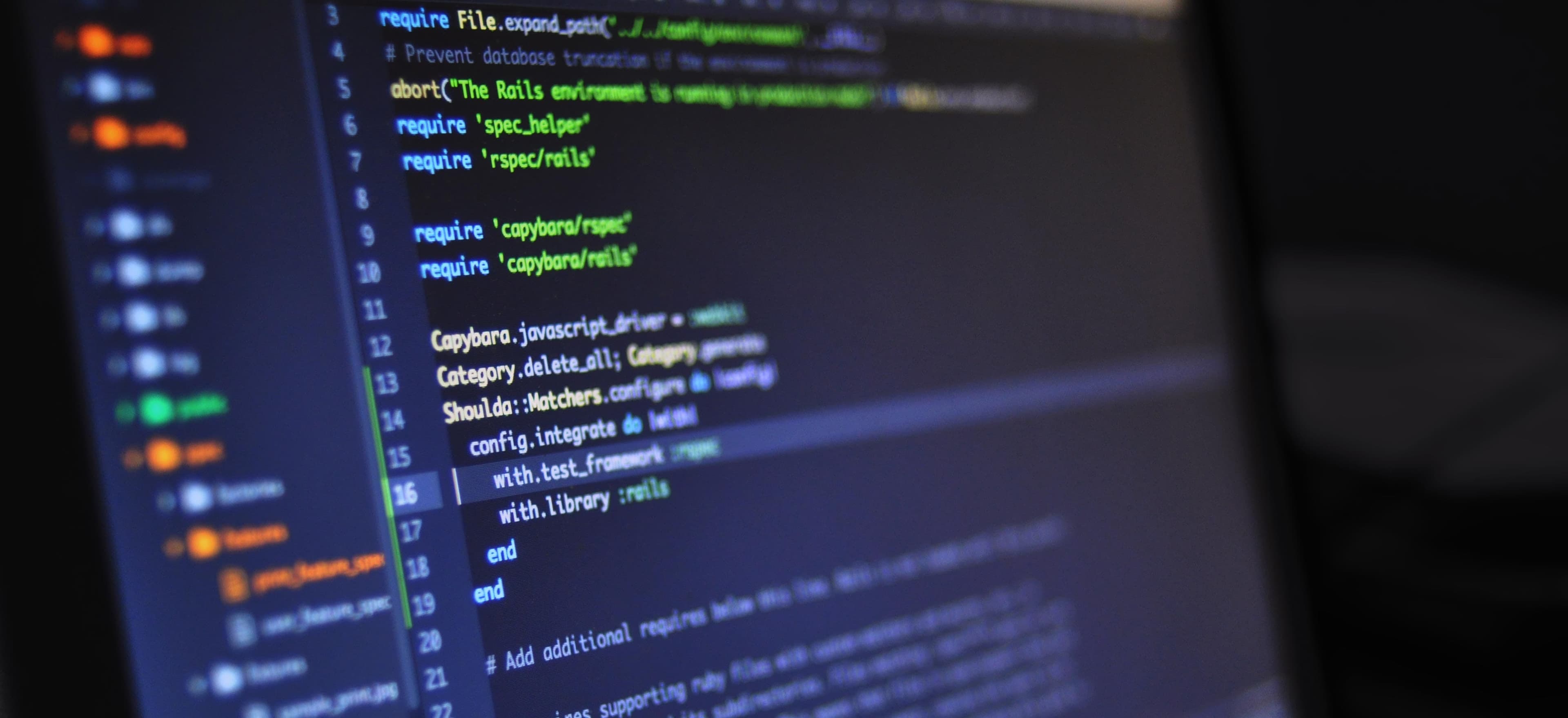
- Published on
Mastering Apache Flink: Solving Stream Processing Challenges
Apache Flink is a powerful and versatile open-source stream processing framework that has gained significant popularity in the Java community. Its ability to handle large amounts of streaming data with low latency makes it a go-to choice for real-time analytics, event-driven applications, and machine learning pipelines. In this blog post, we’ll delve into the intricacies of Apache Flink, explore some common stream processing challenges, and demonstrate how Flink can effectively address them.
Understanding Apache Flink
Apache Flink is designed to process continuous streams of data at scale. It provides a unified runtime for both batch and stream processing, ensuring seamless transition between the two modes. Flink offers robust support for event time processing, stateful computations, and exactly-once processing semantics, making it well-suited for handling real-time data with utmost accuracy and reliability.
With its distributed processing capabilities, Flink can run on various cluster environments, enabling horizontal scalability and fault tolerance. Flink's advanced APIs empower developers to write complex data processing pipelines with ease, leveraging high-level constructs for tasks such as windowing, state management, and event-driven processing.
Stream Processing Challenges
Before we delve into the solutions Apache Flink offers, let's first examine some common challenges encountered in stream processing.
Low Latency
Real-time data processing demands low latency, meaning that the system must be able to handle and process incoming data with minimal delay. Achieving low latency in stream processing requires a framework that can efficiently parallelize the work, manage state, and handle event time processing effectively.
Fault Tolerance
Stream processing systems must be resilient to failures and ensure that data processing continues seamlessly in the event of node failures or data inconsistencies. Fault tolerance involves maintaining consistent state across the processing pipeline and enabling automatic recovery from failures.
State Management
Many stream processing applications involve stateful computations, where the processing logic relies on maintaining and updating state information. Managing this state in a distributed and fault-tolerant manner poses a significant challenge in stream processing systems.
Apache Flink's Solutions
Now, let's explore how Apache Flink addresses these challenges with its powerful features and capabilities.
Low Latency
Apache Flink achieves low latency by employing techniques such as pipelined data processing, adaptive buffering, and efficient network communication. Flink's ability to process and route data in a pipelined fashion minimizes the processing overhead, while adaptive buffering enables it to balance throughput and latency dynamically. Additionally, Flink's native support for event time processing ensures accurate and efficient handling of timestamped data streams.
Example of Pipelined Data Processing in Flink:
// Example of a simple Flink data processing pipeline
DataStream<Event> events = env
.addSource(new EventSource())
.filter(event -> event.getType().equals("user_interaction"))
.map(event -> new EnrichedEvent(event, fetchUserDetails(event.getUserId())))
.keyBy(event -> event.getUserId())
.timeWindow(Time.seconds(30))
.reduce((event1, event2) -> mergeEvents(event1, event2))
.addSink(new EventSink());
In this example, the pipeline processes incoming events in a pipelined fashion, applying different operations such as filtering, mapping, windowing, and reducing to efficiently handle the stream data.
Fault Tolerance
Flink ensures fault tolerance by maintaining consistent state checkpoints and providing mechanisms for automatic recovery in case of failures. Flink's checkpointing mechanism allows it to persist the state of the processing pipeline at regular intervals, enabling the system to restore from the last consistent state in case of failures. Moreover, Flink’s distributed coordination and recovery protocols ensure seamless recovery from failures without compromising data integrity.
Example of Checkpointing in Flink:
// Enabling checkpointing with a 10-second interval
env.enableCheckpointing(10000);
env.getCheckpointConfig().setCheckpointTimeout(60000);
In this example, Flink is configured to enable checkpointing at a 10-second interval, with a checkpoint timeout of 60 seconds, ensuring that the system consistently persists its state to facilitate fault tolerance.
State Management
Flink simplifies state management by providing built-in support for managing and persisting state in a fault-tolerant manner. Flink's stateful processing APIs, such as Keyed State and Operator State, enable developers to manage and query state within the processing logic. The framework handles the distribution, synchronization, and fault tolerance of the state transparently, ensuring that stateful computations are executed reliably.
Example of Keyed State in Flink:
// Example of using Flink's Keyed State for stateful computation
public class UserCountFunction extends KeyedProcessFunction<String, Event, Integer> {
private ValueState<Integer> countState;
@Override
public void open(Configuration parameters) {
ValueStateDescriptor<Integer> descriptor =
new ValueStateDescriptor<>("userCount", Integer.class);
countState = getRuntimeContext().getState(descriptor);
}
@Override
public void processElement(Event event, Context context, Collector<Integer> collector) {
// Update the count state based on the incoming event
int currentCount = countState.value() != null ? countState.value() : 0;
countState.update(currentCount + 1);
collector.collect(currentCount + 1);
}
}
In this example, the UserCountFunction
uses Flink's Keyed State to maintain a count of user interactions, updating and querying the state within the processing logic to perform stateful computations accurately.
Closing the Chapter
Apache Flink offers robust solutions for addressing the challenges of stream processing, making it an ideal choice for real-time data processing and analytics. Its low-latency processing, fault-tolerant design, and simplified state management capabilities set it apart as a leading framework for building scalable and reliable stream processing applications in Java.
By harnessing the power of Apache Flink, developers can tackle the complexities of stream processing with confidence, enabling them to build responsive and resilient data-driven applications.
In conclusion, mastering Apache Flink equips Java developers with the tools and knowledge to excel in the realm of stream processing, unlocking the potential to solve real-time data challenges with finesse.
To further explore Apache Flink, you can refer to the official Apache Flink documentation and dive deeper into its rich ecosystem of features and capabilities.
In summary, Apache Flink stands as a formidable contender in the landscape of stream processing frameworks, empowering developers with the means to conquer the complexities of real-time data processing with finesse and efficiency.