Why You Should Migrate from Java's Deprecated Observer
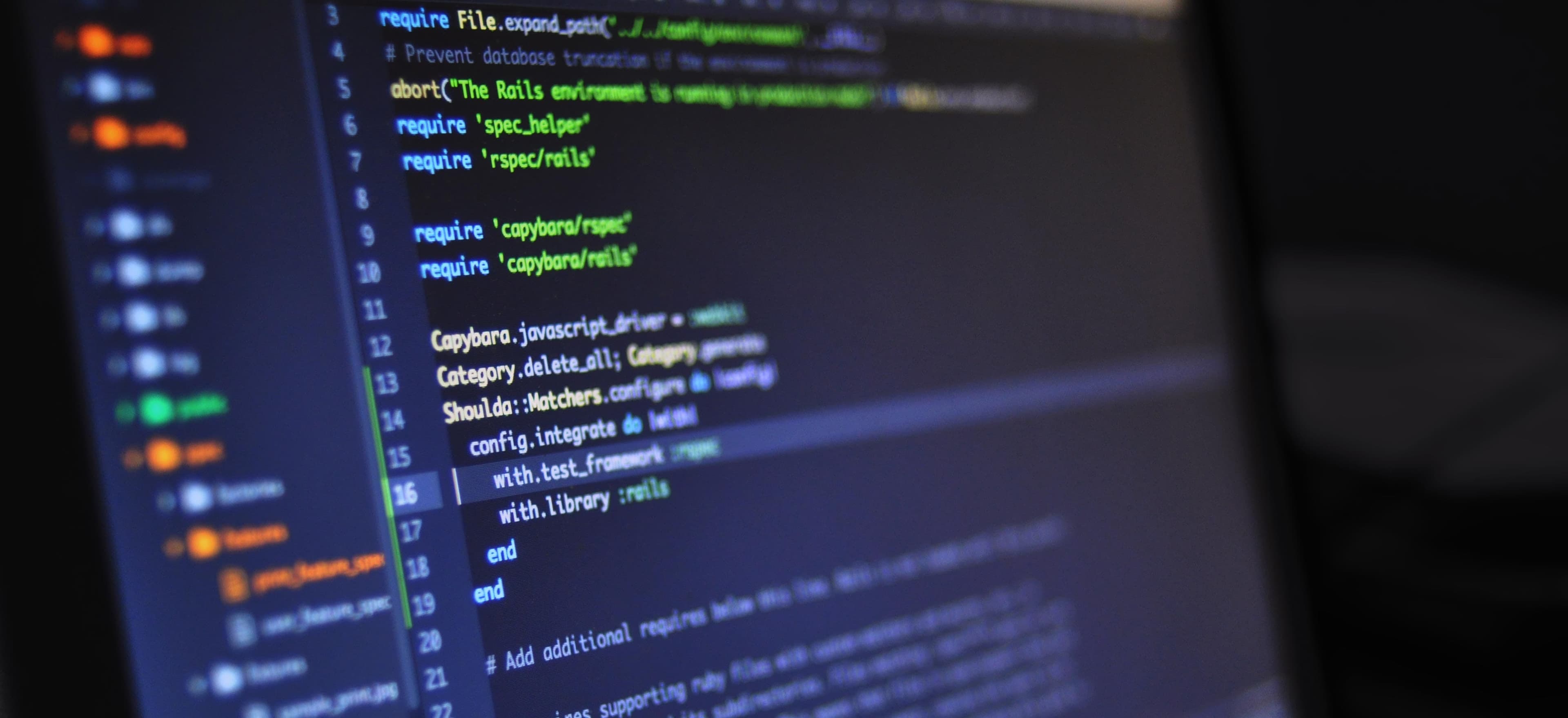
- Published on
Why You Should Migrate from Java's Deprecated Observer
Java has a rich history of programming constructs, some of which, like the Observer design pattern, have served developers well for years. However, as the language and its ecosystem have evolved, certain elements have become outdated and even deprecated. One such feature is the Observer class and interface, and in this blog post, we will explore why migrating away from this deprecated pattern is essential for maintaining modern, efficient, and clean code.
Understanding the Observer Pattern
The Observer pattern is a behavioral design pattern that defines a one-to-many dependency between objects. When one object (the subject) changes its state, all its dependents (observers) are notified and updated automatically. While this pattern has its advantages, its implementation in Java has led to significant drawbacks.
Key Features of the Observer Pattern
- Decoupling: The Observer pattern helps achieve a loose coupling between subjects and observers, making the system more flexible.
- Real-time Notification: Changes in the subject can immediately alert all observers, ensuring that they always have the latest state.
Why the Observer Is Deprecated
Despite its advantages, there are several reasons why the Observer pattern implemented via Java's java.util.Observer
and java.util.Observable
classes has been deprecated in Java 9.
-
Lack of Flexibility: The Observer mechanism restricts observers to extend the
Observable
class, which complicates inheritance chains. This limitation forces developers to use interfaces or alternate patterns to achieve the same result, negating the goal of simplicity. -
Memory Leaks: The Observer pattern can lead to memory leaks if a subject keeps a reference to its observers. If an observer doesn’t unregister itself, it can prevent garbage collection of the observer, leading to performance issues.
-
Thread Safety: The implementation of the Observer pattern as designed in Java does not account for thread safety, which can result in unpredictable behavior in multithreaded applications.
-
Complex Dependency Management: Managing dependencies becomes cumbersome, especially when the number of observers increases, leading to a situation where you may not always be aware of which observers need to be updated.
Alternatives to the Deprecated Observer Pattern
While the Observer pattern has its merits, there are modern alternatives that provide the same functionality without the disadvantages. Here, we will explore a few of the most popular replacements.
1. JavaFX Property Bindings
If you're developing applications using JavaFX, the Property bindings provided by the JavaFX library can be a robust alternative to the Observer pattern. JavaFX properties are observable, allowing changes to be automatically reflected in the user interface.
Example of JavaFX Property Bindings
import javafx.beans.property.SimpleStringProperty;
import javafx.beans.property.StringProperty;
public class User {
private StringProperty name = new SimpleStringProperty();
public String getName() {
return name.get();
}
public void setName(String name) {
this.name.set(name);
}
public StringProperty nameProperty() {
return name;
}
}
In the above code:
- We define a
StringProperty
calledname
. - Changes to the name will trigger listeners that might be bound to elements in the UI.
- This allows for a clean, efficient way to handle data changes in your applications.
By utilizing JavaFX properties, you're also inherently ensuring thread safety as modifications on properties are safely handled by the JavaFX application thread.
2. Java Streams and Collectors
If you're working with a collection of data that needs to be observed for changes, the Java Stream API provides a powerful mechanism for handling changes without needing to implement observers manually.
Example with Java Streams
import java.util.List;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
List<Integer> scores = Arrays.asList(80, 70, 90);
double average = scores.stream()
.mapToInt(Integer::intValue)
.average()
.orElse(0);
System.out.println("Average score: " + average);
}
}
Here, the average score is calculated using streams, eliminating the need for managing observers manually. As data changes, you can recalculate derived states as needed without relying on a costly observer framework.
3. Use of EventBus Libraries
Libraries like Guava's EventBus or Spring's ApplicationEventPublisher are great replacements for traditional observer patterns. They decouple the event source from event handlers, making the system more maintainable.
Example of Guava’s EventBus
import com.google.common.eventbus.EventBus;
import com.google.common.eventbus.Subscribe;
public class Main {
private static EventBus eventBus = new EventBus();
public static void main(String[] args) {
eventBus.register(new Object() {
@Subscribe
public void handleEvent(String event) {
System.out.println("Received event: " + event);
}
});
eventBus.post("Hello, Observer!");
}
}
In this example, an event handler subscribes to the EventBus and reacts to posted events. This system prevents the memory and circular dependency issues prominent in traditional observer patterns.
Migrating from Observer: A Step-by-Step Guide
Step 1: Identify Deprecated Usage
Scan through your codebase to identify Observable
and Observer
classes, noting where they are heavily relied upon.
Step 2: Choose an Alternative
Decide which alternative suits your needs best—JavaFX Properties, Streams, or an EventBus.
Step 3: Refactor the Code
Refactor your code to replace Observable
and Observer
classes with the selected alternative. Ensure to minimize disruptions to functionality during this process.
Step 4: Test Extensively
Once you implement the changes, ensure robust testing to validate that your new implementation accommodates all necessary use cases and edge cases.
Step 5: Educate Your Team
Document your reasoning and the new implementation for your team. Knowledge sharing will help foster a culture of modern development practices.
The Last Word: Embracing Modern Practices
Shifting away from Java's deprecated Observer design pattern paves the way for cleaner, more efficient, and maintainable code. Modern alternatives, such as JavaFX property bindings, streams, and EventBus libraries, provide all the functionality one would need without the drawbacks.
So, if you're still using Observable
and Observer
, it's time to embrace modern practices. Not only will you benefit in terms of cleaner code, but you will also enjoy improved performance and decreased risk of memory leaks in your applications. By standing at the forefront of technology trends, you’re ensuring that your Java applications can grow and adapt with the ever-evolving software development landscape.
For more in-depth knowledge on designing robust software, check out Java Design Patterns and Effective Java as excellent resources.