Fixing Maven Dependency Hell: A Birds-Eye Solution
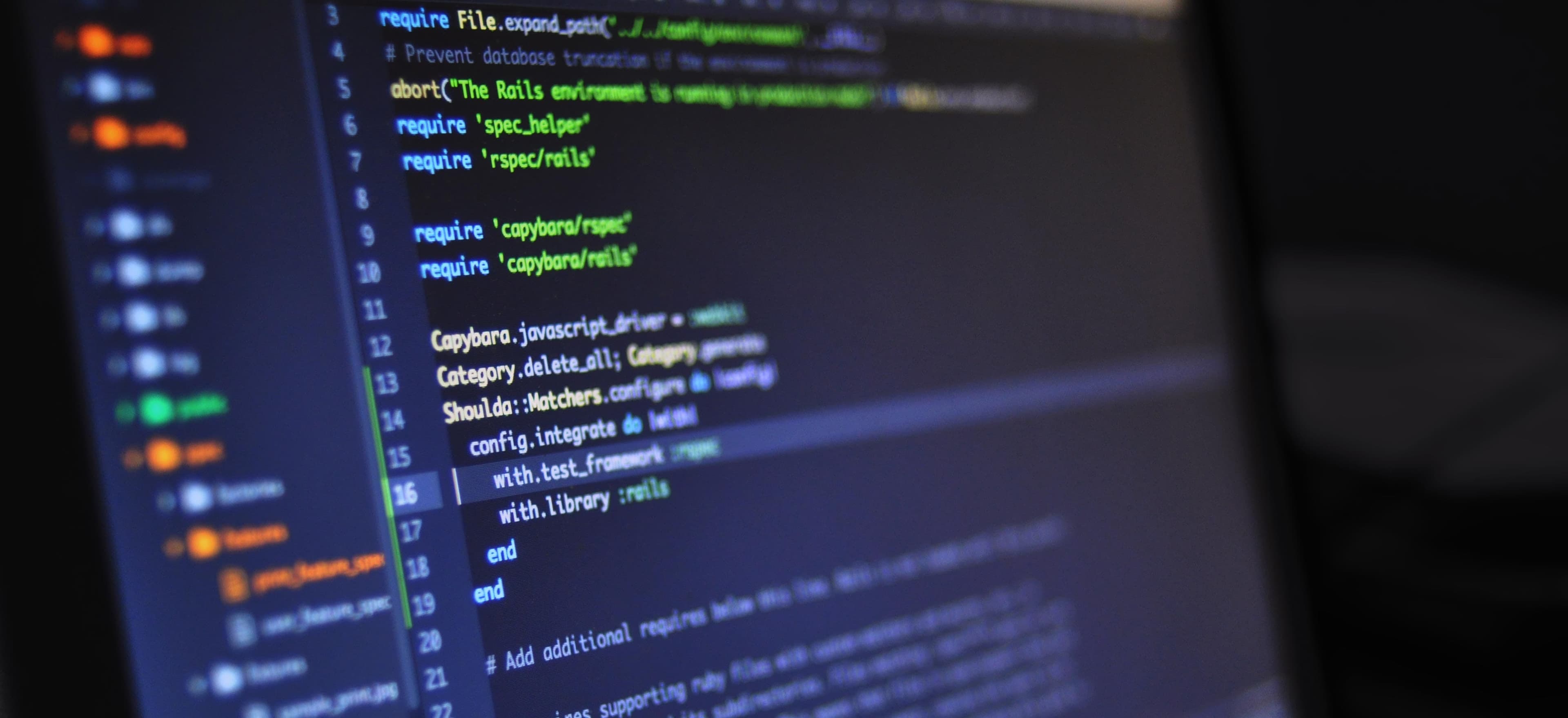
- Published on
Fixing Maven Dependency Hell: A Birds-Eye Solution
Maven is an essential build automation tool for Java projects, widely used for managing dependencies and building projects. However, as projects grow in complexity and scale, Maven dependency management can become cumbersome, leading to what is commonly referred to as "Dependency Hell". In this post, we will delve into the causes of Maven dependency issues and explore effective solutions to mitigate these problems.
Understanding Maven Dependency Hell
What is Maven Dependency Hell?
Maven dependency hell refers to the chaotic situation when a project has complex and conflicting dependencies, leading to build failures, runtime errors, and overall project instability. This can occur due to incompatible versions of transitive dependencies, conflicting dependency trees, or outdated libraries.
Common Causes of Dependency Hell:
- Transitive Dependency Issues: When a project has transitive dependencies, conflicts might arise due to different versions being pulled in by different direct dependencies.
- Conflicting Dependency Versions: When different dependencies require conflicting versions of the same library, it can lead to conflicts and runtime errors.
- Outdated Dependencies: Using outdated versions of dependencies can lead to compatibility issues and potential vulnerabilities.
Effective Strategies to Resolve Maven Dependency Hell
1. Dependency Management
Maven provides a powerful mechanism for dependency management. By explicitly declaring the versions of your dependencies in the pom.xml
file, you can avoid conflicts arising from transitive dependencies pulling in different versions. Using the <dependencyManagement>
section allows you to centralize version definitions, ensuring consistency across the project.
2. Exclusion of Problematic Transitive Dependencies
In scenarios where a transitive dependency is causing issues, Maven allows for exclusion of specific transitive dependencies. This can be done within the <dependencies>
section of the pom.xml
for the problematic dependency.
3. Dependency Tree Analysis
By utilizing the mvn dependency:tree
command, you can visualize and analyze the complete dependency tree of your project. This provides insights into the specific versions being brought in by transitive dependencies, aiding in identifying conflicts and redundancies.
4. Use of Bill of Materials (BOM)
Maven BOM is an effective approach for managing dependencies in a multi-module project. It centralizes the version information for a set of artifacts, ensuring that all modules use the same version of dependencies. This helps in eliminating version mismatches and conflicts.
Code Examples
Explicitly declaring the version of a dependency:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.8</version>
</dependency>
<!-- Other dependencies -->
</dependencies>
In this example, by specifying the version of spring-core
, we ensure that any transitive dependencies pulling in spring-core
will use the declared version, mitigating version conflicts.
Excluding problematic transitive dependency:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<!-- Other dependencies -->
</dependencies>
In this case, the exclusion of spring-boot-starter-tomcat
ensures that its transitive dependencies are not pulled into the project, resolving potential conflicts.
Closing the Chapter
Maven Dependency Hell can be a significant pain point for developers, leading to project instability and build complexities. By adopting effective dependency management strategies, such as explicit version declaration, exclusion of problematic transitive dependencies, dependency tree analysis, and utilization of BOM, it is possible to mitigate these issues and create a more robust and maintainable project structure.
In summary, proactive and vigilant management of dependencies is crucial for ensuring project stability and smooth builds in Maven. By implementing the discussed strategies, developers can navigate through the murky waters of dependency hell and emerge with well-structured, resilient projects.
For further insights into Maven dependency management, consult the official Maven documentation and deepen your understanding of best practices for effective dependency resolution.
Remember, a well-managed set of dependencies can be the bedrock of a successful Java project!
Incorporating these strategies into your Maven projects can drastically reduce the chances of encountering dependency conflicts and inconsistencies, making your development process more manageable and efficient. If you have any questions or thoughts, feel free to share them in the comments section below!
Checkout our other articles