Unlocking Java: The Quirks of Static Synchronized Methods
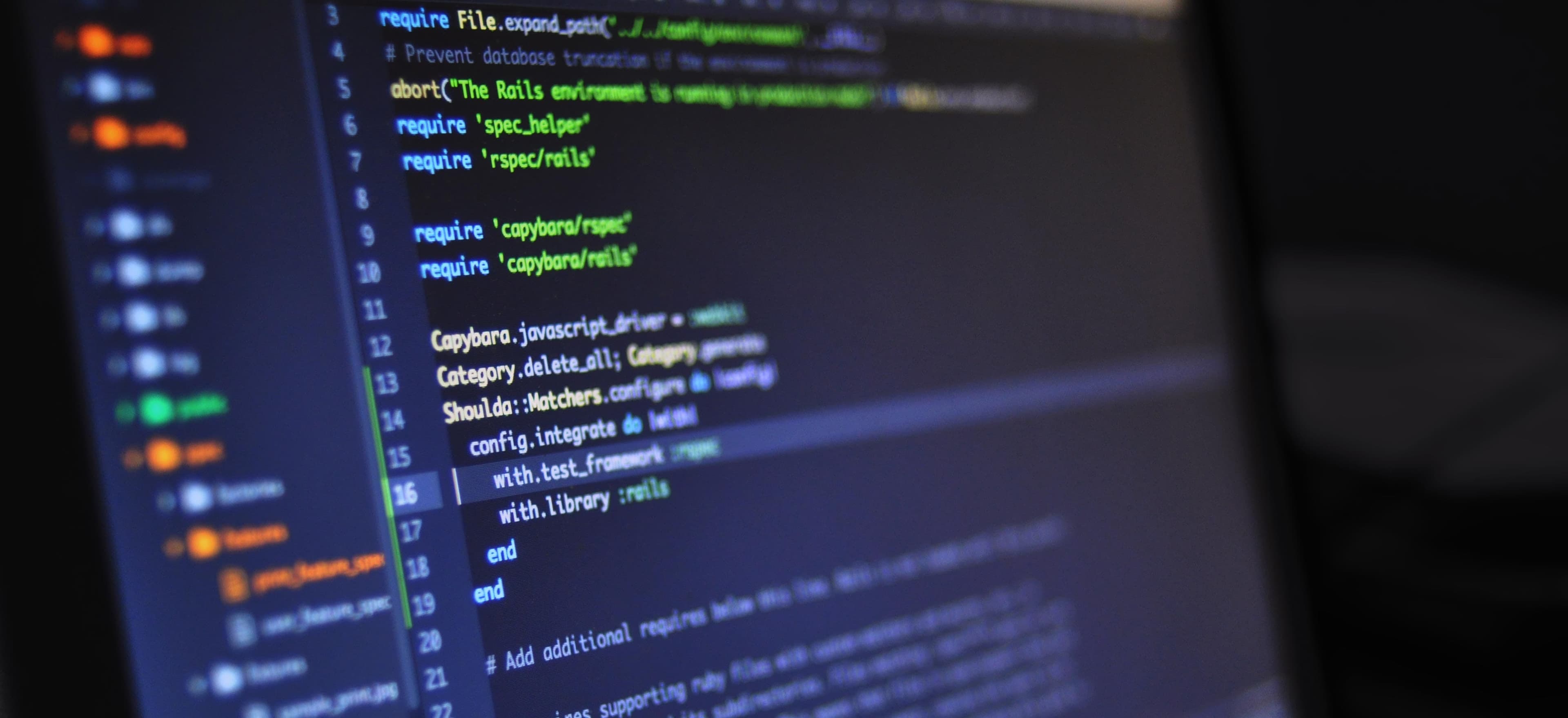
- Published on
Unlocking Java: The Quirks of Static Synchronized Methods
Java stands as one of the most popular programming languages, renowned for its robustness, security features, and object-oriented concepts. Among its various facets, synchronization in Java is a critical concept that ensures thread safety, especially in multi-threaded environments. In this blog post, we will deep dive into a specific aspect of synchronization: Static Synchronized Methods. By understanding their quirks and functionality, we can leverage them to write more efficient and safer code.
Understanding Synchronization in Java
Before we delve into the nuances of static synchronized methods, let's quickly recap what synchronization in Java entails. Synchronization in Java is a mechanism that restricts multiple threads from accessing a certain segment of code at the same time. This is particularly imperative when threads operate on shared resources, preventing scenarios like data inconsistency and thread interference.
Synchronization can be achieved in Java using:
- Synchronized methods
- Synchronized blocks
- Static synchronized methods (our focus)
Static Synchronized Methods: A Deep Dive
A method declared with the static synchronized
modifier means that the method is synchronized on the class itself, not on an instance of the class. This is because static methods belong to the class level, not to any specific instance.
Why Static Synchronization?
When multiple threads attempt to access static synchronized methods of a class, they are synchronized on the class's Class object, ensuring that only one thread can access the class's static synchronized method at any given time. This is quintessential to ensure thread safety for static data managed by static methods.
Code Example: Static Synchronized Method
Consider a scenario where we have a counter class that tracks the number of instances created.
public class InstanceCounter {
private static int count = 0;
public static synchronized void increment() {
count++;
System.out.println("Instances: " + count);
}
}
In this example, the increment
method is a static synchronized method. This ensures that even when multiple threads concurrently access this method, it correctly increments and prints the instance count, providing thread-safe access to the static count
variable.
The Quirks of Using Static Synchronized Methods
-
Class-level Locking: Unlike instance methods, where each object has its lock, a static synchronized method locks the Class object of the class. This means, while a thread holds a lock on a static synchronized method, no other thread can access any static synchronized method of that class.
-
Performance Consideration: While ensuring safety, static synchronization might lead to a performance hit if not used judiciously. Since it locks at the class level, it could potentially block threads that need to access other static synchronized methods of the class, even if those methods do not work on shared resources.
-
Best Practices: Use static synchronized methods when you need to synchronize across all instances of a class or when you are manipulating static fields. However, always be mindful of the broader impact on your application’s performance and scalability. For an in-depth discussion on best practices, visit the official Java documentation.
Alternatives to Static Synchronization
In scenarios where the performance overhead of static synchronization is too great, consider alternative approaches:
-
Concurrent Data Structures: Java provides a wealth of concurrent data structures (like
ConcurrentHashMap
,AtomicInteger
, etc.) designed for multithreaded environments, minimizing the need for explicit synchronization (Java Concurrent Collections). -
Locks: The
java.util.concurrent.locks
package offers more granular control over locking than synchronized methods and blocks, potentially leading to better scalability and performance.
Code Example: Using ReentrantLock
as an Alternative
import java.util.concurrent.locks.ReentrantLock;
public class InstanceCounter {
private static int count = 0;
private static final ReentrantLock lock = new ReentrantLock();
public static void increment() {
lock.lock();
try {
count++;
System.out.println("Instances: " + count);
} finally {
lock.unlock();
}
}
}
In this variant, we use ReentrantLock
to control access to the increment
method. This approach grants the same thread-safety as static synchronization but with potentially better control and performance, especially in high-concurrency scenarios.
Bringing It All Together
Static synchronized methods serve as a powerful tool in Java for ensuring thread safety when accessing static data or methods at the class level. However, it's crucial to understand their quirks and limitations. By judiciously applying static synchronization and considering alternatives when appropriate, developers can maintain efficient, safe, and scalable Java applications.
Remember, every tool and feature in Java serves a purpose, and understanding when and how to use them is key to unlocking the full potential of this versatile programming language.
For more in-depth insights into Java’s synchronization mechanisms and best practices, consider exploring resources like the Oracle Java Tutorials and reputable Java community forums. Happy coding!