Overcoming Performance Hurdles in Scalable Systems
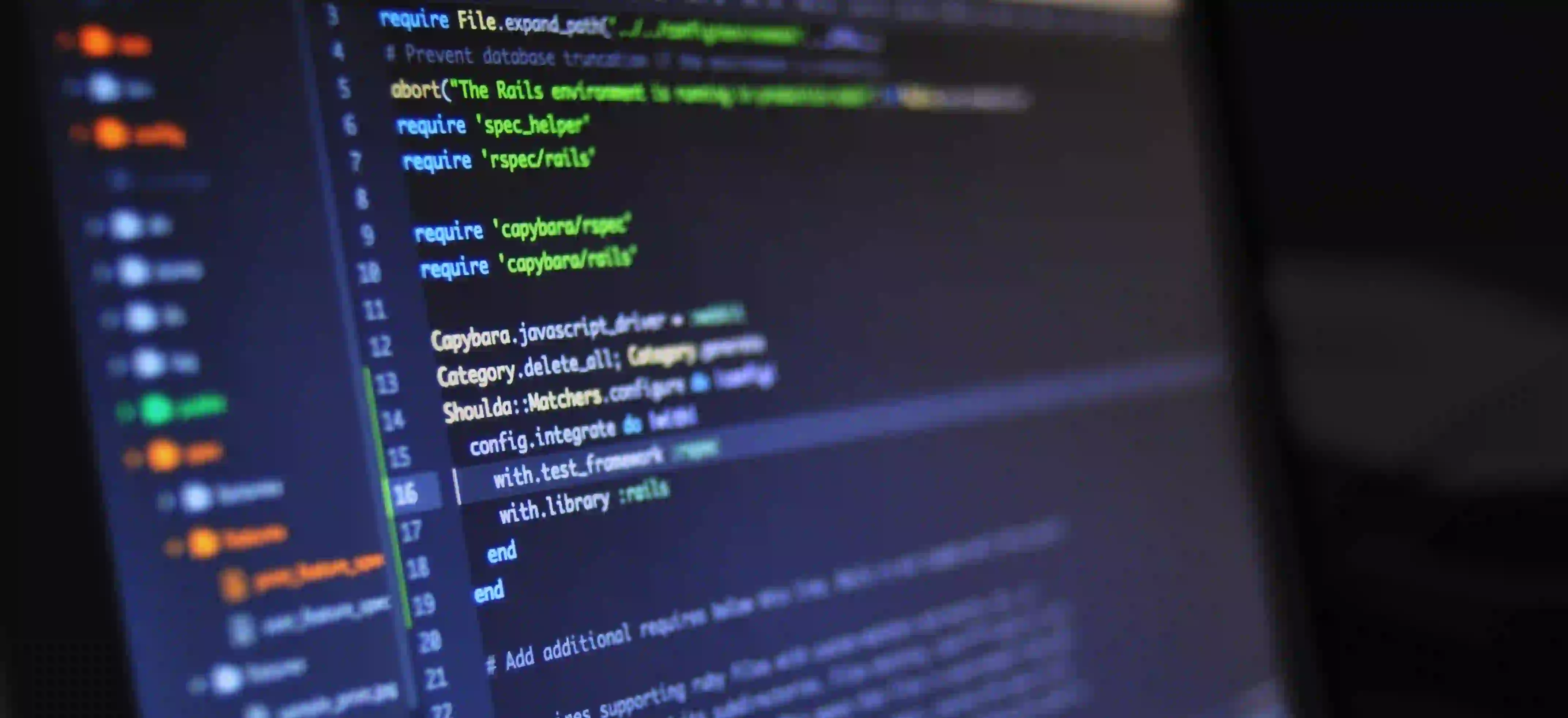
Overcoming Performance Hurdles in Scalable Systems
In the world of software development, performance is crucial. Regardless of the size and complexity of the system, performance issues can significantly impact user experience, customer satisfaction, and the overall success of a project. When it comes to building scalable systems, the challenge to maintain high performance becomes even more critical. In this article, we'll explore some common performance hurdles in scalable systems and discuss strategies to overcome them, specifically in the context of Java development.
Understanding Performance Hurdles in Scalable Systems
Scalable systems are designed to handle increasing amounts of work by adding resources to the system. However, as the system scales, performance bottlenecks can emerge, hindering the overall efficiency. Some of the common performance hurdles in scalable systems include:
1. Database Queries and Indexing
- The Issue: As the system scales, the database can become a bottleneck due to inefficient queries and inadequate indexing.
- The Solution: Optimizing queries, adding appropriate indexes, and denormalizing data can significantly improve database performance.
2. Concurrency and Thread Management
- The Issue: Concurrent access to shared resources can lead to contention and slow response times.
- The Solution: Proper thread management, synchronization, and the use of concurrent data structures can address concurrency issues.
3. I/O Operations
- The Issue: Scalable systems often face performance challenges related to I/O operations, such as reading from and writing to files or network sockets.
- The Solution: Leveraging non-blocking I/O, asynchronous I/O, and efficient use of buffers can enhance I/O performance.
4. Memory Management
- The Issue: Inefficient memory allocation and garbage collection can impact the system's performance as it scales.
- The Solution: Utilizing memory-efficient data structures, minimizing object creation, and tuning garbage collection parameters can improve memory management.
Java Best Practices for Overcoming Performance Hurdles
Java, known for its robustness and performance, provides several tools and techniques to address performance hurdles in scalable systems. Let's delve into some best practices and strategies to overcome these challenges:
1. Database Performance Optimization with JPA and Hibernate
Java Persistence API (JPA) and Hibernate are widely used in Java applications for object-relational mapping (ORM). When dealing with database performance issues, it's crucial to optimize the use of JPA and Hibernate to minimize the number of database queries and fetch only the required data.
@Entity
@Table(name = "products")
public class Product {
@Id
@Column(name = "id")
private Long id;
@Column(name = "name")
private String name;
// Other fields and methods
}
In the above example, the Product
entity is annotated with JPA annotations, providing metadata for object-relational mapping. By carefully crafting JPA queries and utilizing Hibernate's caching mechanisms, unnecessary database hits can be reduced, improving database performance in scalable systems.
2. Effective Thread Management with Java's Executor Framework
Java provides the Executor
framework, which simplifies concurrent task execution. By utilizing thread pools and executor services, Java applications can efficiently manage the execution of asynchronous tasks, mitigating the impact of contention and thread management issues.
ExecutorService executor = Executors.newFixedThreadPool(10);
executor.submit(() -> {
// Asynchronous task logic
});
In the code snippet above, we create a fixed thread pool of size 10 using Executors.newFixedThreadPool()
. Tasks are submitted to the executor service, enabling efficient thread management and concurrency control.
3. Enhancing I/O Performance with NIO and Asynchronous I/O
Java's NIO (New I/O) provides support for non-blocking I/O operations, which can significantly improve the performance of scalable systems that handle a large number of I/O operations. Additionally, Java's asynchronous I/O capabilities, introduced in Java 7, allow for efficient handling of I/O-bound tasks without blocking threads.
// Non-blocking I/O with NIO
ByteBuffer buffer = ByteBuffer.allocate(48);
channel.read(buffer);
// Asynchronous I/O with Java 7's AsynchronousFileChannel
Path file = Paths.get("example.txt");
AsynchronousFileChannel asynchronousFileChannel = AsynchronousFileChannel.open(file, StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024);
asynchronousFileChannel.read(buffer, 0, buffer, new CompletionHandler<Integer, ByteBuffer>() {
@Override
public void completed(Integer result, ByteBuffer attachment) {
// Asynchronous completion logic
}
@Override
public void failed(Throwable exc, ByteBuffer attachment) {
// Error handling logic
}
});
The snippet showcases both non-blocking I/O with NIO and asynchronous I/O with Java 7's AsynchronousFileChannel
, demonstrating the potential for improved I/O performance in scalable systems.
4. Efficient Memory Management Using Java's Garbage Collector Tuning
Java's Garbage Collector (GC) plays a critical role in memory management. By understanding the behavior of the GC and tuning its parameters based on the application's characteristics and workload, developers can optimize memory usage and mitigate performance issues related to inefficient memory allocation and garbage collection.
Configuring GC options such as heap size, generation sizes, and garbage collection algorithms can have a profound impact on the memory footprint and responsiveness of a Java application, especially in scalable systems with varying workloads.
The Last Word
Performance hurdles in scalable systems can be daunting, but with the right tools, techniques, and best practices, Java developers can address these challenges effectively. By optimizing database access, implementing efficient concurrency and thread management, enhancing I/O operations, and mastering memory management, Java applications can achieve high performance and scalability, ensuring a seamless user experience and robust system capabilities.
Striving for optimal performance in scalable systems should be an ongoing commitment, with regular performance monitoring, profiling, and continuous improvement efforts. Equipped with the knowledge and strategies discussed in this article, Java developers can navigate the complexities of scalable system performance and deliver exceptional software solutions that meet the demands of today's dynamic and ever-growing digital landscape.