Mastering API Access: Common Pitfalls and Solutions
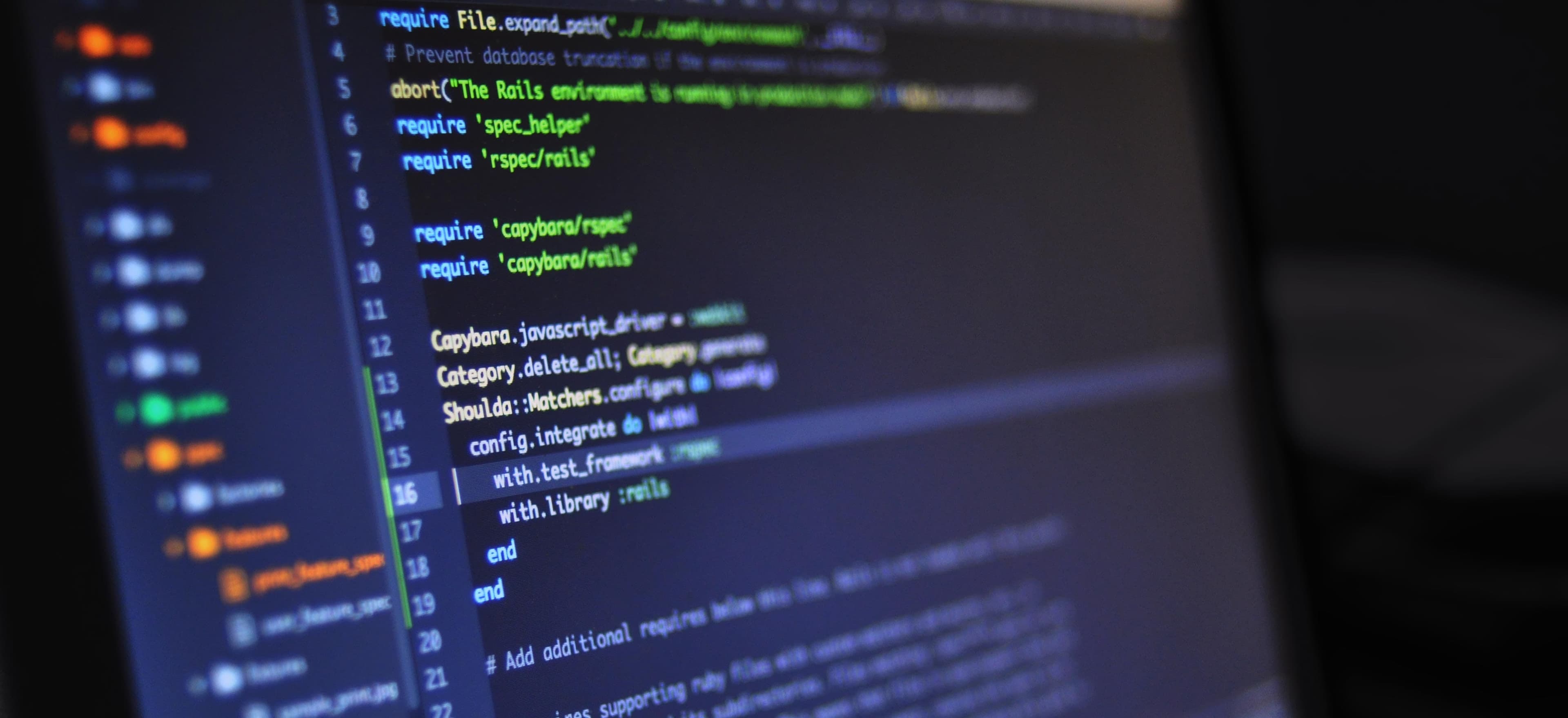
- Published on
Mastering API Access: Common Pitfalls and Solutions
Application Programming Interfaces (APIs) are the backbone of modern software development. They facilitate communication between different applications, enabling integration and functionality that can dramatically improve user experience. However, accessing APIs is fraught with challenges. In this blog post, we will explore some common pitfalls when working with APIs and provide actionable solutions to overcome these issues.
Table of Contents
- Understanding the Basics of API Access
- Common Pitfalls
- 2.1 Lack of Proper Authentication
- 2.2 Misunderstanding API Limits and Rate Limiting
- 2.3 Failing to Handle API Errors Gracefully
- Best Practices for Effective API Use
- Conclusion
Understanding the Basics of API Access
Before diving into common pitfalls, let's clarify what an API is. An API allows different software applications to communicate through a defined set of protocols—think of it as a menu in a restaurant. The menu lists dishes you can order, and the kitchen knows how to prepare those dishes.
In Java, accessing an API typically involves sending HTTP requests and handling the responses. Here’s a simple example of how to make an API call in Java using the HttpURLConnection
class.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class ApiExample {
public static void main(String[] args) {
try {
String url = "https://api.example.com/data";
HttpURLConnection conn = (HttpURLConnection) new URL(url).openConnection();
conn.setRequestMethod("GET");
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) { // 200 OK
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Code Works
- URL Creation: The URL string points to the desired API endpoint.
- HttpURLConnection: This class is initialized with the URL and allows you to set the request method.
- Response Handling: The response is read if the status code is
200 OK
.
Further Reading
For a deeper understanding of how Java handles HTTP requests, you can read Java's official documentation on HttpURLConnection.
Common Pitfalls
1. Lack of Proper Authentication
Many APIs require authentication for access. Failing to provide valid credentials—or using the wrong type of authentication—can lead to errors.
Solution
Always check the API documentation for required authentication methods. If an API uses OAuth 2.0, for instance, you need to obtain a token.
Here’s how you might pass a bearer token in your Java code:
conn.setRequestProperty("Authorization", "Bearer " + token);
2. Misunderstanding API Limits and Rate Limiting
Most APIs have rate limits that restrict the number of requests you can make within a time frame. Ignoring these limits can lead to throttling or outright banning for excessive requests.
Solution
Make sure to read the documentation to understand these limits. Implement exponential backoff in your request strategy.
Here's an outline of how to incorporate this:
public void fetchWithRetry(String url) throws InterruptedException {
int retries = 3;
while (retries > 0) {
try {
// Your API call logic here
break; // Exit if successful
} catch (IOException e) {
Thread.sleep(2000); // Wait before retrying
retries--;
}
}
}
3. Failing to Handle API Errors Gracefully
When things go awry, how your application deals with errors can make or break user experience. APIs can return various HTTP status codes, each indicating a different issue.
Solution
Implement error handling to ensure that your application can respond appropriately. A typical way to handle this is through a switch statement based on the responseCode
.
int responseCode = conn.getResponseCode();
switch (responseCode) {
case HttpURLConnection.HTTP_OK:
// handle success
break;
case HttpURLConnection.HTTP_UNAUTHORIZED:
System.out.println("Unauthorized access!");
break;
case HttpURLConnection.HTTP_NOT_FOUND:
System.out.println("Resource not found!");
break;
default:
System.out.println("Unexpected error: " + responseCode);
}
Best Practices for Effective API Use
-
Read the Documentation: This cannot be overstated. Know what to expect before you start coding.
-
Use Libraries When Possible: Libraries such as Retrofit or OkHttp can simplify API interactions. For instance, Retrofit allows you to define your API calls as interfaces and handles response parsing automatically.
-
Monitor Usage: Keep an eye on your rate limits and call counts. Some APIs offer dashboards to track this info.
-
Cache Responses: Caching can significantly reduce the number of requests. If your data does not change frequently, cache it locally to decrease load on the API.
-
Secure Your App: Never expose your API keys in your client-side code. Use environment variables or server-side logic to keep them secure.
The Last Word
Mastering API access can significantly enhance your application's functionality. By understanding and addressing common pitfalls, you can create robust applications that offer an excellent user experience. Always remember to consult the API documentation to gain insights specific to the API you are working with.
With knowledge, practice, and the right tools, you'll navigate the world of APIs with confidence. Happy coding!
Checkout our other articles