Overcoming File Manipulation Hurdles in Java 7
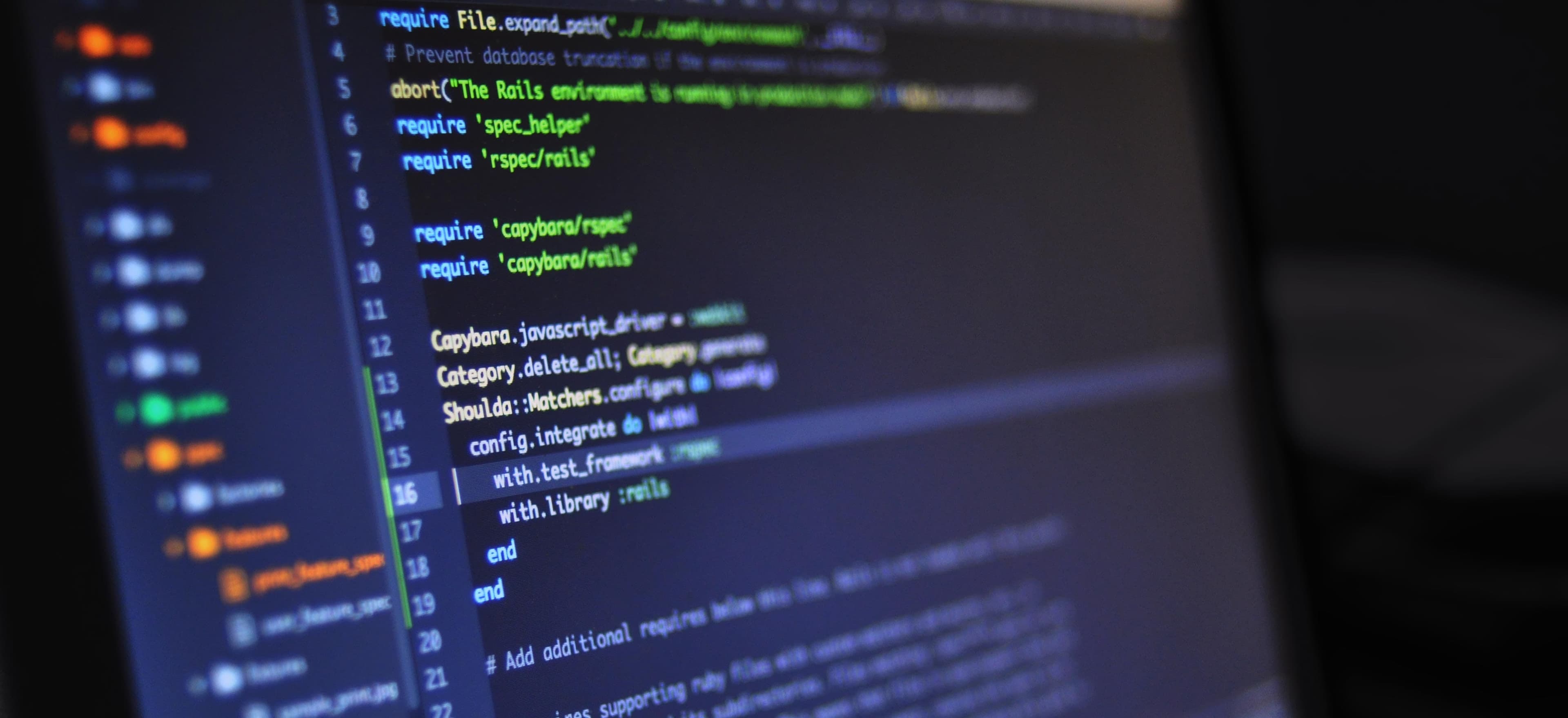
- Published on
Overcoming File Manipulation Hurdles in Java 7
File manipulation is a common task in Java development, and with the release of Java 7, several new features have made this process more efficient and flexible. In this article, we will explore some of the hurdles developers often face when working with files in Java 7 and showcase how to overcome them using the new capabilities introduced in this version.
The Need for Efficient File Manipulation
When working with file manipulation, developers often encounter challenges such as repetitive code for common tasks, difficulty in handling file operations, and lack of support for modern file system features. Java 7 addresses these issues with the introduction of the NIO.2 API, also known as the "New I/O."
Simplifying File Operations with NIO.2
Before Java 7, manipulating files in Java involved using classes like File
, which had limitations and did not provide a fluent API for working with files. NIO.2, on the other hand, introduced the Path
interface, which represents the location of a file or directory on the file system.
Let's take a look at an example of creating a directory using the NIO.2 API:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileManipulationExample {
public static void main(String[] args) {
Path directoryPath = Paths.get("/path/to/newDirectory");
try {
Path newDirectory = Files.createDirectory(directoryPath);
System.out.println("Directory created: " + newDirectory);
} catch (IOException e) {
System.err.println("Unable to create directory: " + e.getMessage());
}
}
}
In this example, we create a new directory using the Files.createDirectory
method, which provides a more streamlined approach compared to the traditional File
class.
Handling File I/O Operations
Another common challenge when working with files is performing I/O operations efficiently. In Java 7, the Files
class provides a wealth of methods for reading, writing, and manipulating files.
Let's examine how to read the contents of a file using the Files.readAllLines
method:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class FileManipulationExample {
public static void main(String[] args) {
try {
List<String> lines = Files.readAllLines(Paths.get("/path/to/file.txt"));
for (String line : lines) {
System.out.println(line);
}
} catch (IOException e) {
System.err.println("Unable to read file: " + e.getMessage());
}
}
}
In the above example, we use Files.readAllLines
to read all lines from a file into a List
of strings. This provides a concise and efficient way to read file contents compared to traditional file I/O operations.
Working with Modern File System Features
Java 7 introduces support for modern file system features such as symbolic links, file permissions, and file attributes. The Files
class includes methods for working with these features, providing developers with more control over file manipulation in Java.
Let's look at an example of setting file permissions using the Files.setPosixFilePermissions
method:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.nio.file.attribute.PosixFilePermission;
import java.nio.file.attribute.PosixFilePermissions;
import java.io.IOException;
import java.util.Set;
public class FileManipulationExample {
public static void main(String[] args) {
Path filePath = Paths.get("/path/to/file.txt");
Set<PosixFilePermission> permissions = PosixFilePermissions.fromString("rwxr-x---");
try {
Files.setPosixFilePermissions(filePath, permissions);
System.out.println("File permissions set successfully");
} catch (IOException e) {
System.err.println("Unable to set file permissions: " + e.getMessage());
}
}
}
In this example, we use the Files.setPosixFilePermissions
method to set POSIX file permissions on a file. This demonstrates the ability to work with modern file system features in Java 7.
Final Considerations
Java 7 has significantly improved file manipulation capabilities with the introduction of the NIO.2 API. Developers can now perform file operations more efficiently, handle I/O operations with ease, and work with modern file system features using the enhanced capabilities provided by Java 7.
By utilizing the Path
interface and the methods available in the Files
class, developers can overcome common file manipulation hurdles and write cleaner, more concise code for working with files in Java.
In conclusion, Java 7 has paved the way for more streamlined and efficient file manipulation in Java, empowering developers to tackle file-related tasks with enhanced capabilities and greater flexibility.
For further exploration and advanced techniques, I highly recommend diving into the official Java 7 documentation and exploring the various functionalities offered by the NIO.2 API.
Are you ready to elevate your file manipulation skills in Java 7? Let's start coding!
Checkout our other articles