Unlock Flexibility: Master Feature Toggles in Spring Boot 2
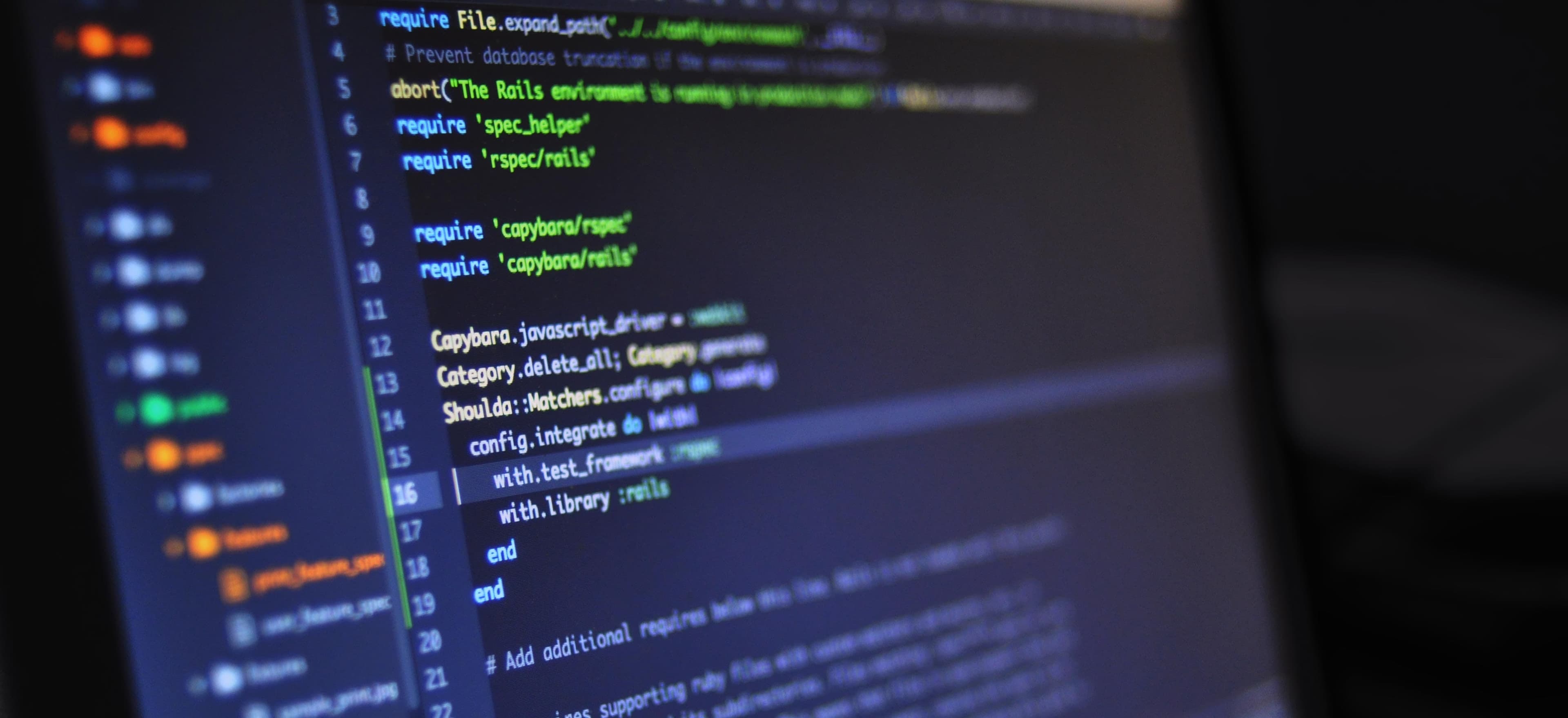
- Published on
Unlock Flexibility: Master Feature Toggles in Spring Boot 2
In the dynamic world of software development, the ability to quickly enable or disable certain features without deploying new code is a game-changer. This is where feature toggles, also known as feature flags, come into play.
What are Feature Toggles?
Feature toggles allow you to control the visibility of specific features in your application, often at runtime, without altering the code. By employing feature toggles, you can enable or disable features remotely, which is invaluable for gradual feature rollouts, A/B testing, and handling unfinished features. Spring Boot 2 provides robust support for incorporating feature toggles into your applications.
The Power of Feature Toggles in Spring Boot 2
Spring Boot 2 empowers developers to harness the power of feature toggles using various strategies. One popular approach is leveraging the Spring environment and configuration properties to control feature flags.
Let's explore how you can use feature toggles in Spring Boot 2 to unlock unparalleled flexibility in your applications.
Setting Up Feature Toggles
To begin, add the spring-boot-starter-actuator
dependency to your pom.xml
file. This is necessary to access the /actuator
endpoints provided by Spring Boot, including the /actuator/health
endpoint, which we will utilize for our feature toggles.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Implementing Feature Toggles
Now, let's implement a feature toggle in a Spring Boot 2 application. Suppose we have a feature that needs to be toggled based on a configuration property.
First, define a property in your application.properties
file:
feature.my-feature-enabled=false
Next, create a component that will utilize this feature toggle:
@Component
public class MyFeatureService {
@Value("${feature.my-feature-enabled}")
private boolean myFeatureEnabled;
public void performFeatureAction() {
if (myFeatureEnabled) {
// Feature-specific logic goes here
} else {
// Alternative behavior
}
}
}
In this example, the MyFeatureService
class reads the feature.my-feature-enabled
property. If the property is true
, the feature-specific logic is executed; otherwise, an alternative behavior is triggered.
By using the @Value
annotation from Spring to inject configuration properties, we enable the dynamic control of feature toggles without modifying the code. This approach fosters agility and flexibility, allowing for real-time adjustments to feature availability.
Leveraging Feature Toggles for Controlled Rollouts
An exciting aspect of feature toggles is their role in controlled rollouts. By gradually activating features for specific users or groups, you can mitigate the risks associated with large-scale deployments and obtain valuable feedback from early adopters.
Spring Boot 2 facilitates controlled rollouts by integrating feature toggles with various strategies, such as user-based toggles or percentage-based toggles. This level of granularity empowers you to make informed decisions and refine features based on actual usage and feedback.
Embracing A/B Testing with Feature Toggles
Another compelling use case for feature toggles is A/B testing, which involves comparing two versions of a feature to determine which performs better. By using feature toggles, Spring Boot 2 enables you to seamlessly switch between different implementations or variations of a feature.
You can create distinct paths within your application and use feature toggles to direct users to one version or the other. This capability provides invaluable insights into user preferences and allows you to iterate on feature enhancements iteratively.
Ensuring Stability with Kill Switches
In certain scenarios, it may be necessary to quickly disable a feature to address issues or prevent disruptions. This is where kill switches, a form of feature toggle, come into play. By incorporating kill switches in your Spring Boot 2 application, you can promptly deactivate problematic features without redeploying or modifying the codebase.
The Bottom Line
Incorporating feature toggles in Spring Boot 2 unlocks a new realm of flexibility, enabling you to adapt to changing requirements, mitigate risks, and gather actionable insights. Leveraging feature toggles within your applications offers a strategic advantage by fostering agility, promoting controlled rollouts, facilitating A/B testing, and ensuring stability with kill switches.
As you continue to explore the capabilities of Spring Boot 2 and feature toggles, consider the numerous ways in which this powerful combination can enhance your development process and elevate the overall user experience.
Start harnessing the potential of feature toggles in Spring Boot 2 today to shape the future of your applications with unprecedented versatility and control.
To delve deeper into feature toggles and Spring Boot 2, explore the official Spring Framework documentation and Spring Boot reference guide. These invaluable resources offer comprehensive insights and best practices for leveraging feature toggles effectively in your Spring Boot 2 applications.
Checkout our other articles