Managing Containers 101: Avoiding Common Pitfalls
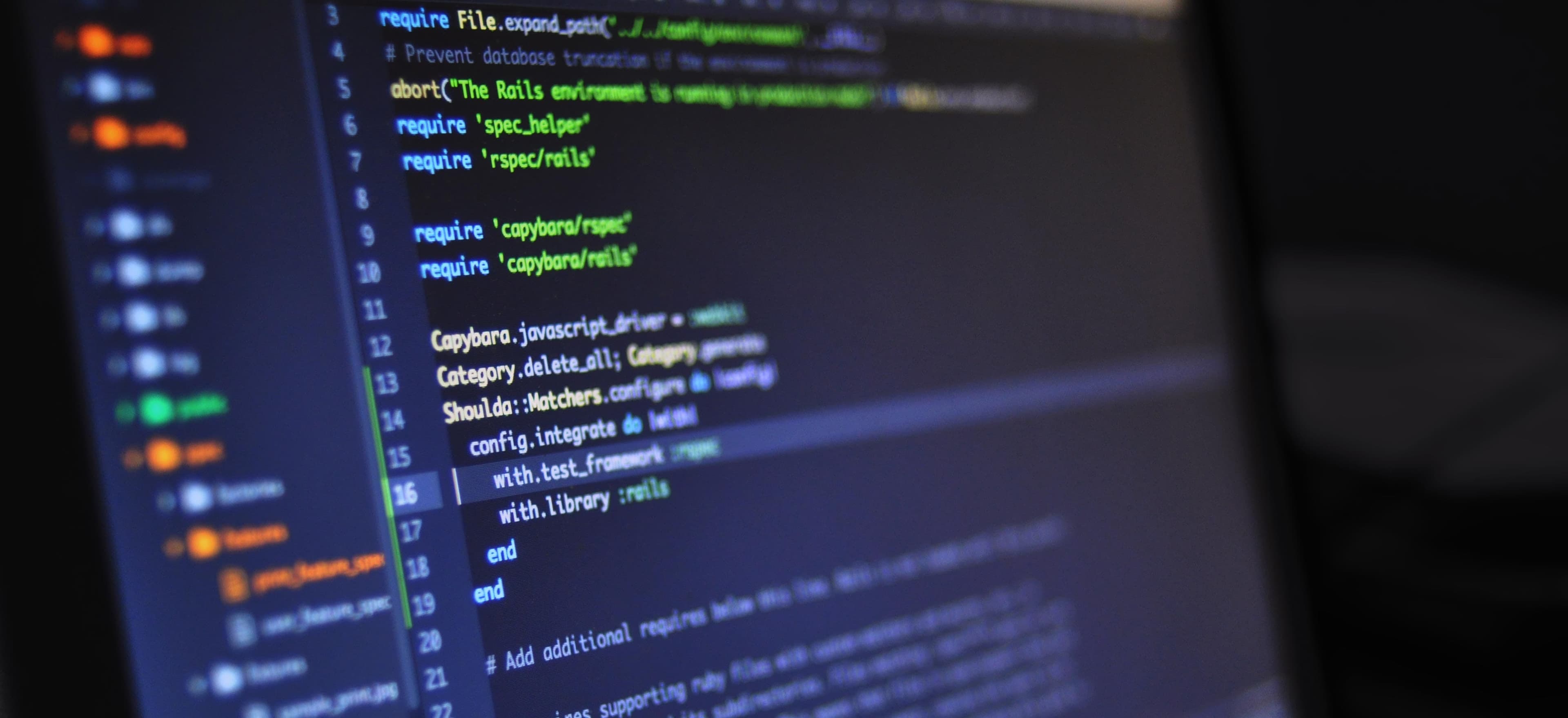
- Published on
Managing Containers 101: Avoiding Common Pitfalls
Containers have become a staple in modern software development and deployment. They offer a lightweight, consistent environment that ensures consistent behavior across different development and production environments. Java, being a popular programming language for enterprise-level applications, is often used in conjunction with containers. However, managing containers effectively is crucial for the success of any project. In this article, we'll explore common pitfalls when managing Java containers and how to avoid them.
Pitfall 1: Ignoring Resource Constraints
One common mistake when managing Java containers is ignoring resource constraints. Java applications are notorious for their memory consumption, and when running inside containers, improper resource allocation can lead to performance issues and unpredictable behavior. To address this, it's essential to set appropriate resource limits and requests for your Java containers.
public class ResourceDemo {
public static void main(String[] args) {
int mb = 1024 * 1024;
Runtime rt = Runtime.getRuntime();
System.out.println("Max Memory: " + rt.maxMemory() / mb + "MB");
System.out.println("Total Memory: " + rt.totalMemory() / mb + "MB");
System.out.println("Free Memory: " + rt.freeMemory() / mb + "MB");
}
}
The above Java code snippet demonstrates how to inspect the memory usage of a Java application. By understanding the memory footprint of your Java application, you can make informed decisions about resource allocation in your container environment.
Pitfall 2: Overlooking Logging and Monitoring
Effective logging and monitoring are crucial for managing Java containers in a production environment. Without proper logging, diagnosing issues becomes a daunting task. Incorporating a robust logging framework such as Log4j or SLF4J and integrating with container orchestration tools like Kubernetes can provide valuable insights into the behavior of your Java applications.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class LoggingDemo {
private static final Logger LOGGER = LoggerFactory.getLogger(LoggingDemo.class);
public static void main(String[] args) {
LOGGER.info("Logging demo in Java container");
}
}
The provided Java snippet showcases a simple logging setup using SLF4J. By diligently implementing logging and monitoring within your Java containers, you gain visibility into application performance and potential issues.
Pitfall 3: Disregarding Security Best Practices
Security should always be a top priority when managing Java containers. Ignoring security best practices, such as using unsecured base images, neglecting proper network policies, and failing to implement container security scanning, can leave your Java applications vulnerable to attacks. Adopting secure coding practices and regularly updating dependencies are critical for safeguarding your containerized Java applications.
Pitfall 4: Neglecting Version Control for Container Configurations
As containerized Java applications become more complex, neglecting version control for container configurations can lead to inconsistencies and deployment challenges. Leveraging version control systems like Git for storing container configurations, Dockerfiles, and deployment descriptors ensures that changes are tracked, traceable, and reversible, promoting a more secure and stable container management workflow.
In Conclusion, Here is What Matters
Effectively managing Java containers requires a proactive approach to address common pitfalls. By acknowledging resource constraints, prioritizing logging and monitoring, adhering to security best practices, and embracing version control for container configurations, you can steer clear of common pitfalls and set the stage for successful containerized Java applications.
In conclusion, navigating the complexities of container management can be challenging, but with careful consideration of these common pitfalls, you can proactively mitigate risks and pave the way for a robust and efficient containerized Java environment. Stay vigilant, stay informed, and stay ahead in managing Java containers.
Checkout our other articles