Boost Performance: Mastering Spring WebFlux for Reactive Apps
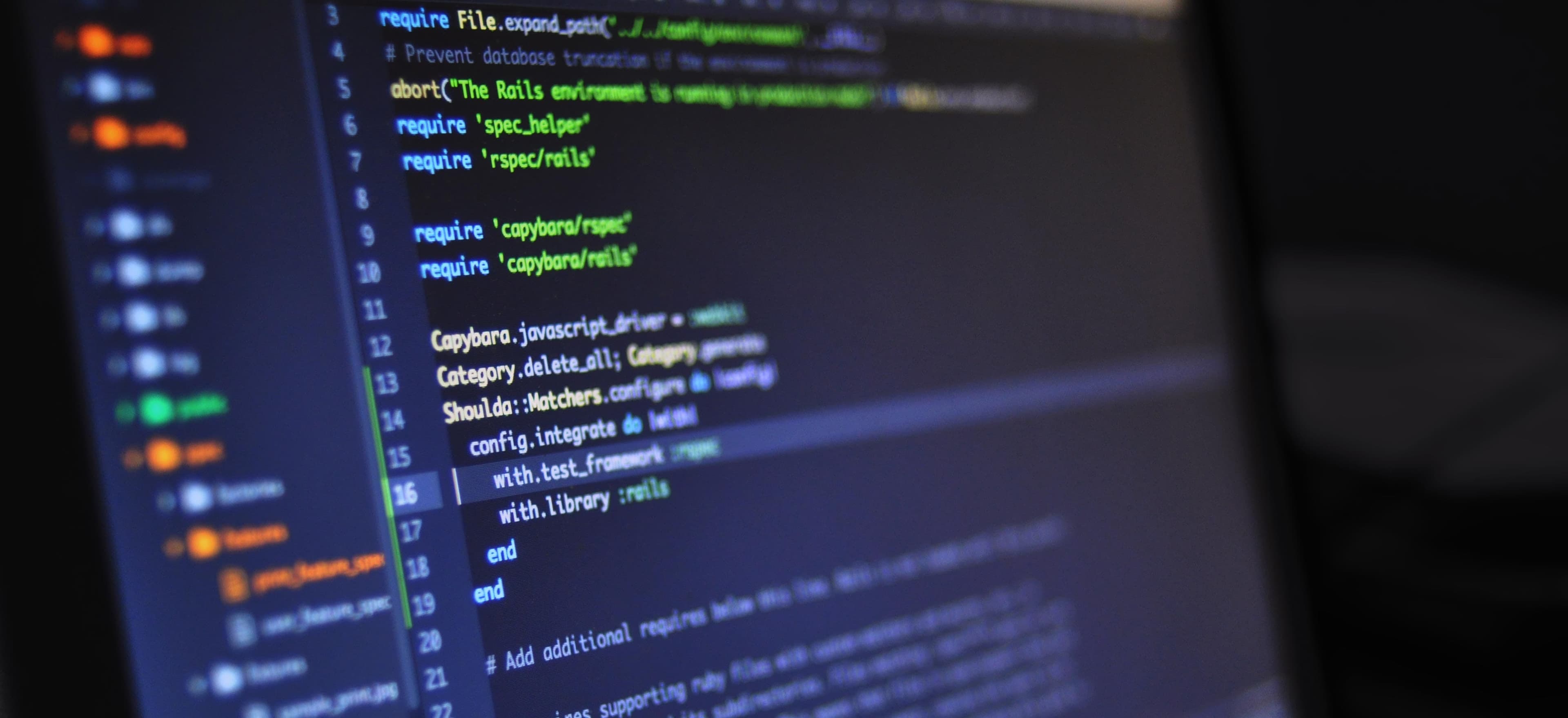
- Published on
Mastering Spring WebFlux for Reactive Apps
In the realm of modern web application development, the demand for high-performance, non-blocking, and reactive systems has surged exponentially. Reactive programming offers a solution to this demand, allowing for scalable and resilient systems. Among the plethora of tools and frameworks available, Spring WebFlux stands out as a powerful and versatile choice for building reactive applications in the Java ecosystem.
What is Reactive Programming?
Reactive programming revolves around the concept of reacting to changes and events in real-time, providing an efficient way to handle a large number of concurrent users and data streams. In essence, it allows developers to build applications that are more responsive, resilient, and scalable.
Introducing Spring WebFlux
Spring WebFlux is the reactive-stack web framework from the Spring Framework team, offering an alternative to the traditional Spring MVC framework. It is built on Project Reactor, a foundational library for building reactive applications. WebFlux provides native support for reactive programming, enabling developers to create non-blocking, asynchronous, and event-driven applications.
Benefits of Spring WebFlux
- Reactive and Non-blocking: Enables handling a large number of concurrent connections with a small number of threads.
- Scalability: Well-suited for applications requiring high throughput and low latency, especially in modern microservices architectures.
- Resilience: Automatic backpressure and efficient error handling mechanisms.
- Functional Endpoints: Support for both annotation-based and functional routing styles.
- Interoperability: Integration with existing Spring features, such as Spring Security and Spring Data.
Getting Started with Spring WebFlux
To leverage the power of Spring WebFlux, it is essential to understand the core components and features that make it a compelling choice for reactive application development.
1. Project Setup
Ensure that you have the necessary dependencies in your pom.xml
if you are using Maven or build.gradle
if you are using Gradle. Here is an example for Maven:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<!-- Add other dependencies as per your project requirements -->
</dependencies>
2. Creating Reactive Endpoints
Spring WebFlux provides two styles for defining endpoints: annotation-based and functional. Let's explore both approaches.
Annotation-based approach
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users/{id}")
public Mono<User> getUserById(@PathVariable String id) {
return userService.getUserById(id);
}
@PostMapping("/users")
public Mono<User> createUser(@RequestBody User user) {
return userService.createUser(user);
}
// Other endpoints...
}
Functional routing approach
@Configuration
public class UserRouter {
@Bean
public RouterFunction<ServerResponse> userRoutes(UserHandler userHandler) {
return RouterFunctions.route()
.GET("/users/{id}", userHandler::getUser)
.POST("/users", userHandler::createUser)
// Other routes...
.build();
}
}
In the annotation-based approach, @RestController
and HTTP method annotations are used to define endpoints. Alternatively, the functional routing approach leverages RouterFunction
and HandlerFunction
for a more programmatic and functional style of defining routes.
3. Working with Reactive Data Access
In a reactive application, it is crucial to utilize reactive repositories for interacting with databases. Spring Data provides excellent support for this through its reactive repositories.
public interface UserRepository extends ReactiveCrudRepository<User, String> {
// Custom queries can be defined here
}
By extending ReactiveCrudRepository
, you gain access to reactive database operations. This includes non-blocking, asynchronous operations for reading and writing data.
Advanced Techniques with Spring WebFlux
To truly master Spring WebFlux, it is imperative to delve into advanced techniques and best practices for building robust and efficient reactive applications.
1. Reactive Streams and Backpressure
Understanding reactive streams and backpressure is crucial when dealing with asynchronous, non-blocking, and backpressure-aware systems. Project Reactor provides the necessary abstractions for working with reactive streams, including Flux
and Mono
.
Flux<Integer> numbers = Flux.range(1, 1000)
.filter(i -> i % 2 == 0)
.map(i -> i * 2)
.onBackpressureBuffer(100, BufferOverflowStrategy.DROP_OLDEST);
In this example, a reactive stream of even numbers is created with backpressure handling using the onBackpressureBuffer
operator, ensuring that the downstream can handle the data at its own pace.
2. Error Handling and Resilience
Reactive applications necessitate robust error handling mechanisms. Project Reactor provides operators for handling errors, such as onErrorResume
, onErrorReturn
, and retry
. These mechanisms play a vital role in ensuring the resilience of reactive systems in the face of failures.
Flux<Integer> numbers = Flux.just(1, 2, 3, 4)
.map(i -> {
if (i % 2 == 0) {
return i;
} else {
throw new RuntimeException("Odd number encountered");
}
})
.onErrorResume(e -> Flux.just(-1));
In this example, the onErrorResume
operator is used to emit a default value in case of an error occurring in the Flux stream.
3. WebFlux Security
Securing reactive applications is critical, and Spring Security seamlessly integrates with Spring WebFlux to provide robust security features. From authentication to authorization, Spring Security offers extensive support for securing reactive endpoints and applications.
@EnableWebFluxSecurity
public class WebSecurityConfig {
@Bean
public SecurityWebFilterChain securityWebFilterChain(ServerHttpSecurity http) {
return http.authorizeExchange()
.pathMatchers("/admin/**").hasRole("ADMIN")
.anyExchange().authenticated()
.and().build();
}
}
This configuration example showcases how to define security rules for different endpoints based on roles and authentication requirements.
Lessons Learned
Mastering Spring WebFlux is a pivotal skill for building high-performance, resilient, and scalable reactive applications in the Java ecosystem. By understanding the fundamental principles of reactive programming, harnessing the power of Spring WebFlux's core components, and exploring advanced techniques, developers can elevate their proficiency in building modern and responsive web applications.
Embracing the reactive paradigm with Spring WebFlux empowers developers to conquer the challenges posed by today's demanding performance and scalability requirements, ultimately leading to the creation of robust and efficient applications.
So, harness the power of reactive programming, elevate your skills with Spring WebFlux, and embark on a journey to build the next generation of reactive applications in Java.