Overcoming WebCrypto API Limits for Secure E-Signatures
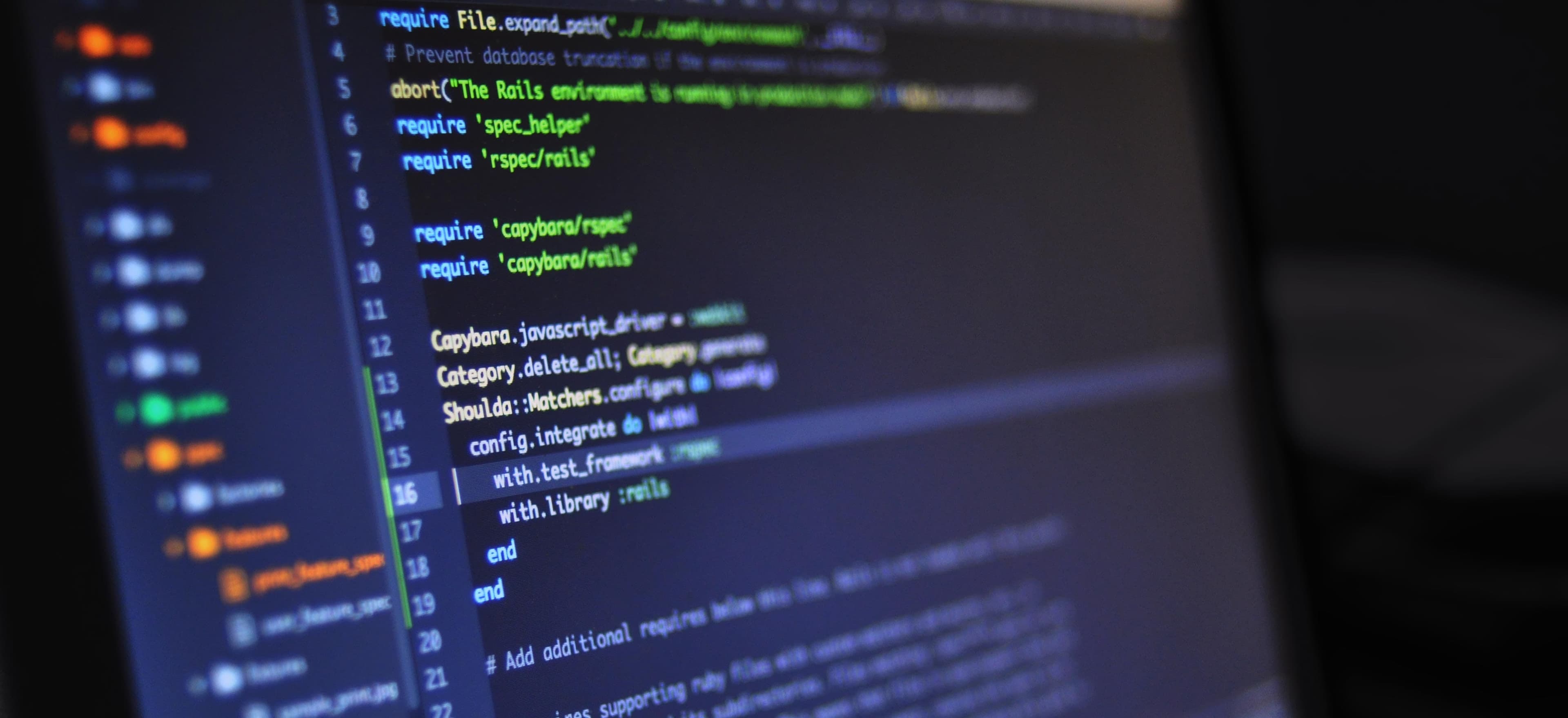
- Published on
Overcoming WebCrypto API Limits for Secure E-Signatures
In the era of digitalization, electronic signatures have become increasingly popular for their convenience and security. The WebCrypto API, a JavaScript API for performing basic cryptographic operations, offers a foundation for implementing secure electronic signatures within web applications. However, the WebCrypto API has certain limitations that developers must overcome to ensure robust and secure e-signature functionality.
In this article, we will explore the challenges posed by the WebCrypto API when implementing secure electronic signatures and provide solutions to overcome these limitations.
Understanding the WebCrypto API
The WebCrypto API provides a set of low-level cryptographic primitives that enable developers to perform cryptographic operations such as encryption, decryption, signing, and verification within the confines of a web browser. These operations are crucial for implementing secure electronic signatures in web applications.
Challenges with WebCrypto API for E-Signatures
While the WebCrypto API offers essential cryptographic functionalities, it presents challenges when it comes to implementing secure electronic signatures. Some of the key challenges include:
-
Limited Algorithm Support: The WebCrypto API has limited support for cryptographic algorithms, which may restrict the ability to use certain algorithms preferred for electronic signatures, such as RSA with SHA-256.
-
Key Generation and Storage: The generation and secure storage of cryptographic keys are essential for electronic signatures. However, the WebCrypto API's key generation and storage capabilities are limited, potentially impacting the generation of secure key pairs for signing operations.
-
Cross-Browser Compatibility: Ensuring consistent behavior and support across different web browsers is critical for web applications. The WebCrypto API's support and behavior may vary across different browsers, posing challenges for achieving cross-browser compatibility.
Overcoming WebCrypto API Limits for E-Signatures
To address the challenges posed by the WebCrypto API when implementing secure electronic signatures, developers can employ various strategies and best practices. Let's explore some effective approaches to overcome these limitations.
1. Algorithm Flexibility with WebCrypto SubtleCrypto
The SubtleCrypto
interface within the WebCrypto API provides access to a wide range of cryptographic functions, including signing and verification. While the API's direct support for specific algorithms may be limited, developers can leverage the SubtleCrypto
interface to implement custom algorithms or workarounds for electronic signatures.
// Example: Creating an RSA-SHA256 signature using SubtleCrypto
async function createRSASignature(privateKey, data) {
const signature = await crypto.subtle.sign(
{
name: "RSA-PSS",
saltLength: 20,
},
privateKey,
data
);
return new Uint8Array(signature);
}
In the above example, the SubtleCrypto
interface is utilized to create an RSA-SHA256 signature, overcoming the limitation of direct support for this algorithm within the WebCrypto API.
2. Key Management with IndexedDB
To address the challenge of key generation and storage, developers can utilize IndexedDB, a web API for storing large amounts of structured data client-side. By leveraging IndexedDB, developers can securely store cryptographic keys within the browser, ensuring their availability for electronic signature operations while maintaining a high level of security.
// Example: Storing cryptographic keys in IndexedDB
async function storeKeyInIndexedDB(key) {
const db = await indexedDB.open("cryptoKeys", 1);
const tx = db.transaction("keys", "readwrite");
tx.objectStore("keys").put(key, "signatureKey");
await tx.complete;
db.close();
}
The above code snippet demonstrates how cryptographic keys can be stored in IndexedDB, providing a solution for secure key management within web applications.
3. Polyfill and Feature Detection for Cross-Browser Compatibility
To ensure consistent behavior across different web browsers, developers can utilize polyfills and feature detection techniques to address variations in WebCrypto API support. Polyfills can fill the gaps in functionality by providing emulated implementations of features not natively supported by certain browsers, while feature detection allows developers to adapt their code based on the browser's capabilities.
// Example: Polyfill for WebCrypto API in browsers lacking support
if (!window.crypto || !window.crypto.subtle) {
window.crypto = window.crypto || {};
window.crypto.subtle = customSubtleCryptoPolyfill;
}
The above example illustrates how a polyfill can be used to support WebCrypto API functionality in browsers that lack native support, ensuring consistent behavior for electronic signature implementation.
Closing Remarks
Implementing secure electronic signatures within web applications using the WebCrypto API comes with its challenges, ranging from algorithm limitations to key management and cross-browser compatibility. By adopting strategies such as leveraging the SubtleCrypto
interface, utilizing IndexedDB for key storage, and addressing cross-browser compatibility through polyfills and feature detection, developers can overcome these limitations and ensure robust and secure e-signature functionality.
As digital transactions continue to grow, overcoming these challenges is essential for enabling secure and reliable electronic signatures in web applications.
To summarize, the WebCrypto API offers essential cryptographic capabilities, but it comes with limitations when implementing secure electronic signatures in web applications. Developers can overcome these limitations by leveraging the SubtleCrypto interface, utilizing IndexedDB for key storage, and addressing cross-browser compatibility through polyfills and feature detection. By doing so, they can ensure robust and secure e-signature functionality, contributing to the advancement of digital transactions.