Streamline Java EE: Master Continuous Delivery with Docker
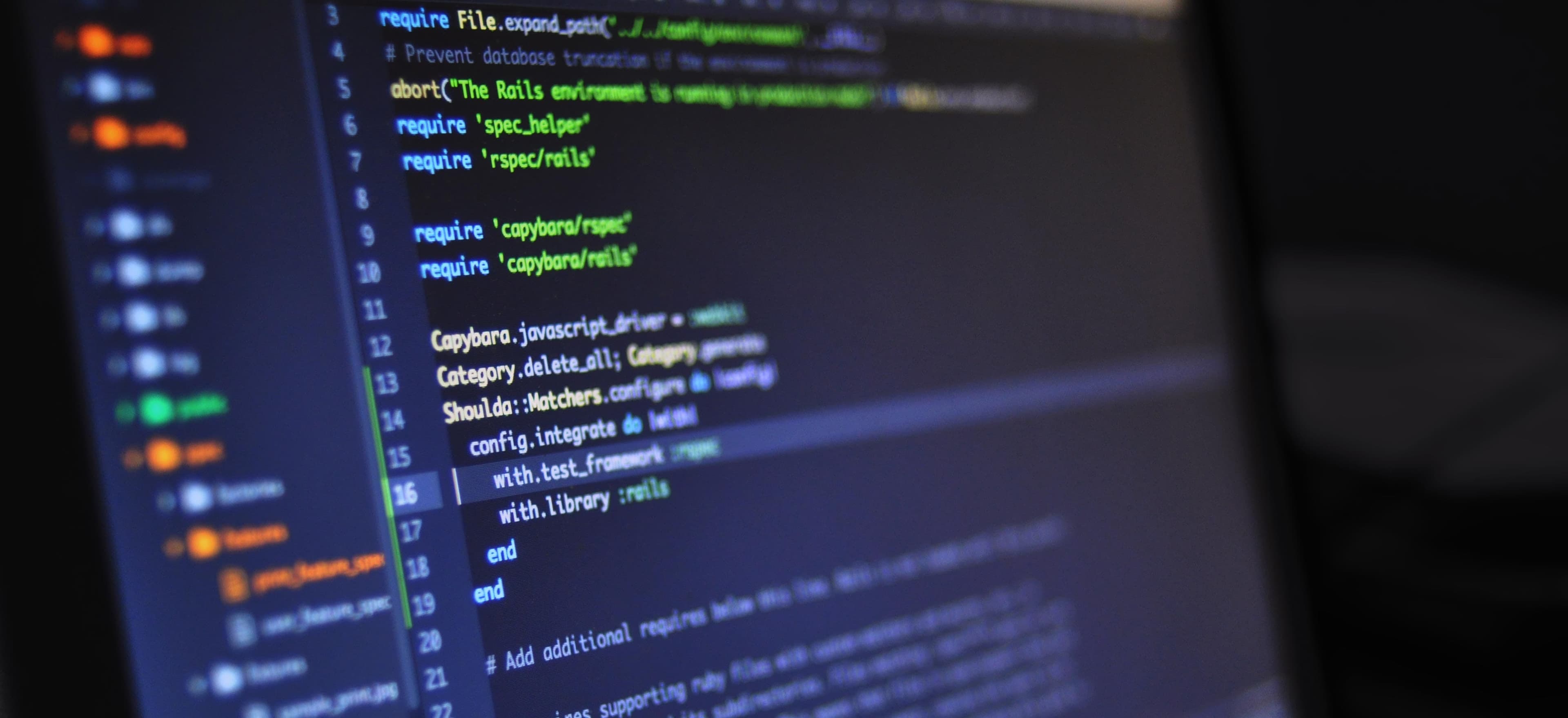
- Published on
Mastering Continuous Delivery with Docker in Java EE
Continuous Delivery (CD) has become a cornerstone of modern software development practices, allowing teams to release high-quality code frequently and efficiently. When it comes to Java Enterprise Edition (EE) applications, leveraging CD with Docker can significantly streamline the deployment process and enhance the overall development lifecycle.
In this article, we'll explore how Java developers can master Continuous Delivery with Docker, enabling them to deliver robust, scalable, and reliable Java EE applications seamlessly.
Embracing Continuous Delivery in Java EE
Continuous Delivery is a software engineering approach in which teams produce software in short cycles, ensuring that the software can be reliably released at any time. In the context of Java EE development, implementing Continuous Delivery involves automating the build, test, and deployment processes, enabling the rapid and consistent delivery of high-quality software.
Leveraging Docker for Seamless Containerization
Docker has revolutionized the way applications are packaged, shipped, and deployed. By utilizing Docker containers, Java EE applications can be isolated from their environment, ensuring consistency across various deployment stages, from development to production. Docker containers also enable seamless scalability and portability, which are essential aspects of modern Java EE application deployment.
Implementing Continuous Delivery with Docker in Java EE
Step 1: Dockerizing the Java EE Application
The first step in mastering Continuous Delivery with Docker in Java EE is dockerizing the application. This involves creating a Docker image that encapsulates the Java EE application and its dependencies. Below is a Dockerfile for containerizing a simple Java EE application:
# Start with a base image
FROM openjdk:11
# Set the working directory in the container
WORKDIR /app
# Copy the application JAR file into the container
COPY target/my-java-ee-app.jar /app
# Define the command to run the application
CMD ["java", "-jar", "my-java-ee-app.jar"]
In this Dockerfile, we start with a base OpenJDK image, set the working directory, copy the Java EE application JAR file, and define the command to run the application. This encapsulates the Java EE application within a Docker container, ensuring consistency and portability.
Step 2: Automating the Build and Deployment Process
To achieve Continuous Delivery with Docker in Java EE, automating the build and deployment process is crucial. Tools such as Jenkins, GitLab CI/CD, and Travis CI can be utilized to automate the build, test, and deploy pipeline. Incorporating Docker within the CI/CD pipeline enables seamless containerization and deployment of Java EE applications.
For example, a Jenkins pipeline can include stages for building the application, creating the Docker image, and deploying it to a container registry. Below is a simplified Jenkinsfile demonstrating the integration of Docker within the Continuous Delivery pipeline:
pipeline {
agent any
stages {
stage('Build') {
steps {
// Build the Java EE application
sh 'mvn clean package'
}
}
stage('Build Docker Image') {
steps {
// Build the Docker image
sh 'docker build -t my-java-ee-app .'
}
}
stage('Deploy to Registry') {
steps {
// Push the Docker image to a container registry
withDockerRegistry(credentialsId: 'docker-hub', url: 'https://registry.hub.docker.com') {
sh 'docker push my-java-ee-app'
}
}
}
}
}
In this Jenkinsfile, we define stages for building the application, building the Docker image, and deploying it to a container registry, highlighting the seamless integration of Docker within the Continuous Delivery pipeline.
Step 3: Orchestration with Docker Compose and Kubernetes
Once the Java EE application is containerized and the CI/CD pipeline is established, orchestrating and managing the Docker containers becomes pivotal. Docker Compose and Kubernetes are popular tools for orchestrating and managing Docker containers in a production environment.
Docker Compose allows developers to define and run multi-container Docker applications, simplifying the process of orchestrating interconnected Java EE components. On the other hand, Kubernetes provides a robust platform for automating deployment, scaling, and operations of application containers across clusters of hosts.
Understanding how Docker Compose and Kubernetes can be leveraged to orchestrate and manage Java EE applications in a containerized environment is essential for mastering Continuous Delivery with Docker in Java EE.
Wrapping Up
Continuous Delivery with Docker empowers Java EE developers to streamline the build, test, and deployment processes, ultimately leading to faster and more reliable software releases. By dockerizing Java EE applications, automating the CI/CD pipeline, and orchestrating containers with tools like Docker Compose and Kubernetes, developers can master Continuous Delivery with Docker, ensuring the seamless deployment of robust Java EE applications.
In conclusion, mastering Continuous Delivery with Docker in Java EE not only enhances the development lifecycle but also enables teams to deliver value to users in a timely and efficient manner, thereby staying ahead in today’s competitive software development landscape.
Embracing Continuous Delivery in Java EE and leveraging Docker for seamless containerization are essential steps toward achieving the goal of delivering high-quality, scalable, and reliable Java EE applications.
Continuous Delivery with Docker: Check out this comprehensive guide on Continuous Delivery with Docker to delve deeper into the integration of Docker within the Continuous Delivery pipeline.
Dockerize a Java EE Application: This tutorial provides a step-by-step guide on Dockerizing a Java EE application, further enhancing your understanding of Docker in Java EE development.
Mastering Kubernetes: Explore this in-depth resource on mastering Kubernetes to gain insights into orchestrating and managing Java EE applications in a containerized environment.
Make sure to check out these additional resources to dive deeper into mastering Continuous Delivery with Docker in Java EE!