Mastering Java for Android: Avoid Common Pitfalls
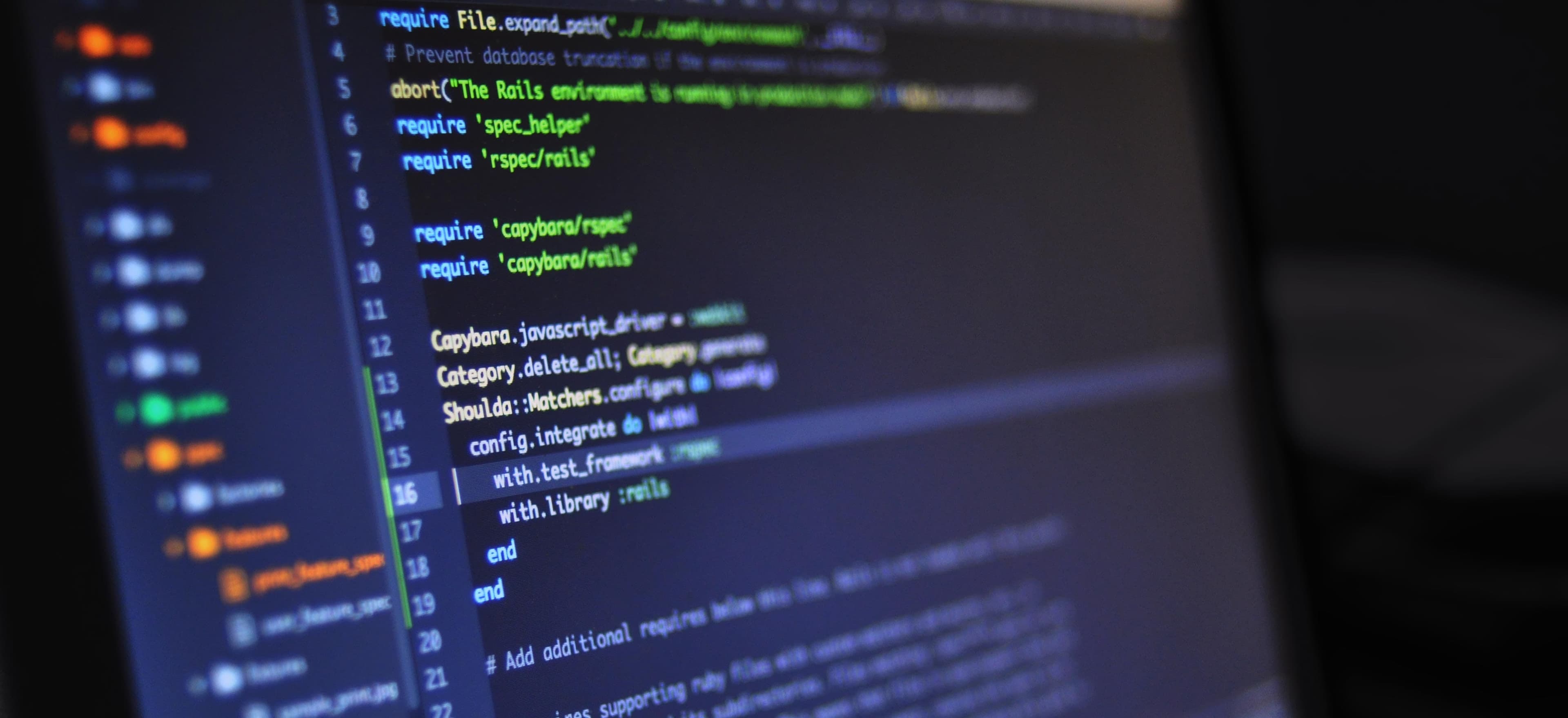
- Published on
Mastering Java for Android: Avoid Common Pitfalls
Java has been the primary language for Android development since the platform's inception. It's essential for Android developers to master Java to build efficient, reliable, and high-performance mobile applications. However, mastering Java involves avoiding common pitfalls that can lead to bugs, crashes, or performance issues in Android apps. In this post, we'll explore some of the most common pitfalls in Java for Android and how to avoid them.
Using Proper Exception Handling
One of the common pitfalls in Java for Android is inadequate exception handling. Proper exception handling is crucial for building robust and fault-tolerant Android applications. It's essential to handle exceptions gracefully to prevent app crashes and provide meaningful error messages to users.
Example:
try {
// Code that may throw an exception
} catch (IOException e) {
// Handle IOException
} catch (Exception e) {
// Handle other exceptions
} finally {
// Clean up resources
}
In the above example, we use a try-catch-finally block to handle exceptions. It's important to catch specific exceptions and handle them appropriately. Additionally, the finally block ensures that any required cleanup code is executed, regardless of whether an exception is thrown.
It's also important to avoid catching generic exceptions like Exception
without specific handling, as it can make debugging more challenging and lead to unexpected behavior.
Memory Management and Resource Handling
Memory management is critical in Android development to ensure that apps perform well and don't consume excessive resources. Improper memory management can lead to memory leaks, which can cause the app to slow down or even crash.
Example:
public void loadImage() {
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.image);
// Use the bitmap
// Don't forget to recycle the bitmap when finished
bitmap.recycle();
}
In the above example, we use the recycle()
method to release the memory associated with the bitmap after we're done with it. Failing to do so can lead to memory leaks and degrade app performance over time.
It's also essential to handle resources such as file streams, database connections, and network sockets properly by closing them when they're no longer needed to prevent resource leaks.
Optimizing Loops and String Manipulation
Inefficient loops and string manipulation can significantly impact the performance of Android apps. It's essential to write optimized code when working with loops and manipulating strings to avoid unnecessary overhead.
Example:
// Inefficient loop
for (int i = 0; i < list.size(); i++) {
// Perform some operation
}
// Optimized loop
for (int i = 0, size = list.size(); i < size; i++) {
// Perform the same operation
}
// Inefficient string concatenation
String result = "";
for (String str : listOfStrings) {
result += str;
}
// Optimized string concatenation
StringBuilder builder = new StringBuilder();
for (String str : listOfStrings) {
builder.append(str);
}
String result = builder.toString();
In the above example, we demonstrate optimized loop iteration by caching the size of the list outside the loop, which can improve performance, especially for large collections. Additionally, using StringBuilder
for string concatenation is more efficient than using the +
operator, especially when dealing with multiple concatenations.
Proper Threading and Concurrency
Android apps often require handling concurrent operations and background tasks. Improper threading and concurrency management can lead to bugs, race conditions, and inconsistent app behavior.
Example:
// Incorrect: Updating UI from a background thread
new Thread(() -> {
// Perform some background task
updateUI(); // This will cause an exception
}).start();
// Correct: Using runOnUiThread to update UI from a background thread
new Thread(() -> {
// Perform some background task
runOnUiThread(() -> {
// Update UI
});
}).start();
In the above example, updating the UI from a background thread directly will cause an exception. Instead, we should use runOnUiThread
to ensure that UI updates are performed on the main thread.
It's also important to use synchronization mechanisms such as synchronized
blocks or locks when accessing shared resources from multiple threads to prevent data corruption and race conditions.
Utilizing Proper Design Patterns
Using appropriate design patterns is crucial for building maintainable, scalable, and testable Android applications. Design patterns like MVC (Model-View-Controller), MVVM (Model-View-ViewModel), and Dependency Injection can help in organizing code and separating concerns.
Example:
// Using MVVM design pattern with LiveData and ViewModel
public class MyViewModel extends ViewModel {
private MutableLiveData<String> data = new MutableLiveData<>();
public LiveData<String> getData() {
return data;
}
public void updateData(String newData) {
data.setValue(newData);
}
}
In the above example, we use the MVVM pattern with LiveData
and ViewModel
to separate the UI logic from the business logic, leading to a more maintainable and testable codebase.
Utilizing design patterns not only improves code organization but also helps in achieving better code reusability and maintainability.
Final Considerations
Mastering Java for Android involves not only learning the language but also understanding and avoiding common pitfalls that can lead to issues in Android applications. By focusing on proper exception handling, memory management, optimized code, threading and concurrency management, and utilizing design patterns, developers can build high-quality Android apps that perform well and provide a great user experience.
Remember, mastering Java for Android is an ongoing process, and staying updated with best practices and new developments in the Android ecosystem is crucial for building successful Android applications.
By paying attention to these common pitfalls and best practices, developers can become proficient in Java for Android and build apps that stand out in the crowded app marketplace.
In the end, it's all about delivering a seamless and delightful user experience through well-crafted Java code in the world of Android development.
Checkout our other articles