Switching from Tomcat to Jetty in Spring Boot Made Easy
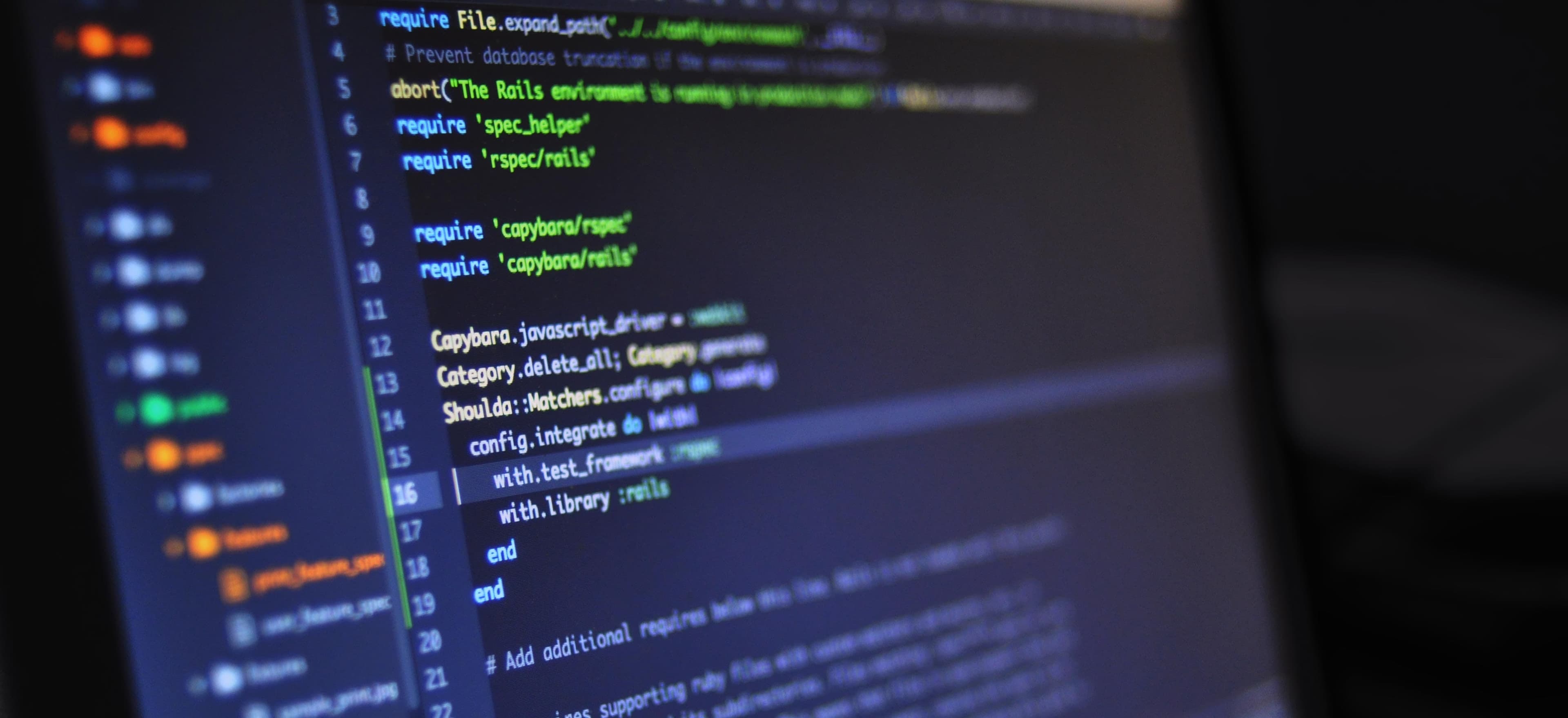
- Published on
Switching from Tomcat to Jetty in Spring Boot Made Easy
In the vast ocean of Java frameworks and application servers, Spring Boot has emerged as the lighthouse for Java developers. It offers a fast, configurable, and simple approach to kick-start applications, leaving the cumbersome XML configurations behind. Amongst its array of features, Spring Boot allows easy switching between different servlet containers. While Apache Tomcat rules the realm as the default choice, there’s another contender that’s been gaining ground for its performance, simplicity, and lightweight footprint: Eclipse Jetty. Today, we're diving deep into how you can switch from Tomcat to Jetty in your Spring Boot application, simplifying what might seem like a daunting process into manageable, easy-to-implement steps.
Why Switch to Jetty?
Before we dive into the "how," it's essential to understand the "why." Eclipse Jetty provides a robust, efficient, and flexible server and servlet engine. It’s designed for projects that require fewer resources, quick startup times, and are in need of embedding capabilities. Moreover, its lightweight nature doesn't compromise on performance, making it a compelling choice for microservices architecture and lightweight applications.
Step-by-Step Guide to Switching
Switching from Tomcat to Jetty in your Spring Boot application is simpler than you might think. All it takes is altering your pom.xml
or build.gradle
file and a few minor tweaks. Let’s break down the process.
1. Update Your Build Configuration
Spring Boot uses a set of starter dependencies to simplify your project setup. To make the switch, you need to exclude the spring-boot-starter-tomcat
dependency and include the spring-boot-starter-jetty
dependency.
For Maven Users:
If you're using Maven, open your pom.xml
file and make the following changes:
<dependencies>
<!-- Exclude Tomcat starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<!-- Add Jetty starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
</dependency>
</dependencies>
For Gradle Users:
In case you're leveraging Gradle, modify your build.gradle
file accordingly:
dependencies {
// Exclude Tomcat starter
implementation('org.springframework.boot:spring-boot-starter-web') {
exclude group: 'org.springframework.boot', module: 'spring-boot-starter-tomcat'
}
// Add Jetty starter
implementation 'org.springframework.boot:spring-boot-starter-jetty'
}
2. Understanding the Code Changes
What this does is instruct Spring Boot to stop auto-configuring Tomcat as the embedded server and to set up Jetty instead. It’s a seamless transition that requires no additional code. Spring Boot’s auto-configuration capabilities recognize the absence of Tomcat and the presence of Jetty and automatically configure your application to use Jetty as its embedded servlet container.
3. Performance Tuning and Configuration
It's always a good idea to fine-tune your server settings to ensure optimal performance. With Jetty, you can easily configure thread pools, port settings, and more through the application.properties
or application.yml
file in your Spring Boot project.
For example, to change the server port and configure the max thread pool size in application.properties
, you would add:
server.port=8080
server.jetty.threads.max=200
This simple configuration showcases Jetty's flexibility and ease of customization.
4. Testing the Switch
After making the switch, it’s critical to thoroughly test your application to ensure everything works as expected. Check the startup logs to confirm that Jetty is initialized. You should see logs indicating that Jetty is starting, similar to:
Started Jetty Server
Running a series of integration and smoke tests can help verify the seamless operation of your application on its new servlet container.
Additional Resources
For more in-depth knowledge and advanced configuration options, the official Spring Boot documentation (Spring Boot Reference Guide) and the Eclipse Jetty documentation (Eclipse Jetty Documentation) are invaluable resources. They provide comprehensive guides on everything from basic setup to advanced features and performance tuning.
The Bottom Line
Switching from Tomcat to Jetty in a Spring Boot application isn’t just feasible; it’s straightforward with Spring Boot’s flexible architecture. Whether you’re looking for increased performance, reduced resource consumption, or simply exploring alternatives, transitioning to Jetty offers tangible benefits with minimal hassle. By following the steps outlined above, you can make the switch smoothly and take advantage of Jetty's robust features in your Spring Boot applications. Happy coding!
Remember, the choice of servlet container can significantly impact your application's performance and resource utilization. Hence, evaluating the needs and constraints of your project before making a switch is crucial. However, should Jetty be the path you choose, rest assured the process is simpler than it seems, setting the stage for a lightweight, high-performance application environment.
Checkout our other articles