Cracking Data Joins in MapReduce: A Beginner's Guide
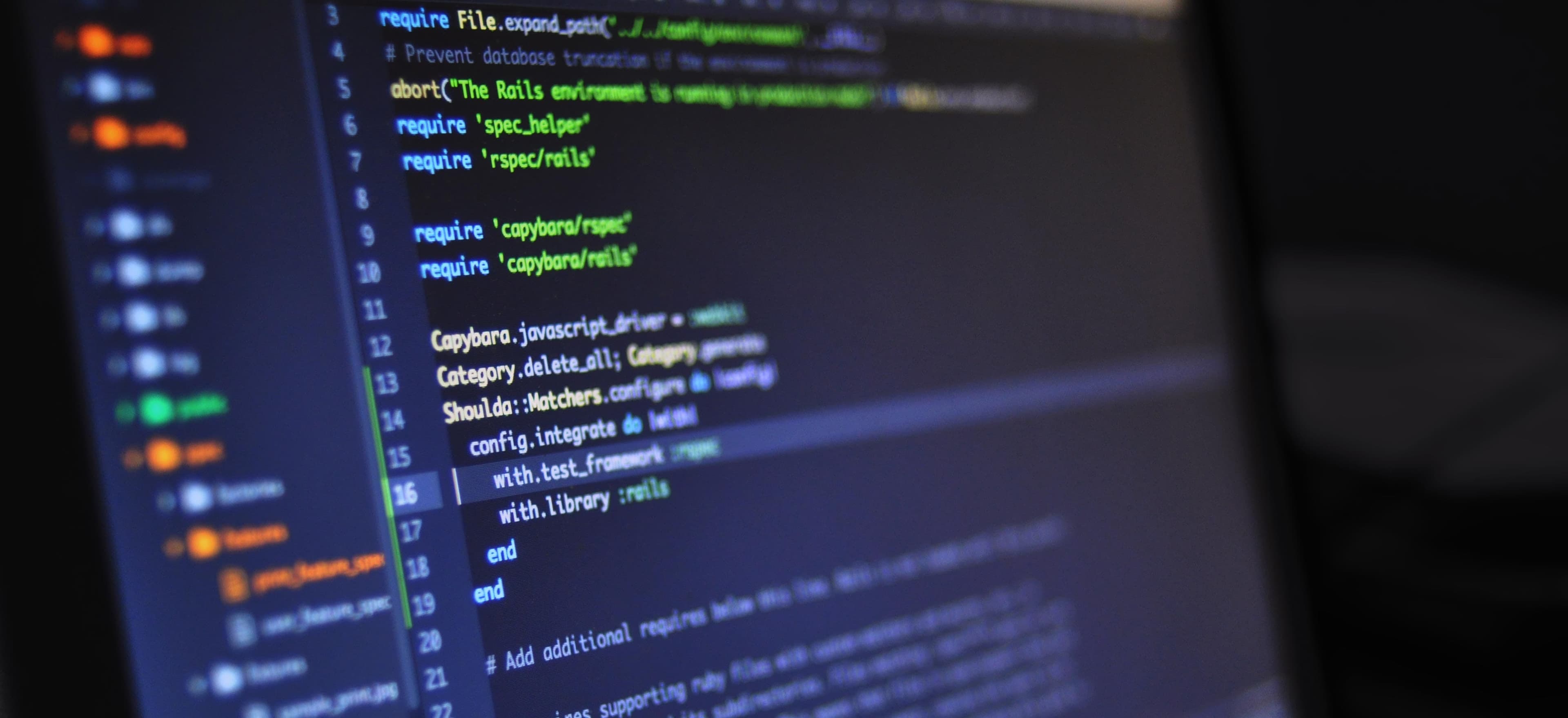
- Published on
Cracking Data Joins in MapReduce: A Beginner's Guide
Data joins are fundamental operations in big data processing. They involve combining data from multiple sources based on a common field. In the context of MapReduce, data joins can be tricky due to the distributed nature of the processing. In this blog post, we will explore how to crack data joins in MapReduce using Java.
Understanding Data Joins
In the world of big data, data joins are essential for combining datasets that reside across multiple machines or nodes. There are several types of data joins, including inner joins, outer joins, left joins, and right joins, each serving a specific purpose in data consolidation.
In MapReduce, data joins are performed by dividing the input datasets into key-value pairs, where the keys serve as the join attributes. These key-value pairs are then distributed across the mapper nodes for parallel processing, leading to significant performance gains.
MapReduce Data Join Techniques
Map-Side Joins
Map-side joins are a type of data join in which the join operation is performed before the data reaches the reducer. This technique is highly efficient as it minimizes the data transfer between the mappers and reducers.
Let's consider an example where we have two datasets A
and B
that we want to join based on a common key. We can leverage map-side joins by ensuring that both datasets are partitioned and sorted based on the join key before being passed through the mappers. In this scenario, the mappers can process the join locally without the need to shuffle data across the network.
Reduce-Side Joins
Reduce-side joins involve performing the join operation during the reduce phase of MapReduce. In this approach, the mappers emit key-value pairs, which are then shuffled and sorted by the framework before being passed to the reducers. The reducers are responsible for combining the values associated with the same key to accomplish the join.
Implementing MapReduce Data Joins in Java
To illustrate the process of cracking data joins in MapReduce using Java, let's consider a simple example of performing an inner join between two datasets using the map-side join technique.
Setting up the Project
First, we need to set up a Maven project and include the necessary dependencies for Hadoop in the pom.xml
file.
<dependencies>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-client</artifactId>
<version>3.3.1</version>
</dependency>
</dependencies>
Writing the Mapper
We will start by implementing the mapper, where the map-side join logic will be applied.
public class JoinMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text joinKey = new Text();
private Text value = new Text();
@Override
protected void map(LongWritable key, Text input, Context context) throws IOException, InterruptedException {
// Split the input into key and value
String[] parts = input.toString().split(",");
joinKey.set(parts[0]);
value.set(parts[1]);
context.write(joinKey, value);
}
}
In this mapper, we extract the join key from the input and emit it as the output key, along with the rest of the value as the output value.
Writing the Reducer
Next, we need to implement the reducer responsible for performing the join operation.
public class JoinReducer extends Reducer<Text, Text, Text, Text> {
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
List<String> datasetA = new ArrayList<>();
List<String> datasetB = new ArrayList<>();
for (Text value : values) {
// Populate datasetA and datasetB based on the source of the value
if (isFromDatasetA(value.toString())) {
datasetA.add(value.toString());
} else {
datasetB.add(value.toString());
}
}
// Perform the join operation
for (String a : datasetA) {
for (String b : datasetB) {
context.write(key, new Text(a + "," + b));
}
}
}
private boolean isFromDatasetA(String value) {
// Determine if the value is from dataset A
// Add logic here based on the structure of the datasets
return true;
}
}
In the reducer, we iterate through the values associated with the same key and populate separate lists for the two datasets. Once the lists are populated, we perform the join operation and emit the joined key-value pairs.
Configuring the Job
Finally, we configure the MapReduce job by setting the input paths, output paths, mapper, reducer, and input/output formats.
public class JoinDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "MapSideJoinExample");
// Set input and output paths
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
// Set mapper and reducer classes
job.setMapperClass(JoinMapper.class);
job.setReducerClass(JoinReducer.class);
// Set input and output formats
job.setInputFormatClass(TextInputFormat.class);
job.setOutputFormatClass(TextOutputFormat.class);
// Set output key and value classes
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
In the JoinDriver
class, we configure the MapReduce job by specifying the input and output paths, mapper, reducer, input/output formats, and output key-value classes.
Wrapping Up
Data joins in MapReduce are powerful techniques for consolidating data from disparate sources. By understanding and implementing map-side and reduce-side joins in Java, you gain the ability to efficiently process large datasets and derive valuable insights from them.
By following this beginner's guide, you have learned the fundamentals of cracking data joins in MapReduce, along with a practical example of implementing an inner join using the map-side join technique in Java.
In future projects, you can explore more advanced join techniques, such as distributed cache joins and composite join strategies, to further enhance your data processing capabilities in MapReduce.
In conclusion, mastering data joins in MapReduce can significantly elevate your big data processing skills and pave the way for solving complex data integration challenges in the real world.
For more in-depth understanding and practical examples, you can refer to the official Apache Hadoop documentation and examples provided in the Hadoop GitHub repository.
Start your journey of mastering data joins in MapReduce today and unleash the power of distributed data processing with Java!