Mocking Made Easy: Streamline Your Database Tests!
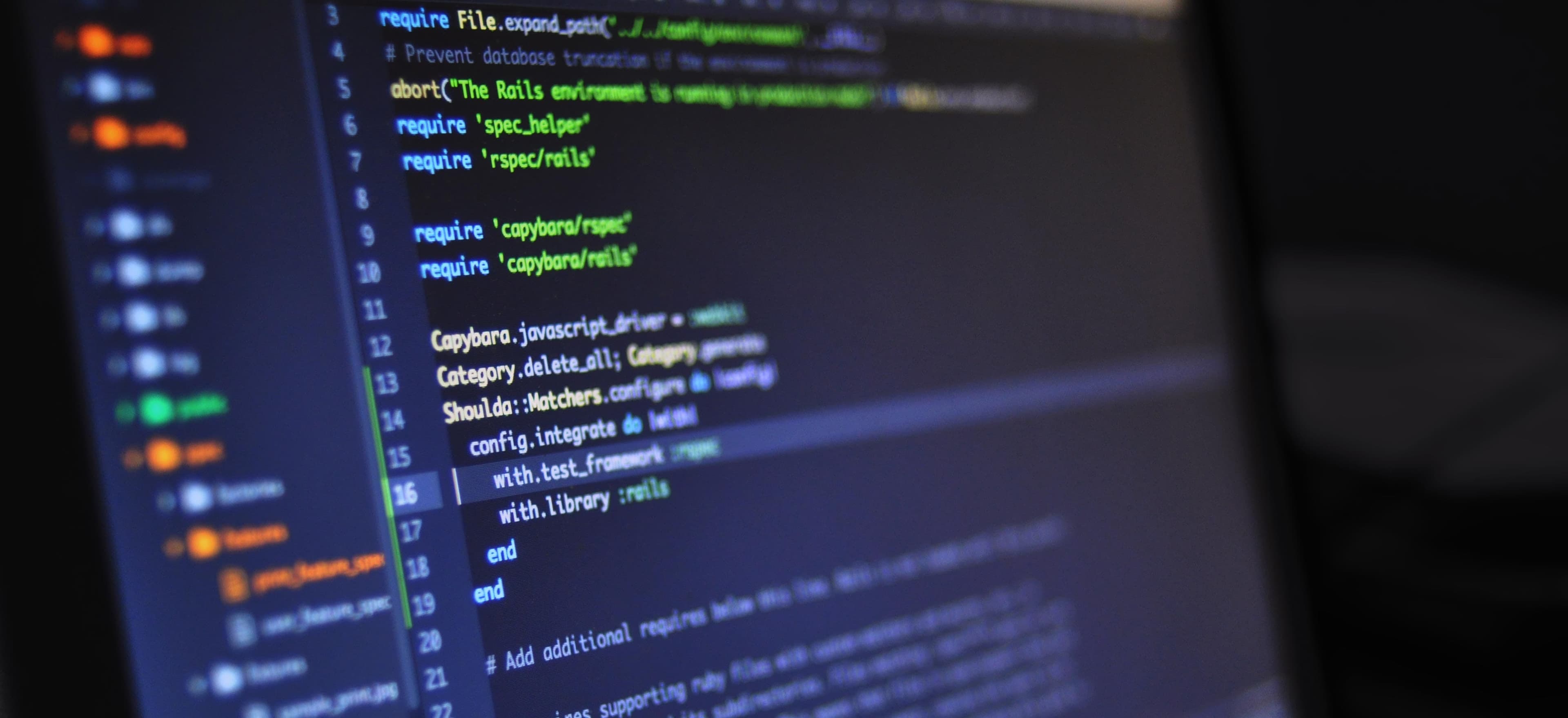
- Published on
Mocking Made Easy: Streamline Your Database Tests!
When it comes to writing tests for your Java applications, efficient database testing is essential for ensuring the reliability and stability of your code. However, interacting with an actual database during tests can be slow, complex, and potentially unreliable. This is where mocking comes to the rescue, allowing you to simulate the behavior of external dependencies such as a database. In this post, we'll explore how to streamline your database tests using mocking in Java, specifically focusing on the Mockito framework.
Why Use Mocking for Database Testing?
Mocking is a powerful technique in software testing that enables you to create mock objects that simulate the behavior of real objects. When it comes to database testing, using actual database instances for every test can lead to several drawbacks, including:
- Slow Execution: Interacting with a real database can significantly slow down the execution of your test suite, affecting developer productivity.
- Complex Setup: Setting up and tearing down a database for each test can lead to overly complex and brittle test code.
- Unreliability: Tests that rely on a real database can be unreliable due to factors such as network connectivity, database state, or data corruption.
By using mocking, you can create lightweight, controlled representations of the database behavior, enabling faster, more predictable, and maintainable tests.
Introducing Mockito for Mocking
Mockito is a popular mocking framework for Java that provides a simple and flexible API for creating and working with mock objects. It allows you to define the behavior of dependencies, verify interactions, and inject mocks into your code seamlessly.
Let's dive into a practical example to demonstrate how Mockito can be used to streamline database testing in Java.
Setting Up the Project
First, ensure that you have the Mockito dependency included in your project. If you are using Maven, simply add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.12.4</version>
<scope>test</scope>
</dependency>
For Gradle, include the following dependency in your build.gradle
file:
testImplementation 'org.mockito:mockito-core:3.12.4'
Writing Database Tests with Mockito
Suppose we have a UserService
class that interacts with a database to perform user-related operations. We want to test the functionality of this class without actually hitting the real database. In this scenario, we can utilize Mockito to mock the database interactions.
Here's a simplified version of the UserService
class:
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public boolean isUserActive(Long userId) {
User user = userRepository.findById(userId);
return user != null && user.isActive();
}
}
In this example, UserRepository
is an interface that provides methods for accessing user-related data from the database.
Now, let's create a test for the UserService
class using Mockito to mock the UserRepository
dependency.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testIsUserActive() {
// Create a mock UserRepository
UserRepository userRepository = mock(UserRepository.class);
// Create a test user
User activeUser = new User(1L, "John Doe", true);
// Stub the findById method to return the test user
when(userRepository.findById(1L)).thenReturn(activeUser);
// Create an instance of UserService with the mock UserRepository
UserService userService = new UserService(userRepository);
// Invoke the method under test
boolean isActive = userService.isUserActive(1L);
// Verify that the method interacts with the UserRepository
verify(userRepository).findById(1L);
// Assert the expected result
assertTrue(isActive);
}
}
In this test, we:
- Created a mock
UserRepository
usingmock(UserRepository.class)
. - Defined the behavior of the mock by stubbing the
findById
method to return a test user usingwhen(userRepository.findById(1L)).thenReturn(activeUser)
. - Instantiated the
UserService
with the mockUserRepository
. - Invoked the method under test to validate its behavior.
- Verified that the method interacts with the
UserRepository
usingverify(userRepository).findById(1L)
. - Asserted the expected result using JUnit assertions.
By using Mockito to mock the UserRepository
, we were able to isolate the UserService
class from the actual database, making the test faster, deterministic, and independent of the database state.
Bringing It All Together
In this post, we've explored the importance of using mocking to streamline database testing in Java. By leveraging the Mockito framework, you can effectively simulate database interactions, leading to faster, more reliable, and maintainable tests for your Java applications. Mocking allows you to focus on testing the actual business logic without being encumbered by the complexities of real database dependencies.
Mocking is a powerful tool in your testing arsenal, and mastering it can significantly improve the efficiency and effectiveness of your test suite. As you continue to refine your skills in Java development and testing, incorporating mocking into your testing strategy will undoubtedly be a valuable asset.
So go ahead, integrate mocking into your database tests, and experience the benefits of faster, more robust, and maintainable test suites for your Java applications!