Top Java Pitfalls: How to Learn Java Without Them
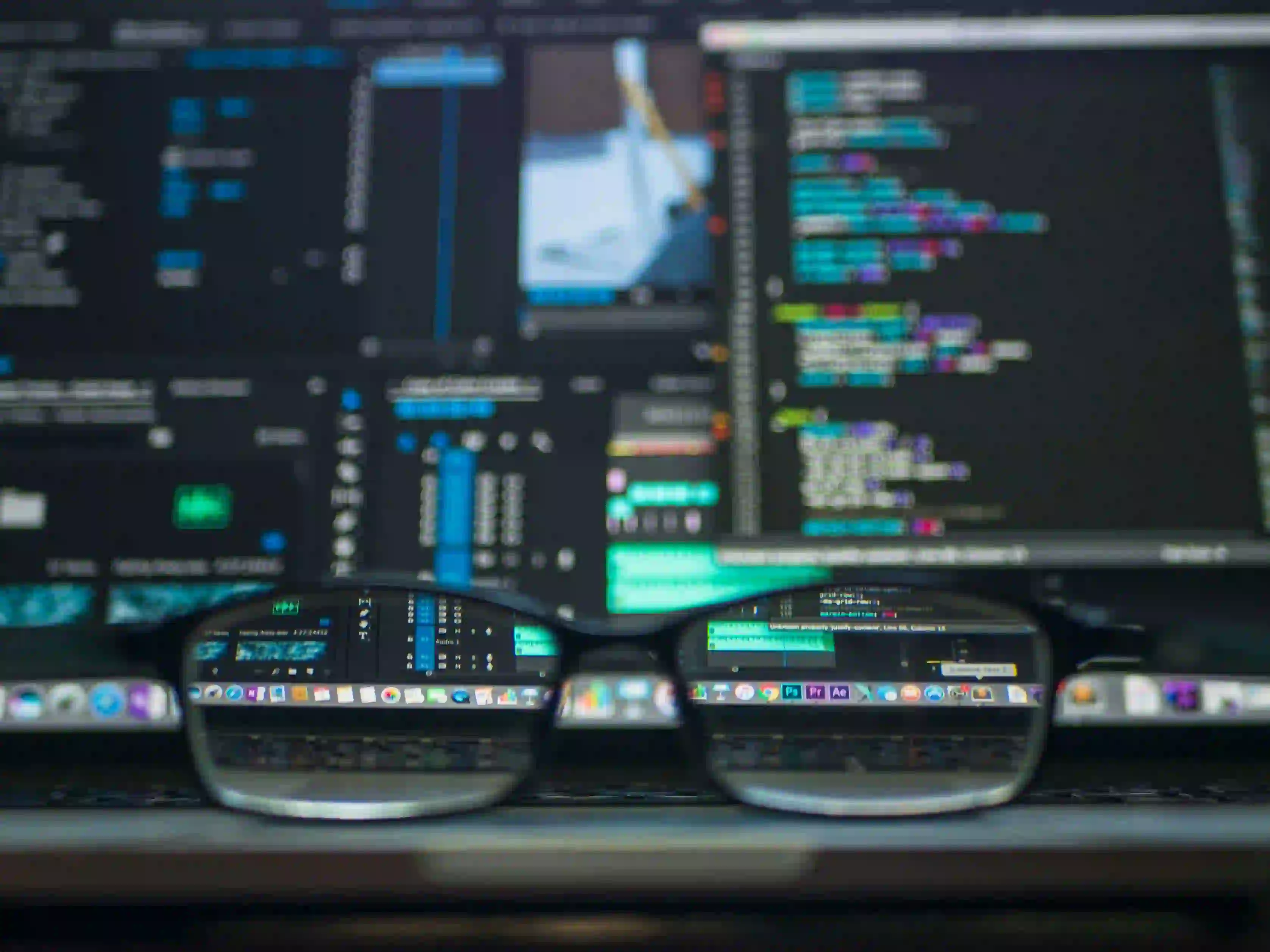
Top Java Pitfalls: How to Learn Java Without Them
Java is a powerful and versatile programming language that has been the backbone of countless applications and systems for many years. Whether you are a beginner or an experienced developer, understanding the common pitfalls in Java can save you a lot of time and frustration. In this blog post, we will explore some of the top Java pitfalls and provide tips on how to avoid them.
Not Understanding the Basics
One of the most common pitfalls for beginners is diving into Java without a solid understanding of the basics. It's crucial to grasp fundamental concepts such as variables, data types, control structures, and object-oriented programming principles. Without a strong foundation, you'll find yourself struggling to comprehend more advanced topics and debugging simple errors.
Code Example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In the above example, public
, class
, static
, and void
are all keywords in Java. Understanding their significance is crucial to writing and comprehending Java programs effectively.
Ignoring Object-Oriented Principles
Java is an object-oriented programming (OOP) language, and ignoring its OOP principles is a significant pitfall. Failure to grasp concepts like encapsulation, inheritance, and polymorphism can lead to writing inefficient and error-prone code. Embracing OOP principles not only makes your code more robust and maintainable but also aligns with best practices in Java development.
Mismanaging Memory
Java abstracts memory management through its automatic garbage collection process. However, improper memory handling, such as memory leaks and inefficient memory usage, can still occur. It's crucial to understand how memory is managed in Java and to avoid common memory pitfalls, such as holding references to objects unnecessarily or creating unnecessary objects.
Neglecting Exception Handling
Exception handling is essential for writing reliable and robust Java applications. Neglecting to handle exceptions properly can result in unexpected crashes and unpredictable behavior. By understanding and implementing effective exception handling, you can write code that gracefully handles errors and maintains application stability.
Code Example:
try {
// Code that may throw an exception
} catch (IOException e) {
// Handle the IOException
} finally {
// Code to be executed regardless of whether an exception is thrown
}
In this example, the try
block contains the code that may throw an exception, the catch
block handles the exception, and the finally
block ensures that certain code is executed regardless of whether an exception is thrown or not.
Overlooking Performance Considerations
Java's performance is often a key factor in the success of an application. It's vital to consider performance implications when writing Java code, such as avoiding unnecessary object creation, using efficient data structures, and optimizing algorithms. Overlooking performance considerations can lead to sluggish and resource-intensive applications.
Not Keeping Up with Java Best Practices
As Java evolves, best practices and coding conventions also evolve. Failing to keep up with the latest best practices can result in writing outdated and suboptimal code. Keeping abreast of new features, tools, and coding standards is essential for writing clean, efficient, and maintainable Java code.
The Closing Argument
Avoiding these top Java pitfalls is essential for any developer looking to master the Java programming language. By understanding the basics, embracing object-oriented principles, managing memory effectively, handling exceptions, considering performance, and staying updated on best practices, you can write high-quality Java code that is reliable, efficient, and maintainable.
Java is a vast and ever-growing language, and there will always be more to learn. However, by steering clear of these common pitfalls, you can build a strong foundation and continue to expand your Java expertise with confidence.
Remember, learning Java is a journey, and as with any journey, mistakes are bound to happen. However, with each mistake comes an opportunity to learn and grow as a Java developer.
Now, armed with the knowledge of these common pitfalls, go forth and code with confidence!
For a comprehensive guide on learning Java, check out Oracle's Java Tutorials.
Happy coding!