Overcoming Common Container Orchestration Challenges
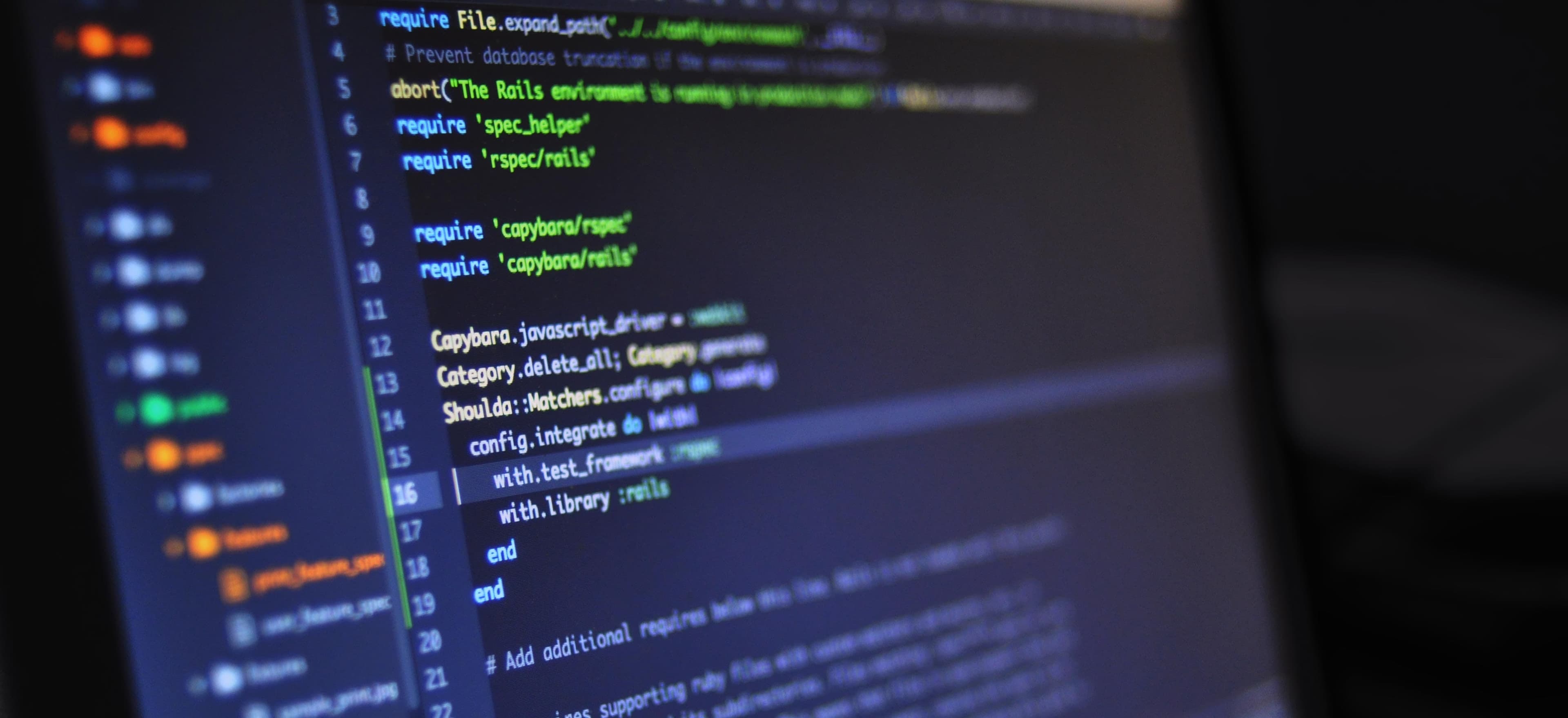
- Published on
Overcoming Common Container Orchestration Challenges
Container orchestration has become an essential part of modern application development and deployment. As organizations transition to microservices architectures, the complexities of managing these containerized applications grow. Despite the benefits, there are significant challenges that teams often face. This article explores the most common container orchestration challenges and offers strategies to overcome them.
Understanding Container Orchestration
Before delving into the challenges, let's briefly understand what container orchestration is. Container orchestration automates the deployment, scaling, and management of containerized applications. Popular tools like Kubernetes, Docker Swarm, and Apache Mesos provide the frameworks necessary for developers to efficiently manage their containers, but complexities can arise.
Challenge 1: Complexity of Architecture
Discussion
Microservices architecture is both a blessing and a curse. While it allows for the development of independent, scalable services, it also introduces complexity. Every microservice may require its own stack, which can make orchestration cumbersome.
Solution
To cope with this complexity, consider using a service mesh architecture. A service mesh simplifies communication between microservices without requiring changes to the service code itself. Tools like Istio and Linkerd can help manage communication, providing capabilities like load balancing and fault tolerance.
Example Code Snippet: Service Discovery with Kubernetes
Kubernetes offers built-in mechanisms for service discovery through its DNS system. Here is an example of how to define a service:
apiVersion: v1
kind: Service
metadata:
name: my-service
spec:
selector:
app: my-app
ports:
- protocol: TCP
port: 80
targetPort: 8080
Why? The above code creates a service named my-service
that will route traffic to the application pods labeled my-app
. This encapsulation helps developers access services without worrying about the location of the pods themselves.
Challenge 2: Scaling Applications
Discussion
Scaling containerized applications can be challenging. Both horizontal scaling (adding more replicas) and vertical scaling (adding more resources to a pod) have their complexities. Moreover, improper scaling can affect performance and costs.
Solution
Employ horizontal pod autoscaling (HPA). HPA scales the number of pods in a deployment or replication controller based on observed CPU utilization or other select metrics.
Example Code Snippet: Setting Up Horizontal Pod Autoscaling
apiVersion: autoscaling/v2beta2
kind: HorizontalPodAutoscaler
metadata:
name: my-app-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: my-app
minReplicas: 1
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 50
Why? This YAML file configures HPA for a deployment called my-app
. It scales the application between one and ten pods based on CPU usage, ensuring efficient resource use and performance.
Challenge 3: Monitoring and Logging
Discussion
As the number of containers increases, keeping an eye on their health becomes a daunting task. Busy development teams may find it challenging to track logs in real-time and respond to failures quickly.
Solution
Implement centralized logging and monitoring. Tools like Prometheus for monitoring and ELK (Elasticsearch, Logstash, Kibana) stack for logging can make data collection and visualization more manageable.
Example Code Snippet: Setting Up Prometheus on Kubernetes
apiVersion: v1
kind: Service
metadata:
name: prometheus
spec:
type: ClusterIP
ports:
- port: 9090
targetPort: 9090
selector:
app: prometheus
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: prometheus
spec:
replicas: 1
selector:
matchLabels:
app: prometheus
template:
metadata:
labels:
app: prometheus
spec:
containers:
- name: prometheus
image: prom/prometheus
args:
- "--config.file=/etc/prometheus/prometheus.yml"
ports:
- containerPort: 9090
Why? The code above sets up a Prometheus monitoring service within Kubernetes, allowing teams to monitor container metrics effectively, which is vital for scaling decisions and fault diagnosis.
Challenge 4: Security Vulnerabilities
Discussion
Security is another serious concern in container orchestration. Mapping network security, managing secrets, and ensuring containers run with the least privilege can be overwhelming.
Solution
Adopting a security-first approach is crucial. Use tools like Aqua Security or Twistlock to scan images for vulnerabilities before deploying. Implement Role-Based Access Control (RBAC) in Kubernetes to enforce the principle of least privilege.
Example Code Snippet: Kubernetes Role-Based Access Control
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: my-namespace
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
---
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: my-namespace
subjects:
- kind: User
name: alice
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
Why? This defines a Role and RoleBinding allowing a user named Alice to read pod resource specifications. It showcases how RBAC can help secure your Kubernetes environment.
Balancing Challenges with Best Practices
As organizations adopt container orchestration, it is vital to implement best practices to mitigate challenges. Here are a few recommended measures:
- Documentation: Maintain detailed documentation for every microservice, its endpoints, and dependencies.
- Automation: Automate as many aspects of deployment and monitoring as possible to reduce human error.
- Regular Audits: Conduct security audits to identify vulnerabilities early and adapt as necessary.
- Community Engagement: Engage with communities and forums. Resources like Kubernetes Documentation and Docker Documentation offer a treasure trove of knowledge.
Final Thoughts
Overcoming container orchestration challenges is an ongoing effort that requires adeptness and knowledge. By implementing best practices and leveraging the right tools, organizations can efficiently manage their containerized applications, yielding better performance and resilience.
As container orchestration technology continues to evolve, staying updated and adapting your strategies will be key to success.
For more in-depth examples and real-world applications, consider exploring the comprehensive resources available here and here.
Remember, the journey towards effective container orchestration is about continuous improvement, learning, and adaptation. Happy orchestrating!
Checkout our other articles