Migrating from ColdFusion to Java: Navigating Challenges
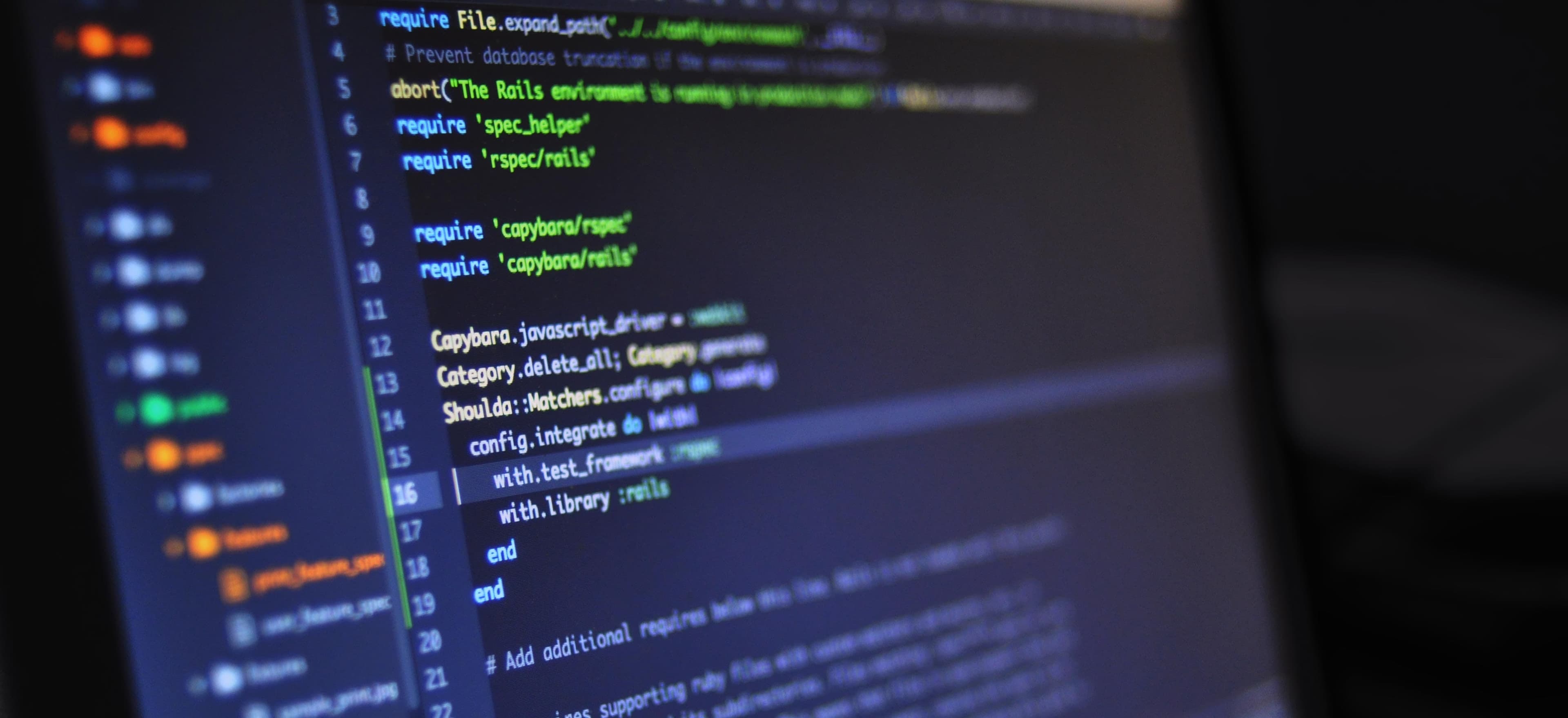
- Published on
Migrating from ColdFusion to Java: Navigating Challenges
Many organizations that have previously relied on ColdFusion for their web development are now considering migrating to Java due to its robust ecosystem and better performance. However, this transition is not without its challenges. In this article, we'll explore the key challenges involved in migrating from ColdFusion to Java and discuss strategies for overcoming them.
Understanding the Key Differences
One of the fundamental differences between ColdFusion and Java is that ColdFusion is a rapid application development platform, while Java is a general-purpose programming language. ColdFusion abstracts many of the complexities of web development, making it easier for developers to rapidly create web applications. On the other hand, Java provides greater flexibility and control but requires a deeper understanding of programming concepts.
Assessing the Codebase
Before diving into the migration process, it's essential to assess the existing ColdFusion codebase. This involves identifying the core functionalities, dependencies, and potential areas of refactoring. Understanding the existing codebase will help in planning a structured approach for the migration process.
Example: Assessing the Existing Codebase
// Sample code for assessing the existing ColdFusion codebase
// Identify core functionalities
// Check for dependencies
// Note potential areas of refactoring
In the example above, we can see a basic outline for assessing the existing ColdFusion codebase. This initial step is crucial for gaining insights into the code that needs to be migrated.
Handling Database Integration
ColdFusion has traditionally provided seamless integration with databases using its own query language, CFQuery. Migrating to Java would require transitioning to a more standardized approach such as Java Database Connectivity (JDBC) or an Object-Relational Mapping (ORM) framework like Hibernate.
Example: Database Integration in Java
// Using JDBC for database connection in Java
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
In the example above, we demonstrate the use of JDBC for connecting to a database in Java. This approach provides greater flexibility and control over database operations.
Adapting to Object-Oriented Programming
ColdFusion, being a tag-based language, has a different paradigm compared to Java, which is an object-oriented language. Migrating to Java would entail restructuring the codebase to adhere to object-oriented principles, such as encapsulation, inheritance, and polymorphism.
Example: Transitioning to Object-Oriented Code
// Refactoring ColdFusion code to adhere to object-oriented principles in Java
public class Employee {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
// Additional methods and properties...
}
In this example, we showcase the refactoring of ColdFusion code to conform to object-oriented principles in Java. This transition allows for better organization and maintainability of the codebase.
Addressing Template Engine Differences
ColdFusion utilizes its own templating language for server-side scripting, whereas Java typically relies on JavaServer Pages (JSP) or other templating engines such as Thymeleaf or FreeMarker. Adapting to the syntax and functionalities of these template engines is a significant aspect of the migration process.
Example: Using JavaServer Pages (JSP) for Templating
<!-- Sample JSP code for templating in Java -->
<!DOCTYPE html>
<html>
<head>
<title>Employee Details</title>
</head>
<body>
<h1>Welcome, <%= employee.getName() %></h1>
<!-- Additional templating content -->
</body>
</html>
In the example above, we illustrate the use of JavaServer Pages (JSP) for templating in Java. Understanding and adapting to the syntax of such template engines is crucial for a successful migration.
Ensuring Security Measures
Security considerations are paramount during the migration process. ColdFusion and Java have different security models and best practices. This necessitates a thorough review of the existing security measures in the ColdFusion codebase and the implementation of corresponding security measures in the Java environment.
Leveraging Frameworks and Libraries
Java offers a rich ecosystem of frameworks and libraries for various functionalities such as web development, database interaction, and security. Leveraging these frameworks and libraries can significantly expedite the migration process and enhance the performance of the migrated application.
Example: Using Spring Framework for Dependency Injection
// Utilizing the Spring Framework for dependency injection
@Component
public class EmployeeService {
private final EmployeeRepository employeeRepository;
@Autowired
public EmployeeService(EmployeeRepository employeeRepository) {
this.employeeRepository = employeeRepository;
}
// Additional methods and properties...
}
In the example above, we demonstrate the usage of the Spring Framework for dependency injection, a core aspect of modern Java development.
Closing Remarks
Migrating from ColdFusion to Java presents various challenges, ranging from transitioning code paradigms to adapting to new tools and frameworks. However, with a systematic approach and a clear understanding of the differences between the two platforms, organizations can successfully navigate these challenges and harness the capabilities of Java for their web development needs. By addressing database integration, object-oriented principles, template engine differences, security measures, and leveraging the Java ecosystem, the migration process can lead to a more robust and scalable web application. With careful planning and strategic implementation, the transition from ColdFusion to Java can pave the way for enhanced performance and future scalability.
In summary, migrating from ColdFusion to Java may be challenging, but it is a process that can yield long-term benefits for organizations seeking modern, high-performance web development solutions.
Remember, the key to a successful migration is a deep understanding of the existing codebase, strategic planning, and leveraging the robust features and frameworks that Java has to offer.
Start your Java migration journey today!
Checkout our other articles