Testing Spring Boot REST APIs with JUnit and Mockito
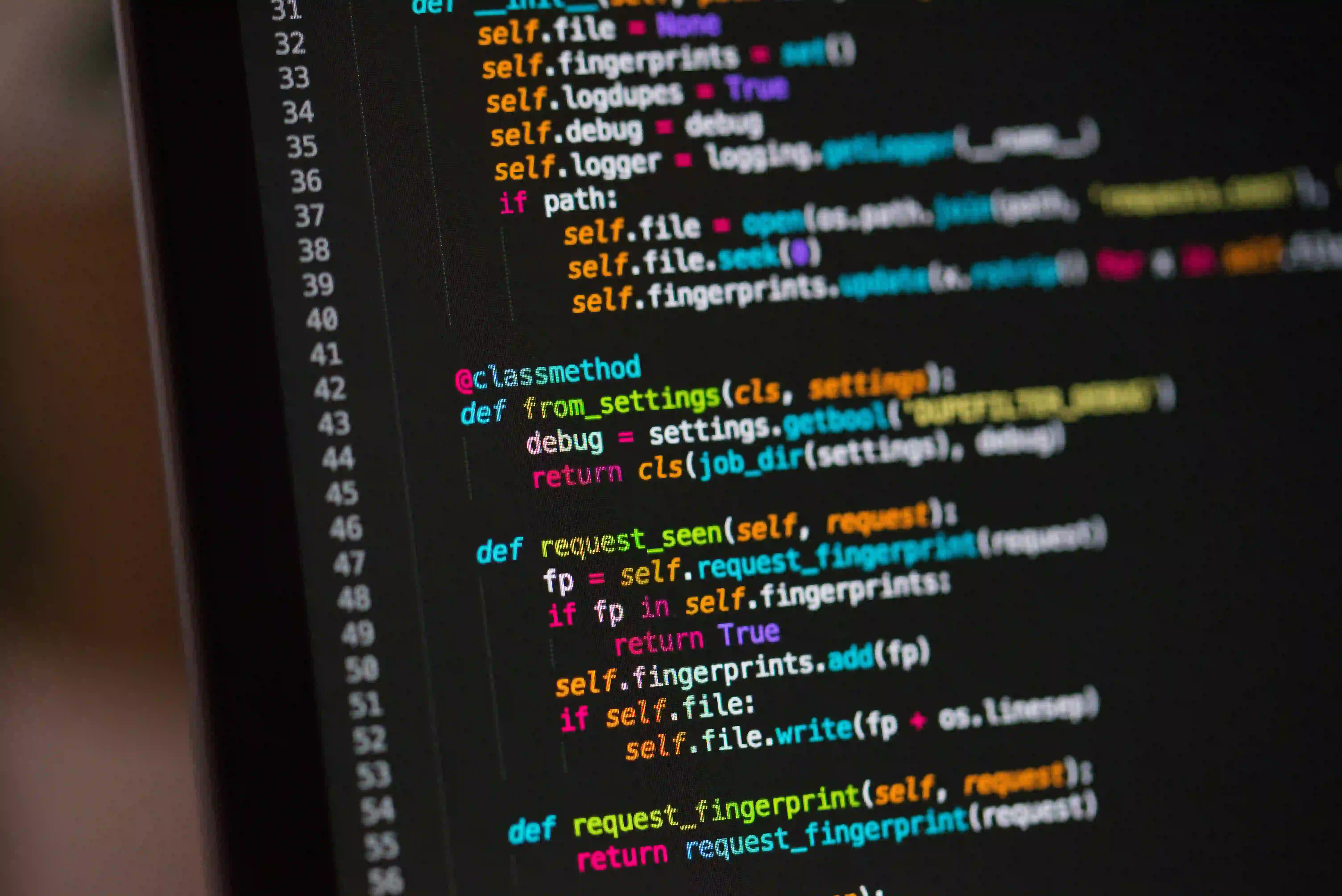
Testing Spring Boot REST APIs with JUnit and Mockito
Testing is a crucial aspect of software development as it ensures that the code behaves as expected. In a Spring Boot application, testing the REST APIs is particularly important as they form the interface through which the application communicates with the outside world. In this article, we'll explore how to test Spring Boot REST APIs using JUnit and Mockito.
Setting Up the Project
Before we dive into testing, let's set up a basic Spring Boot project. You can use Spring Initializr to generate a new project with the necessary dependencies. For this example, we'll include Spring Web
and Lombok
as dependencies.
Writing the Controller
Let's start by creating a simple REST controller that we can test. We'll create a UserController
with a single endpoint to retrieve user details.
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.getUserById(id);
return ResponseEntity.ok(user);
}
}
In this controller, we have a single endpoint GET /api/users/{id}
that retrieves user details based on the provided ID. The userService
dependency will be injected using Spring's dependency injection.
Writing the Service
Next, let's create a simple UserService
that the UserController
will rely on.
@Service
public class UserService {
public User getUserById(Long id) {
// Logic to retrieve user from database
}
}
For the sake of this example, we'll omit the actual logic to retrieve the user from the database and assume that it returns a User
object.
Writing the Test
Now, let's write a test for the UserController
using JUnit and Mockito. We'll use Mockito to mock the UserService
and isolate the controller for testing.
@RunWith(MockitoJUnitRunner.class)
public class UserControllerTest {
@InjectMocks
private UserController userController;
@Mock
private UserService userService;
@Test
public void testGetUserById() {
User mockUser = new User(1L, "John Doe");
Mockito.when(userService.getUserById(1L)).thenReturn(mockUser);
ResponseEntity<User> response = userController.getUserById(1L);
assertEquals(HttpStatus.OK, response.getStatusCode());
assertEquals("John Doe", Objects.requireNonNull(response.getBody()).getUserName());
}
}
In this test, we use @RunWith(MockitoJUnitRunner.class)
to enable Mockito annotations. The @InjectMocks
annotation is used to inject the mocked UserService
into the UserController
, and the @Mock
annotation creates a mock of the UserService
.
We then use Mockito.when
to define the behavior of the mocked UserService
when getUserById
is called with a specific ID. Finally, we invoke the getUserById
method of the userController
and assert the response using JUnit assertions.
Running the Test
To run the test, simply execute the test class as a JUnit test. If everything is set up correctly, the test should pass, indicating that the UserController
behaves as expected when invoking the GET /api/users/{id}
endpoint.
Bringing It All Together
In this article, we explored how to test Spring Boot REST APIs using JUnit and Mockito. Testing is an essential part of the software development lifecycle, and it ensures the reliability and correctness of the code. By following the principles of unit testing and using tools like JUnit and Mockito, we can create robust and maintainable code.
Now that you have a basic understanding of testing Spring Boot REST APIs, you can further explore advanced topics such as integration testing, security testing, and performance testing in the context of Spring Boot applications.
Remember, writing tests not only validates your code but also serves as living documentation for future developers working on the project. Happy testing!
Incorporate thorough testing in your software development process to ensure the robustness and reliability of your Spring Boot REST APIs.