5 Pain Points of Automating Development & How to Solve
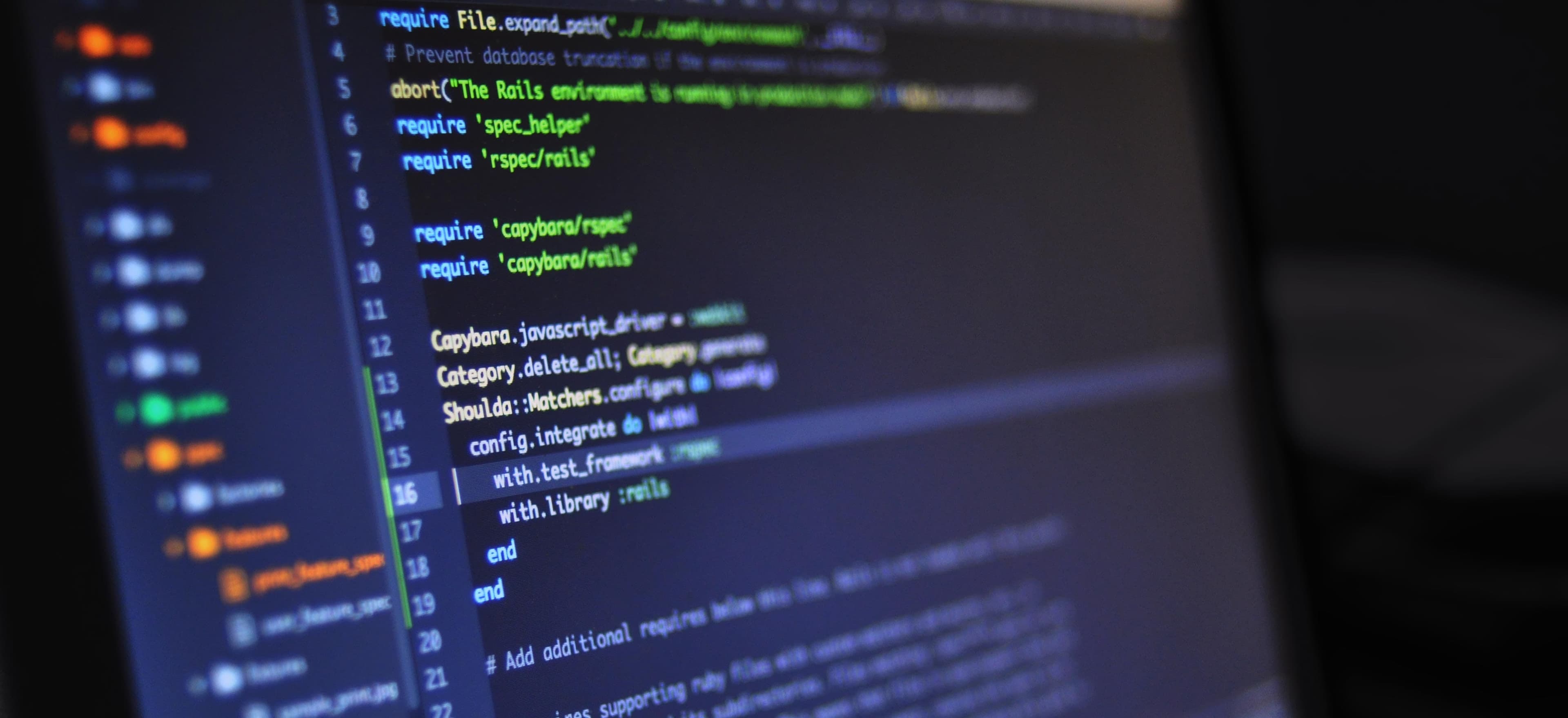
- Published on
5 Pain Points of Automating Development & How to Solve
Automating development processes is a crucial aspect of modern software development. It accelerates the delivery of high-quality software and reduces the likelihood of human error. However, as with any technical endeavor, automating development comes with its own set of challenges. In this blog post, we will explore five common pain points of automating development and discuss strategies for addressing them.
1. Complexity of Deployment
Automating the deployment process can be complex, especially in large-scale projects with multiple environments (development, testing, production). It requires careful coordination of configurations, dependencies, and networking settings.
To solve this pain point, consider using containerization technologies such as Docker. Docker allows you to package the application and its dependencies into a standardized unit for software development, which ensures that it will run consistently on any infrastructure. Additionally, tools like Kubernetes can help automate the deployment, scaling, and operations of application containers.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In the above Java code snippet, we have a simple "Hello, World!" program. Automating the deployment of this program using Docker and Kubernetes can significantly simplify the deployment process.
2. Integration Testing
Automating integration testing can be challenging due to the need to replicate real-world scenarios and interactions between various components of the software system. Setting up and maintaining an environment for integration testing can be time-consuming and error-prone.
One way to address this pain point is to use tools like Selenium for automating web application testing and RestAssured for automating REST API testing. Additionally, consider using mock servers to simulate external dependencies and automate the testing of individual components in isolation.
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 5);
assertEquals(8, result);
}
In the above JUnit test case, we are automating the integration testing of a Calculator
class using JUnit. This allows for automated validation of the add
method's functionality, enhancing the reliability of the software system.
3. Continuous Integration and Continuous Deployment (CI/CD)
Implementing a robust CI/CD pipeline is essential for automating the build, test, and deployment processes. However, managing the pipeline, ensuring its stability, and integrating it with version control systems can be daunting tasks.
To overcome this challenge, utilize CI/CD tools such as Jenkins, GitLab CI/CD, or CircleCI. These tools provide a platform for automating the entire software delivery process, from code commits to production deployment. Additionally, incorporate automated testing and code quality checks into the pipeline to catch issues early in the development cycle.
pipeline {
agent any
stages {
stage('Build') {
steps {
// Maven build
sh 'mvn clean package'
}
}
stage('Test') {
steps {
// Automated testing
sh 'mvn test'
}
}
stage('Deploy') {
steps {
// Deployment to staging/production
sh 'kubectl apply -f deployment.yaml'
}
}
}
}
In the above Jenkins Pipeline script, we have defined stages for build, test, and deployment, automating the entire CI/CD process for a Java application.
4. Infrastructure as Code (IaC)
Managing infrastructure for development, testing, and production environments manually can lead to inconsistencies and configuration drift. Infrastructure as Code (IaC) is the practice of managing and provisioning infrastructure through machine-readable definition files, rather than physical hardware configuration or interactive configuration tools.
To address the pain point of manual infrastructure management, adopt tools such as Terraform or AWS CloudFormation for defining and provisioning infrastructure in a declarative manner. By treating infrastructure as code, you can automate the creation, modification, and teardown of infrastructure, ensuring consistency across environments.
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
In the above Terraform configuration, we are defining an AWS EC2 instance as code, enabling automated provisioning and management of infrastructure resources.
5. Monitoring and Alerting
Automating development also entails automating the monitoring and alerting of software systems. Without proper monitoring and alerting in place, it becomes challenging to detect and respond to issues in a timely manner.
To alleviate this pain point, employ monitoring tools like Prometheus or Datadog for collecting and visualizing metrics from your applications and infrastructure. Utilize alerting mechanisms to notify the appropriate personnel when predefined thresholds are exceeded, ensuring proactive incident response and resolution.
public void performHealthCheck() {
// Check application health
if (!isApplicationHealthy()) {
// Send alert
AlertService.sendAlert("Application health check failed");
}
}
In the above Java method, we are automating the health check of an application and triggering an alert if the application's health status is compromised. Implementing such automated health checks and alerting mechanisms enhances the reliability and resilience of the software system.
In conclusion, automating development processes is essential for achieving greater efficiency and reliability in software delivery. However, it is crucial to address the pain points associated with automation to ensure successful implementation. By leveraging appropriate technologies and best practices, such as containerization, automated testing, CI/CD pipelines, Infrastructure as Code, and proactive monitoring, organizations can overcome these challenges and reap the benefits of streamlined and efficient development processes.
Checkout our other articles