Config Woes? Effortless Spring Data Solr Setup Guide
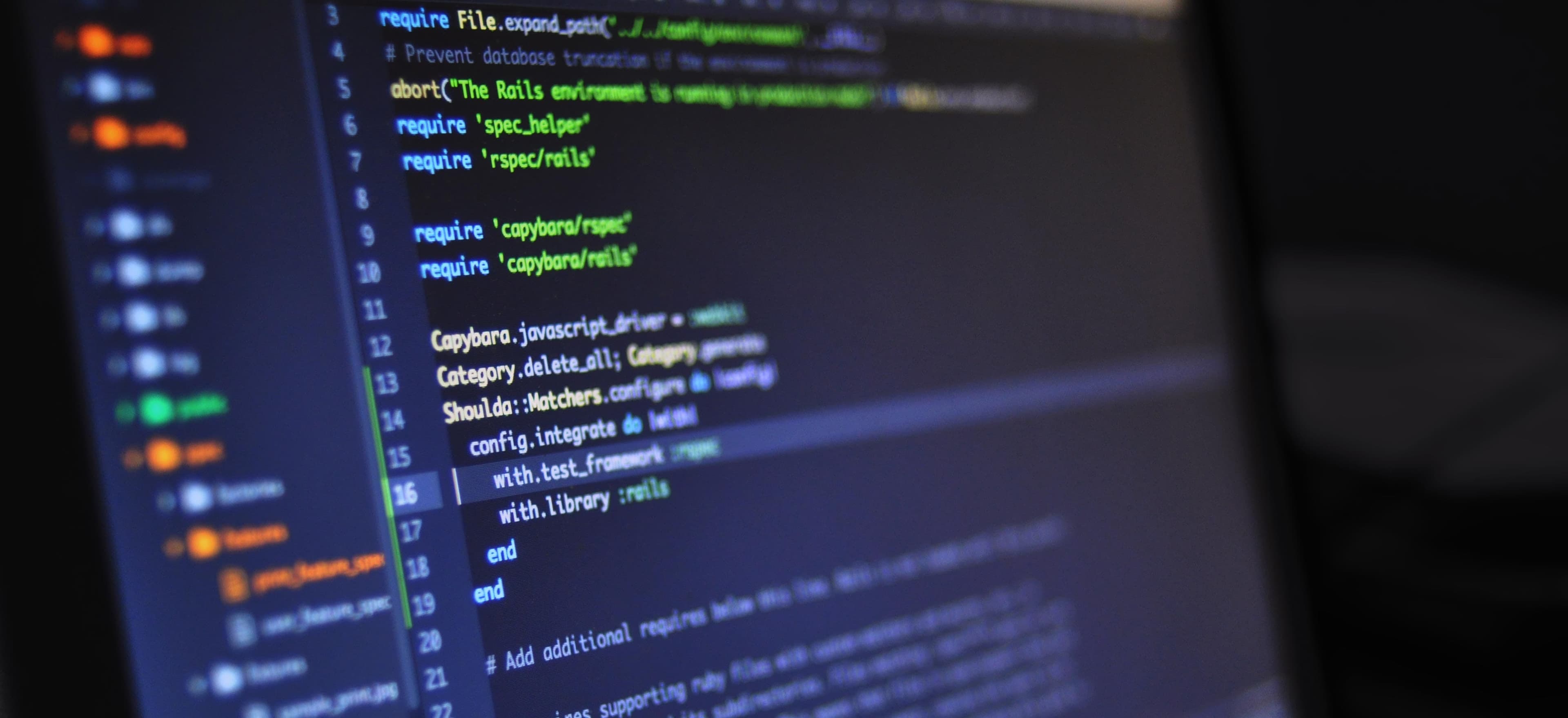
- Published on
Effortless Spring Data Solr Setup Guide: Solving Your Configuration Woes
When it comes to integrating powerful search functionality into Java applications, Apache Solr stands out as a robust, scalable tool. However, melding Solr with Spring Boot applications can sometimes feel like navigating a labyrinth. This guide aims to light the path, making the configuration of Spring Data Solr not just manageable but effortless. Whether you're an experienced developer or just starting out, this post will walk you through the crucial steps and best practices for a smooth Spring Data Solr setup, ensuring your projects leverage the full power of both technologies.
Why Spring Data Solr?
Spring Data Solr provides a familiar and consistent Spring-based programming model for data access while retaining Solr's powerful search capabilities. It abstracts the complexity of data storage, making database interactions seamless and efficient, which significantly speeds up the development process.
For more detailed insights into Spring Data Solr, the official Spring documentation is an excellent starting point: Spring Data Solr Reference Documentation.
Prerequisites
Before diving into the configuration, ensure you have the following:
- Java Development Kit (JDK): Version 8 or higher.
- Apache Solr Server: Installed and running. For installation guidance, refer to the official Solr guide.
- Spring Boot: Knowledge of basic Spring Boot concepts and project setup.
- Maven or Gradle: For project dependency management.
Step-by-Step Configuration
1. Starting Your Spring Boot Project
First, generate your Spring Boot project skeleton using the Spring Initializr. Select your preferred dependencies (Spring Data Solr should be your go-to for this guide, alongside any other dependencies your project might require).
2. Adding Dependencies
Include Spring Data Solr in your project by adding the following dependency in your pom.xml
for Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-solr</artifactId>
</dependency>
For Gradle, add this line to your build.gradle
:
implementation 'org.springframework.boot:spring-boot-starter-data-solr'
3. Configuring Solr in application.properties
Spring Boot's auto-configuration takes care of most of the setup, but you still need to configure your Solr endpoint in the application.properties
or application.yml
file:
spring.data.solr.host=http://localhost:8983/solr/mycollection
Replace mycollection
with the name of your specific Solr collection.
4. Defining a Solr Document
To communicate with Solr, we define an entity class, annotated with @SolrDocument
:
import org.apache.solr.client.solrj.beans.Field;
import org.springframework.data.annotation.Id;
import org.springframework.data.solr.core.mapping.SolrDocument;
@SolrDocument(collection = "mycollection")
public class Product {
@Id
@Field
private String id;
@Field
private String name;
@Field
private float price;
// Constructors, getters, and setters
}
The @SolrDocument
annotation maps this class to a Solr collection, while @Field
annotations map class fields to Solr document fields.
5. Creating a Repository
Spring Data Solr uses repositories to abstract the data layer. Here's how you can define a simple repository for the Product
document:
import org.springframework.data.solr.repository.SolrCrudRepository;
public interface ProductRepository extends SolrCrudRepository<Product, String> {
// Define custom query methods here
}
Spring Data automatically implements this repository, providing CRUD operations on Product
entities.
6. Writing a Simple Test
Before you proceed, it's crucial to ensure everything is wired correctly. Write a simple test to save and fetch a Product
:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class SolrIntegrationTest {
@Autowired
private ProductRepository repository;
@Test
public void whenSavingProduct_thenAvailableOnRetrieval() {
final Product product = new Product("1", "Spring Data Solr Guide", 29.99f);
repository.save(product);
final Product retrievedProduct = repository.findById("1").get();
assert(retrievedProduct.getName().equals(product.getName()));
}
}
This test saves a Product
document and then retrieves it, validating the setup.
Troubleshooting Common Issues
While configuring Spring Data Solr, developers often encounter hurdles. Here's how to tackle some of the most common ones:
- Connection Refused: Ensure your Solr server is running and accessible at the configured URL.
- Mapping Errors: Verify that your entity class accurately mirrors the Solr document structure.
- Dependency Conflicts: Make sure you're using compatible versions of Spring Boot and Spring Data Solr. Consult the Spring Initializr for guidance on compatible versions.
The Bottom Line
Integrating Spring Data Solr into your Spring Boot application might initially seem daunting, but with the right steps, it's a straightforward process. By following this guide, you've learned how to configure Spring Data Solr, from adding dependencies to writing your first repository and integration test. The amalgamation of Spring Boot's simplicity and Solr's powerful search capabilities offers a potent toolset for developing sophisticated, search-enabled applications.
For continued learning and troubleshooting, the broader Spring and Solr communities offer a wealth of resources and forums. Your journey with Spring Data Solr is just beginning, and there's much more to explore and master.
Happy coding, and may your search functionality be as seamless and powerful as the tools you're wielding!