Overcoming Data Consistency in Microservices: A Guide
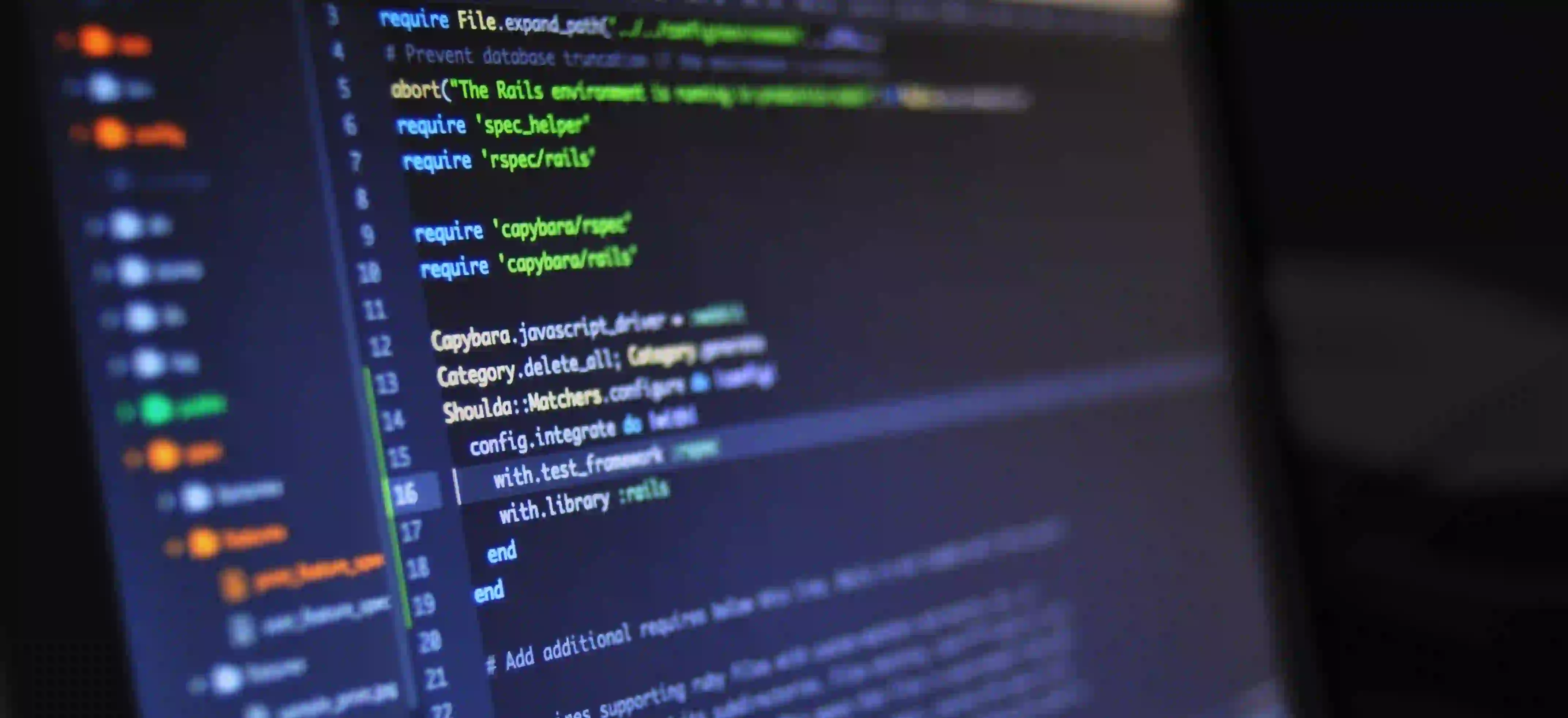
Overcoming Data Consistency in Microservices: A Guide
Microservices architecture has gained popularity due to its ability to break down large, monolithic applications into smaller, independently deployable services. Each microservice manages its own data, leading to challenges in maintaining data consistency across the system. In this article, we'll explore the importance of data consistency in microservices and discuss strategies and tools to overcome this challenge.
The Importance of Data Consistency in Microservices
Data consistency is crucial in any application, but it becomes more challenging in a microservices environment. As each microservice has its own database or data store, ensuring that data remains consistent across services can be complex. Inconsistencies can lead to a range of issues, including inaccurate reporting, incorrect business decisions, and ultimately, a poor user experience.
Challenges of Maintaining Data Consistency in Microservices
1. Distributed Transactions
- In a microservices architecture, a single business operation often spans multiple microservices, requiring coordination and consistency across these services. Traditional ACID transactions, which work within a single database, are not suitable for distributed transactions.
- Sample code:
☕snippet.java
@Transactional public void placeOrder(Order order) { // Code to place the order }
- Why: In the above code, the
@Transactional
annotation ensures that theplaceOrder
method runs within a transaction, maintaining data consistency within a single database.
2. Eventual Consistency
- Microservices often opt for eventual consistency, allowing data changes to propagate asynchronously across services. While this approach offers scalability and performance benefits, it introduces the challenge of dealing with temporarily inconsistent data.
- Sample code:
☕snippet.java
@PostMapping("/update") public void updateData(@RequestBody Data data) { // Code to update data in the database }
- Why: The
@PostMapping
annotation in the code snippet represents a common REST API endpoint used to update data in a microservice, potentially leading to eventual consistency challenges.
3. Polyglot Persistence
- Using different databases or data storage technologies for individual microservices (polyglot persistence) adds complexity to maintaining data consistency. Each database may have its own consistency model and transaction support, leading to varied approaches for ensuring consistency.
- Sample code:
☕snippet.java
public void saveData(Data data) { // Code to save data in a NoSQL database }
- Why: The above code snippet demonstrates saving data in a NoSQL database, which may have a different consistency model compared to traditional relational databases.
Strategies to Overcome Data Consistency Challenges
1. Design for Autonomy
- Each microservice should aim for autonomy in managing its data. This includes defining clear boundaries and interfaces for data access, ensuring that each service is responsible for its own data consistency.
- When designing microservices, consider the principles of Domain-Driven Design to establish clear boundaries and responsibilities for each service.
2. Saga Pattern
- The Saga pattern provides a way to manage distributed transactions in a microservices architecture. It breaks a transaction into a series of smaller, more manageable steps, each with its own compensating transactions to ensure eventual consistency.
- Sample code:
☕snippet.java
public void createOrderSaga(Order order) { // Step 1: Reserve items // Step 2: Charge payment // ... }
- Why: The Saga pattern allows breaking down a complex transaction, such as creating an order, into smaller steps that can be individually managed for consistency.
3. Event Sourcing and CQRS
- Implementing Event Sourcing and Command Query Responsibility Segregation (CQRS) can help address data consistency challenges. Event sourcing captures all changes to the application state as a sequence of events, while CQRS separates the command (write) and query (read) responsibilities.
- Sample code:
☕snippet.java
@EventSourcingHandler public void onOrderCreated(OrderCreatedEvent event) { // Code to update the order read model }
- Why: Event sourcing and CQRS enable capturing and processing events to maintain data consistency, and separating read and write operations for improved scalability.
Tools for Managing Data Consistency in Microservices
1. Apache Kafka
- Apache Kafka is a distributed event streaming platform that can be used to build real-time data pipelines and streaming applications. It facilitates the communication and synchronization of data across microservices through its distributed, fault-tolerant architecture.
- Sample configuration:
📄snippet.txt
bootstrap.servers=kafka-broker1:9092,kafka-broker2:9092 group.id=data-consistency-group
2. Jaeger
- Jaeger is an open-source, end-to-end distributed tracing system, which can be instrumental in understanding and troubleshooting the flow of data and transactions across microservices. It provides insights into the latency and flow of data between services.
- Sample instrumentation:
☕snippet.java
Tracer tracer = new Configuration("service-name").getTracer();
3. Redis
- Redis is an in-memory data structure store that can act as a caching layer to improve the performance and consistency of data access across microservices. It supports various data structures and can be used for implementing distributed locks and queues.
- Sample usage:
☕snippet.java
redisClient.set("key", "value");
To Wrap Things Up
Maintaining data consistency in a microservices architecture is a complex but critical aspect of building reliable and scalable systems. By embracing design patterns, such as Saga and Event Sourcing, and leveraging tools like Apache Kafka and Jaeger, developers can address the challenges of data consistency while reaping the benefits of a microservices architecture. Remember, each microservice should prioritize autonomy while ensuring eventual consistency across the system.
In this guide, we have delved into the intricacies of managing data consistency in a microservices architecture, from the challenges posed by distributed transactions and eventual consistency to the strategies and tools available for overcoming these hurdles. By considering the importance of data consistency, implementing appropriate design patterns, and utilizing effective tools, developers can navigate the complexities of maintaining data consistency in a microservices environment.