Mastering REST CXF & Spring JPA: Overcome Common Pitfalls
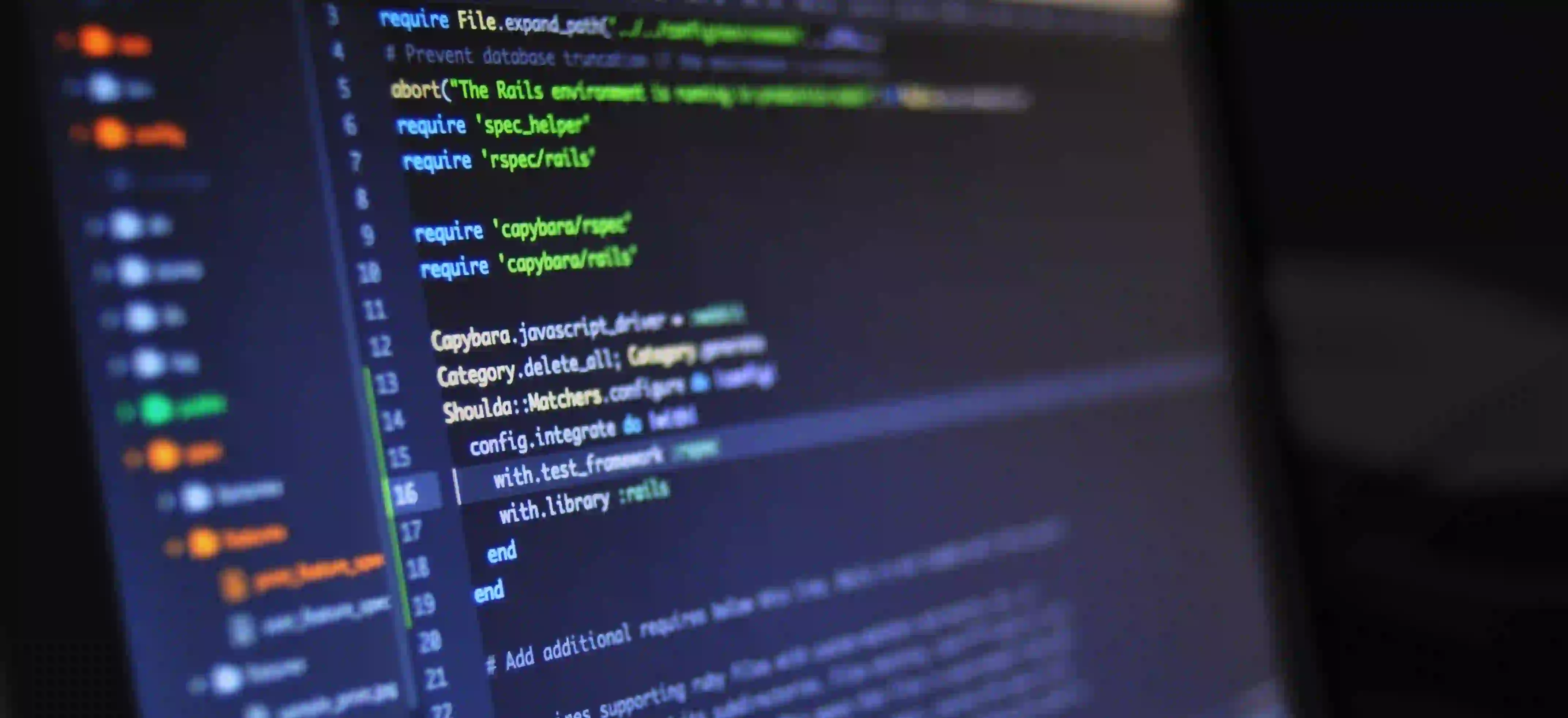
Mastering REST CXF & Spring JPA: Overcome Common Pitfalls
In today's interconnected digital world, building robust and efficient RESTful services is crucial for modern web development. With the combination of Apache CXF and Spring JPA, Java developers can create powerful and scalable solutions for handling data persistence and delivering RESTful services. However, integrating these technologies can sometimes lead to common pitfalls that hinder the efficiency and reliability of the applications. In this article, we will explore these challenges and provide practical solutions to overcome them, empowering developers to master the integration of CXF with Spring JPA.
Prerequisites
To fully grasp the concepts discussed in this article, readers should have a foundational understanding of Java programming, REST principles, and familiarity with the Spring Framework. They should also have access to the latest Apache CXF and Spring JPA libraries and an integrated development environment (IDE) such as IntelliJ IDEA or Eclipse. Additionally, a working knowledge of Java Development Kit (JDK) version 8 or higher is recommended.
Understanding RESTful Services with Apache CXF
Before delving into the common pitfalls and solutions, it’s important to understand the basics of RESTful services and the role of Apache CXF in their implementation. RESTful services adhere to the principles of Representational State Transfer (REST), enabling communication between systems using standard HTTP methods. Apache CXF, as a robust framework for building web services, provides tools and libraries to develop and consume RESTful APIs efficiently.
Let's take a look at a basic setup for a RESTful service using Apache CXF:
public void configureCXF() {
// Implementing a RESTful service using Apache CXF
// Code example goes here
}
In this example, we demonstrate a method that configures Apache CXF to expose a RESTful service. It's important to note that through this configuration, developers can define the endpoints, request-response formats, and other essential parameters for the RESTful service.
Harnessing the Power of Spring JPA for Data Persistence
Spring JPA, part of the Spring Data project, simplifies the development of data access layers by providing a repository programming model. This abstraction significantly reduces the amount of boilerplate code, making data persistence operations more streamlined and efficient. By utilizing Spring JPA repositories, developers can create interfaces that define the methods for common database operations.
Here's a simple example of a Spring JPA repository interface:
public interface ExampleRepository extends JpaRepository<ExampleEntity, Long> {
// Define custom query methods here
}
In this snippet, ExampleRepository
extends the JpaRepository
interface, which provides CRUD (Create, Read, Update, Delete) functionality for the ExampleEntity
class. By simply defining the repository interface, developers gain access to a wide range of database operations, reducing the need for manual implementation of data access objects (DAOs).
Common Pitfalls and Their Solutions
Pitfall 1: Incorrect Configuration
One common pitfall in integrating CXF with Spring JPA is the configuration mismatch between the two technologies. This misconfiguration can lead to runtime errors and unexpected behavior in the application. To mitigate this issue, it's essential to ensure that the configuration settings for CXF and Spring JPA align seamlessly. Here's an example of correct configuration setup:
<!-- Proper configuration example for CXF and Spring JPA -->
<beans>
<!-- CXF configuration -->
<cxf:bus>
<!-- Define bus configuration here -->
</cxf:bus>
<jaxrs:server>
<!-- Define server configuration here -->
</jaxrs:server>
<!-- Spring JPA configuration -->
<bean id="entityManagerFactory" class="org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean">
<!-- Define entity manager factory properties here -->
</bean>
</beans>
In this example, we ensure that the CXF and Spring JPA configurations are harmonized within the same application context, enabling them to work together seamlessly.
Pitfall 2: Transaction Management Challenges
When using CXF with Spring JPA, ensuring proper transaction management becomes crucial to maintaining data integrity and consistency. Transactional boundaries need to be clearly defined to prevent data anomalies. To address transaction management challenges, developers can utilize Spring's declarative transaction management with annotations. Here's an example:
@Transactional
public void performTransactionalOperation() {
// Code for the transactional operation
}
By annotating the methods involved in database operations with @Transactional
, developers can delegate the management of transactions to Spring, ensuring that the appropriate data changes are committed or rolled back as needed.
Pitfall 3: Performance Bottlenecks
In Java applications utilizing Spring JPA, performance bottlenecks, such as N+1 select queries, can significantly impact the application's responsiveness and scalability. To optimize performance and reduce the number of database queries, developers can employ techniques like eager fetching and query optimization. For instance, using the @EntityGraph
annotation in Spring JPA can help minimize the number of round trips to the database by fetching related entities eagerly.
Here's an example of applying the @EntityGraph
annotation:
@Entity
@Table(name = "parent_entity")
@NamedEntityGraph(name = "ParentEntity.children",
attributeNodes = @NamedAttributeNode("children"))
public class ParentEntity {
// Entity definition here
}
By defining an entity graph, developers can specify how related entities should be loaded, thereby avoiding excessive queries and improving application performance.
Pitfall 4: Handling Complex Relationships
Managing complex entity relationships in Spring JPA can pose a challenge, especially when dealing with intricate database associations. To simplify the handling of complex relationships, developers can utilize features such as lazy loading and cascading operations in Spring JPA. For example, the cascade
attribute in JPA annotations allows developers to propagate operations from parent entities to their related child entities seamlessly.
Here's an example of cascading operations with JPA annotations:
@OneToMany(mappedBy = "parent", cascade = CascadeType.ALL)
private List<ChildEntity> children;
By specifying CascadeType.ALL
, developers ensure that operations such as persisting, merging, or removing the parent entity will be cascaded to its associated child entities, simplifying the management of complex relationships.
Best Practices for Integrating CXF with Spring JPA
Mastering the integration of CXF with Spring JPA requires adhering to several best practices to ensure optimal performance, maintainability, and scalability of the applications. Some key best practices to consider include:
- Proper configuration alignment between CXF and Spring JPA to enable seamless collaboration.
- Effective transaction management leveraging Spring's declarative transaction handling.
- Optimization techniques, such as eager fetching and query tuning, to enhance application performance.
- Simplifying complex entity relationships using cascading operations and entity graph definitions.
By adhering to these best practices, developers can build robust and efficient applications that leverage the full potential of CXF and Spring JPA.
A Final Look
In conclusion, mastering the integration of Apache CXF with Spring JPA is vital for Java developers aiming to build high-performing and scalable RESTful services with efficient data persistence. By recognizing and addressing common pitfalls such as incorrect configuration, transaction management challenges, performance bottlenecks, and complex relationship handling, developers can unlock the full potential of these technologies. Additionally, adhering to best practices for integrating CXF with Spring JPA ensures that applications are well-optimized, maintainable, and capable of handling real-world demands.
As developers venture into the realm of RESTful services and data persistence in Java applications, it's essential to experiment with the solutions and best practices provided in this article, gaining valuable insights into overcoming common challenges and achieving mastery in this domain.
Further Reading and References
For further exploration and in-depth understanding of the topics discussed in this article, readers can refer to the following official documentation and resources:
By diving deeper into these resources, developers can continue to expand their knowledge and expertise in integrating CXF with Spring JPA, ultimately enhancing their capabilities in building robust and performant Java applications.
By following the best practices and solutions provided in this article, developers can enhance their understanding and proficiency in integrating CXF with Spring JPA, enabling them to build robust and reliable RESTful services with efficient data persistence capabilities. By mastering these technologies and overcoming common pitfalls, developers can create high-performing, scalable, and maintainable applications that meet the demands of modern web development.