Winning with Hibernate: Why Choose Bidirectional Sets Over Lists?
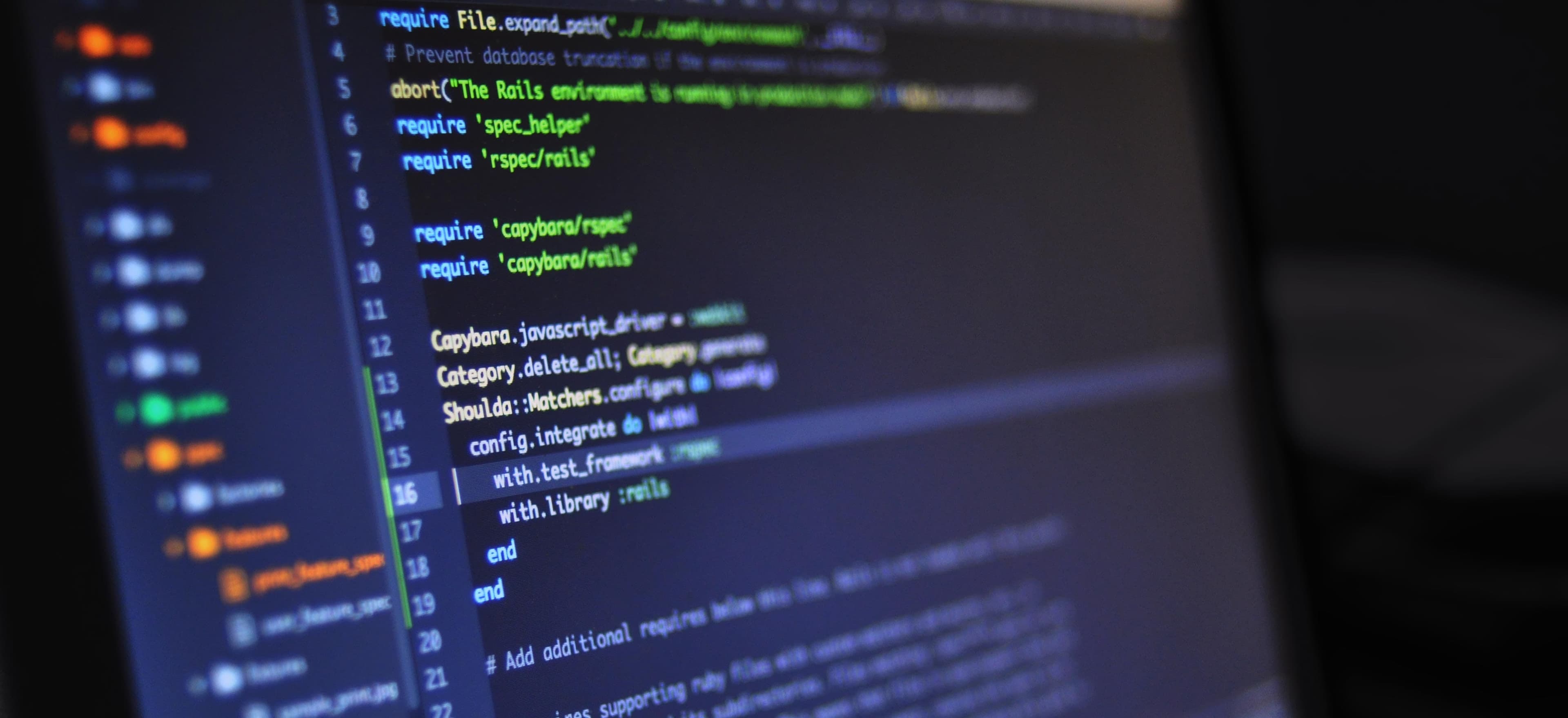
- Published on
Winning with Hibernate: Why Choose Bidirectional Sets Over Lists?
In the world of Java programming and database management, Hibernate has become a frontrunner for developers. Its ability to map Java classes to database tables, and Java data types to SQL data types, offers a seamless persistence mechanism to store and retrieve objects from a database. One of the critical aspects when dealing with Hibernate is managing relationships between entities. In this blog post, we will discuss one such relationship aspect: the choice between bidirectional sets and lists, and why bidirectional sets might be the winning choice for your Hibernate-based application.
Understanding the Basics
Before we dive into the debate of sets vs. lists, let's take a moment to understand the concept of bidirectional relationships in Hibernate. In a bidirectional relationship, two entities are connected in such a way that they are aware of each other. This awareness allows for efficient navigation in both directions, which is crucial when dealing with associations between entities.
For instance, consider a scenario of an employee and a department. An employee belongs to a department, and a department has multiple employees. This creates a bidirectional association where an employee is aware of their department, and a department is aware of its employees.
The Battle: Sets vs. Lists
When it comes to representing the collections of associated entities in a bidirectional relationship, Hibernate offers two main options: sets and lists. Both sets and lists have their own advantages and use cases, but in many scenarios, using sets can offer better performance and maintainability compared to lists.
Performance Matters
In a bidirectional one-to-many relationship, using a set instead of a list can provide better performance. This performance gain stems from the fact that a set does not allow duplicate entries, leading to potentially faster retrieval and storage operations compared to a list, which maintains the order of elements and permits duplicates.
In situations where the order of elements is not critical, leveraging sets can result in optimizations during database operations. Additionally, when working with large datasets, the performance benefits of sets become even more noticeable, making it a favorable choice for scaling applications.
Avoiding Duplicates
By nature, a set ensures uniqueness among its elements. When dealing with bidirectional relationships, this characteristic can prevent the inadvertent addition of duplicate entities. In scenarios where data integrity is a priority, using sets can serve as a safeguard against unintentional data redundancy, consequently enhancing the reliability of the application's data layer.
Simplified Code Maintenance
One of the often-overlooked advantages of using sets in bidirectional relationships is the simplification of code maintenance. When entities are associated bidirectionally and collections are managed using sets, adding and removing elements becomes more straightforward. The unique constraint imposed by sets ensures that developers can focus on the logic of the operations without having to code additional checks for duplicate entries, thereby streamlining the codebase and reducing the potential for errors.
Code Comparison: Sets vs. Lists
Let's examine a practical code example to illustrate the differences between using sets and lists in a bidirectional relationship within Hibernate.
Using a Set
public class Department {
@OneToMany(mappedBy = "department")
private Set<Employee> employees = new HashSet<>();
// other attributes and methods
}
In this example, the Department entity uses a set to represent the employees associated with it. By employing a set, the Department entity ensures that each employee is unique, thus avoiding duplicate entries.
Using a List
public class Department {
@OneToMany(mappedBy = "department")
private List<Employee> employees = new ArrayList<>();
// other attributes and methods
}
On the other hand, the use of a list in the Department entity to manage the associated employees allows for the maintenance of the order of elements. However, it does not guarantee uniqueness, potentially leading to the inclusion of duplicate employee entries.
The Last Word
While the choice between sets and lists ultimately depends on the specific requirements of an application, leveraging bidirectional sets in Hibernate can offer notable benefits in terms of performance, data integrity, and code maintenance. By understanding the nuances and implications of using sets and lists in a bidirectional relationship, developers can make informed decisions to optimize the efficiency and robustness of their Hibernate-based applications.
In conclusion, when considering bidirectional relationships in Hibernate, choosing sets over lists can pave the way for enhanced performance, data integrity, and simplified code maintenance, ultimately contributing to the overall success of the application.
Understanding the intricacies of Hibernate and its relationship management is pivotal for developing efficient and scalable applications. By grasping the significance of bidirectional associations and making informed choices when handling collections, developers can ensure the seamless integration of Hibernate within their projects.
So, the next time you find yourself at the crossroads of deciding between sets and lists for managing bidirectional relationships in Hibernate, consider the potential advantages that sets bring to the table, and pave the way for optimized, reliable, and maintainable data interactions within your application.
For further understanding, refer to the official documentation of Hibernate for in-depth insights on entity associations and the use of collections.