Overcoming Delayed Messages in RabbitMQ: A Guide
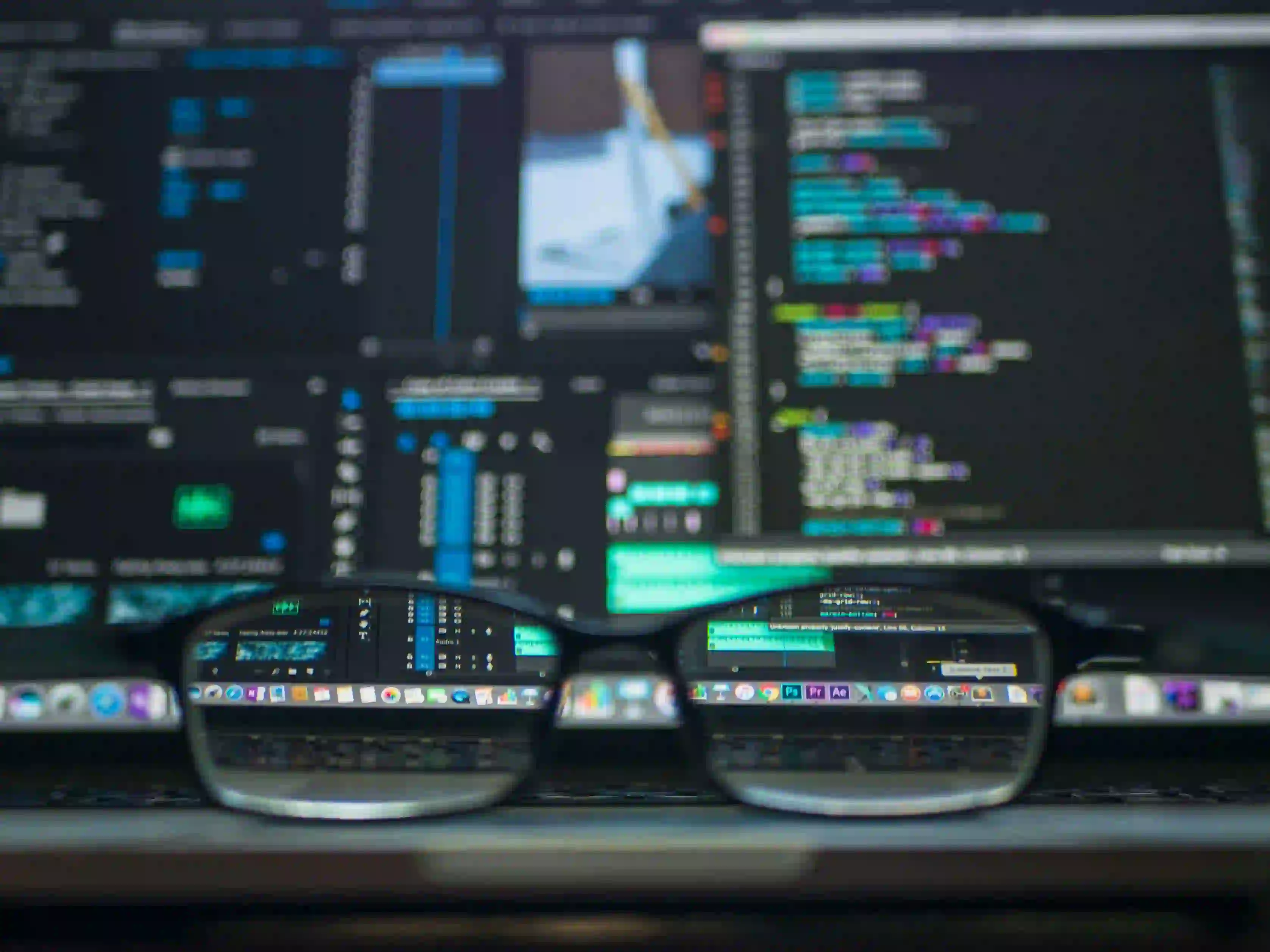
Overcoming Delayed Messages in RabbitMQ: A Guide
For any software application, ensuring prompt message delivery is crucial for maintaining optimal performance and user satisfaction. RabbitMQ, a leading open-source message broker, excels at processing vast amounts of messages. However, developers often encounter the challenge of delayed messages, which can hinder application responsiveness and efficiency. This comprehensive guide delves into the reasons behind message delays in RabbitMQ and presents practical solutions, complete with Java code examples, to efficiently manage and mitigate these issues.
Understanding the Cause of Delayed Messages in RabbitMQ
Before diving into solutions, it's essential to grasp why messages get delayed in RabbitMQ. Several factors contribute to this problem, including:
- Queue Length: A long queue can significantly delay the processing of messages.
- Resource Constraints: Limited CPU, memory, or network bandwidth can slow down message delivery.
- Consumer Performance: Slow message processing by consumers can cause a backlog of messages.
- Configuration Missteps: Incorrectly configured exchanges, queues, or bindings can lead to inefficiencies.
Recognizing these factors is the first step towards addressing delayed messages.
Strategies to Overcome Delayed Messages
Implementing effective strategies can significantly enhance message processing times. Here are some practical approaches:
1. Prioritize Messages
Using priority queues enables critical messages to be processed faster. RabbitMQ supports priority queues, allowing developers to set message priorities on a scale:
import com.rabbitmq.client.AMQP;
import com.rabbitmq.client.ConnectionFactory;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.Channel;
public class PriorityProducerExample {
private static final String QUEUE_NAME = "priority_queue";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
// Declare a priority queue
channel.queueDeclare(QUEUE_NAME, true, false, false, Map.of("x-max-priority", 10));
String criticalMessage = "Critical task";
String normalMessage = "Normal task";
// Publish messages with different priorities
AMQP.BasicProperties priorityProps = new AMQP.BasicProperties.Builder()
.priority(9) // for critical messages
.build();
channel.basicPublish("", QUEUE_NAME, priorityProps, criticalMessage.getBytes());
System.out.println(" [x] Sent '" + criticalMessage + "' with high priority");
channel.basicPublish("", QUEUE_NAME, null, normalMessage.getBytes());
System.out.println(" [x] Sent '" + normalMessage + "' with default priority");
}
}
}
In the example above, we declare a priority queue and send messages with different priorities. This approach helps in ensuring that critical tasks are attended to promptly, thus reducing delays.
2. Optimize Consumer Performance
Improving consumer performance is vital for processing messages efficiently. You can distribute the workload among multiple consumers or use acknowledgment batches to decrease processing time:
import com.rabbitmq.client.*;
public class Worker {
private static final String TASK_QUEUE_NAME = "task_queue";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
final Connection connection = factory.newConnection();
final Channel channel = connection.createChannel();
channel.queueDeclare(TASK_QUEUE_NAME, true, false, false, null);
System.out.println(" [*] Waiting for messages. To exit press CTRL+C");
DeliverCallback deliverCallback = (consumerTag, delivery) -> {
String message = new String(delivery.getBody(), "UTF-8");
System.out.println(" [x] Received '" + message + "'");
try {
doWork(message);
} finally {
System.out.println(" [x] Done");
channel.basicAck(delivery.getEnvelope().getDeliveryTag(), false);
}
};
boolean autoAck = false; // Enable explicit acknowledgments
channel.basicConsume(TASK_QUEUE_NAME, autoAck, deliverCallback, consumerTag -> { });
}
private static void doWork(String task) {
// Simulate work
}
}
This snippet demonstrates how a worker consumes messages from a queue and acknowledges them once processed, thus clearing the queue faster.
3. Monitor and Scale
Constant monitoring can provide insights into bottleneck areas, enabling timely adjustments. Using RabbitMQ's management plugin, you can monitor queue lengths, message rates, and more. Scale horizontally by adding more consumers or vertically by upgrading hardware resources based on your observations.
Additional Tips for Managing Delayed Messages
While the strategies above are effective, here are additional tips to consider:
- Message TTL (Time-To-Live): Setting a TTL on messages ensures they don't clog the queue for too long. Learn more about message TTL.
- Dead Letter Exchanges: Use dead letter exchanges to redirect messages that can't be processed. Explore dead letter exchanges.
- Efficient Routing: Design efficient routing strategies to ensure messages are sent only to relevant queues.
- RabbitMQ Clustering: Implementing a RabbitMQ cluster can enhance scalability and reliability.
Closing the Chapter
Delays in message processing can be a stumbling block for applications leveraging RabbitMQ. By understanding the root causes and implementing the strategies outlined in this guide, developers can ensure smooth, efficient message delivery. Remember, the key to managing RabbitMQ effectively lies in constant monitoring, optimization, and scalability.
With these strategies and best practices, you can overcome the challenge of delayed messages in RabbitMQ, ensuring your applications remain responsive and performant. Happy coding!
I hope this guide serves as a valuable resource for developers looking to optimize their use of RabbitMQ with Java. Embracing these practices will not only address delayed messages but also enhance the overall robustness and efficiency of your messaging system.