Unlocking Scalability: Mastering Cloud-Native Patterns
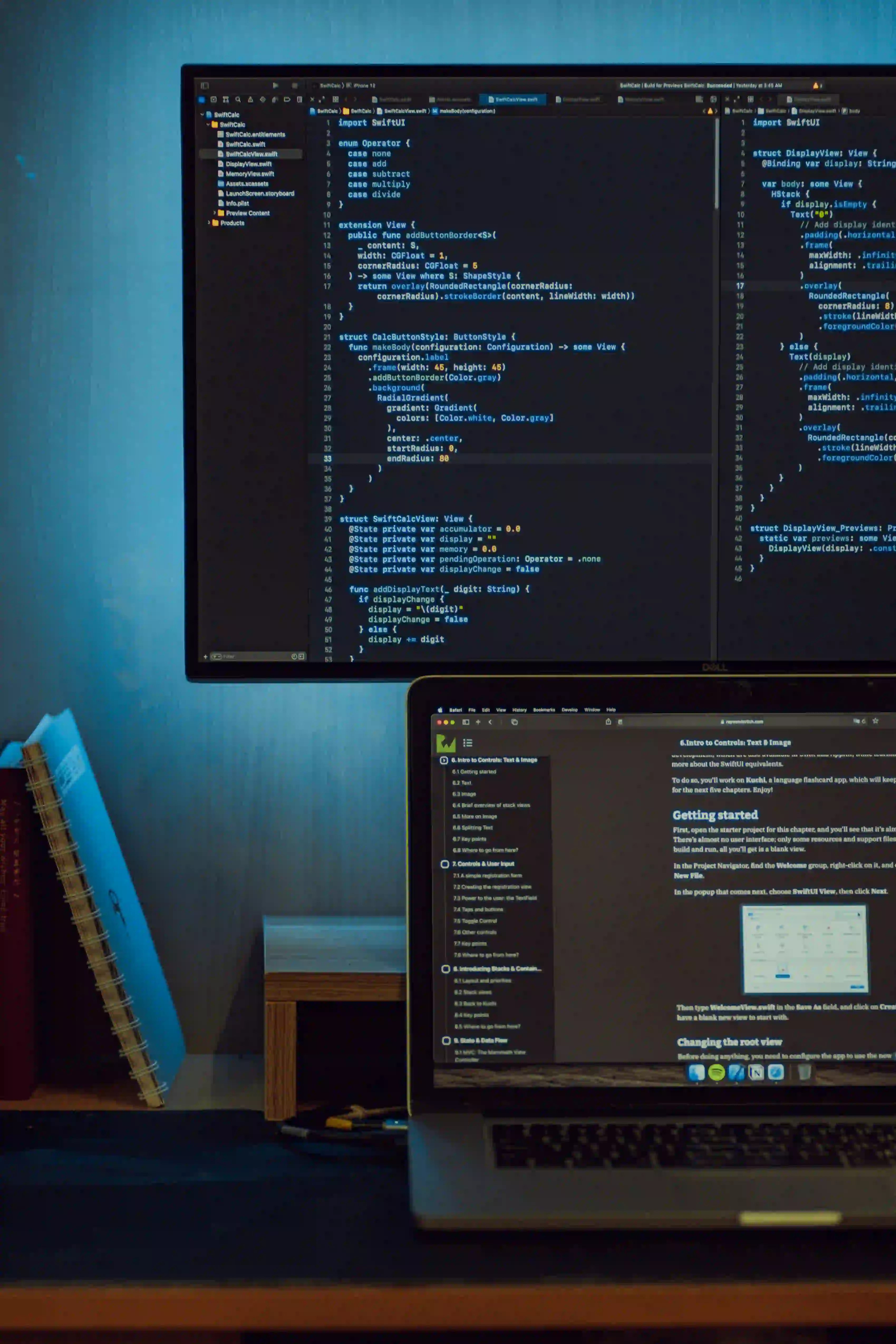
Unlocking Scalability with Cloud-Native Java Applications
In the world of software development, the need for scalable applications has become increasingly important. As user bases grow and traffic spikes occur, the ability to seamlessly handle increased demand is crucial. This is where cloud-native applications shine. By leveraging the power of cloud computing and adopting a microservices architecture, Java developers can build highly scalable applications that are resilient, flexible, and efficient.
In this blog post, we'll explore the concept of scalability in the context of cloud-native Java applications. We'll delve into essential patterns and best practices for creating scalable, resilient, and high-performing applications. We'll also examine how to harness the capabilities of cloud platforms to achieve unparalleled scalability.
Understanding Scalability in Cloud-Native Applications
Scalability is the ability of a system to handle growing amounts of work by adding resources to the system. In the context of cloud-native applications, scalability is achieved through horizontal scaling, which involves adding more instances of a service to distribute the load.
Java, with its robust ecosystem and mature tooling, is an excellent choice for building cloud-native applications. Leveraging frameworks like Spring Boot and Quarkus, developers can create microservices that can be independently deployed and scaled. Additionally, Java's support for asynchronous programming and message-driven architecture lends itself well to building scalable and responsive systems.
Embracing Microservices for Scalability
Microservices architecture has gained widespread adoption in building scalable applications. By breaking down monolithic applications into smaller, independently deployable services, developers can effectively distribute the load and scale individual components based on demand.
Let's consider a simple example of a microservice that handles user authentication. Instead of having authentication logic tightly coupled with other functionalities, such as user management and data processing, the authentication functionality can be extracted into its own microservice. This decoupling allows the authentication service to be scaled independently based on the incoming workload.
// Example of a simple authentication microservice in Java using Spring Boot
@RestController
public class AuthenticationController {
@Autowired
private AuthenticationService authenticationService;
@PostMapping("/login")
public ResponseEntity<String> login(@RequestBody LoginRequest request) {
// Validate credentials and generate JWT token
String token = authenticationService.authenticate(request.getUsername(), request.getPassword);
return ResponseEntity.ok(token);
}
// Other authentication endpoints
}
In this snippet, we see a basic Spring Boot controller for handling user authentication. By encapsulating this functionality into a microservice, it becomes easier to scale and maintain.
Leveraging Asynchronous Communication
Scalable applications often rely on asynchronous communication patterns to ensure responsiveness and efficient resource utilization. In cloud-native environments, message brokers like Apache Kafka and RabbitMQ play a crucial role in enabling asynchronous communication between microservices.
Using Java's support for asynchronous programming, developers can seamlessly integrate with message brokers to build highly scalable and responsive systems. By offloading time-consuming tasks to asynchronous queues, the overall throughput of the system can be greatly improved.
// Example of producing messages to an Apache Kafka topic in Java
public class KafkaProducer {
private final KafkaTemplate<String, String> kafkaTemplate;
public KafkaProducer(KafkaTemplate<String, String> kafkaTemplate) {
this.kafkaTemplate = kafkaTemplate;
}
public void sendMessage(String topic, String message) {
kafkaTemplate.send(topic, message);
}
// Other producer methods
}
In this example, we demonstrate a simple Kafka producer that publishes messages to a topic. By leveraging asynchronous communication, the producer can efficiently handle message publishing without blocking the main application flow, thus contributing to overall scalability.
Creating Resilient and Self-Healing Systems
Scalable applications must also exhibit resilience and self-healing capabilities. In cloud-native environments, failure is inevitable, and applications need to be designed to gracefully handle failures without causing widespread outages.
Java provides robust libraries for implementing resilience patterns such as circuit breakers, bulkheads, and retries. Frameworks like Resilience4j and Hystrix offer comprehensive support for building fault-tolerant microservices.
// Example of using Resilience4j to decorate a method with a CircuitBreaker
@CircuitBreaker(name = "backendA")
public String performRemoteCall() {
// Make a remote call to another service
return remoteService.call();
}
In this code snippet, we apply a circuit breaker using Resilience4j to protect a method that makes a remote call. If the failure threshold is exceeded, the circuit breaker will open, preventing further calls to the remote service and allowing the system to gracefully handle the failure.
Harnessing the Power of Cloud Platforms
Cloud-native applications thrive in cloud environments, where they can fully leverage the elasticity and scalability offered by cloud providers. Platforms such as Amazon Web Services (AWS), Microsoft Azure, and Google Cloud Platform (GCP) offer a wide array of services tailored to building and running scalable applications.
By integrating with cloud-native services like AWS Lambda, Azure Functions, and GCP Cloud Functions, Java applications can achieve auto-scaling capabilities, where resources are provisioned dynamically based on incoming workload.
// Example of defining a serverless function using AWS Lambda and Java
public class MyLambdaHandler implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
// Process the input and return a result
return "Processed: " + input;
}
}
This snippet illustrates a basic AWS Lambda function written in Java. By deploying functions as serverless components, developers can benefit from automatic scaling, paying only for the resources consumed during execution.
The Last Word
In conclusion, building scalable cloud-native applications in Java requires a holistic approach that encompasses microservices architecture, asynchronous communication, resilience patterns, and cloud platform integration. By adopting these principles and best practices, developers can unlock the full potential of scalability and build resilient, high-performing applications that can effortlessly handle varying workloads.
Scaling Java applications in the cloud is not just about increasing capacity; it's about embracing a mindset that prioritizes flexibility, responsiveness, and fault tolerance. By mastering cloud-native patterns and leveraging the power of Java, developers can embark on a journey towards unlocking unparalleled scalability and building the next generation of robust, resilient, and scalable applications.