Mastering DSL in Groovy: Simplify Complex Tasks!
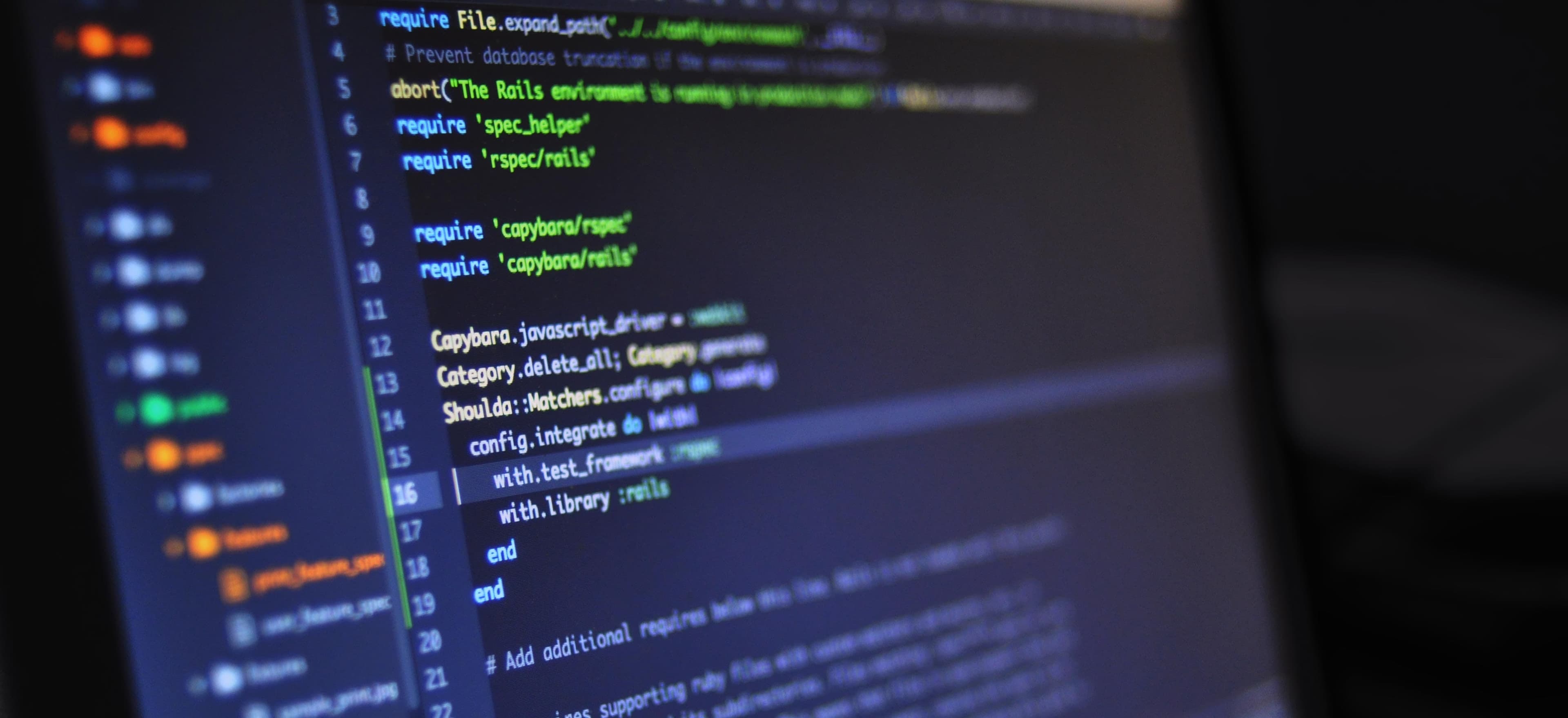
- Published on
Mastering Domain Specific Languages in Groovy: Simplify Complex Tasks!
Domain Specific Languages (DSLs) are a powerful tool in software development for expressing intricate business rules and complex logic in a clear and intuitive manner. In the world of Java and JVM languages, Groovy stands out as a language that excels in creating expressive and concise DSLs. In this blog post, we will delve into the world of DSLs in Groovy, understand their benefits, and learn how to master them for simplifying complex tasks.
What is a DSL?
A Domain Specific Language is a programming language or specification language dedicated to a particular problem domain, a particular problem representation technique, and/or a particular solution technique. A DSL is designed to be readable and expressive for users in a specific domain, making it easier to write and maintain code that deals with the complexities of that domain.
In Groovy, DSLs can be created using its rich and flexible syntax, making it an ideal choice for building powerful and intuitive DSLs.
Types of DSLs in Groovy
DSLs in Groovy can be categorized into two types: external and internal DSLs.
External DSLs
An external DSL is a separate language that is not a subset or superset of Groovy. It requires a separate parser and semantic analyzer. Although powerful, creating and maintaining an external DSL is a complex task.
Internal DSLs
An internal DSL is written in Groovy and leverages its syntax and features to create a language that feels like a native part of the Groovy code. Internal DSLs are easier to create, maintain, and integrate with existing Groovy code.
For the purpose of this blog post, we will focus on internal DSLs in Groovy.
Benefits of DSLs in Groovy
Using DSLs in Groovy offers several advantages:
-
Readability: DSLs can be designed to closely match the language of the specific domain, making the resulting code highly readable and expressive.
-
Maintainability: By abstracting complex logic into a DSL, the overall codebase becomes more maintainable and easier to understand, as the DSL serves as a clear representation of the domain's rules and logic.
-
Productivity: DSLs can boost developer productivity by reducing the cognitive load of dealing with intricate domain-specific logic in a more natural and intuitive way.
-
Collaboration: DSLs can bridge the gap between technical and non-technical stakeholders by enabling discussions and collaboration using a shared, domain-specific language.
Creating DSLs in Groovy
Now, let's dive into an example of creating an internal DSL in Groovy for configuring a simple web server. We will use Groovy's closures, method delegation, and other language features to build a concise and expressive DSL for defining server configurations.
class WebServer {
String host
int port
boolean started
def configureServer(Closure config) {
config.delegate = this
config()
}
void start() {
// Logic for starting the server
this.started = true
println("Server started at http://${host}:${port}")
}
}
def server = new WebServer()
server.configureServer {
host = "localhost"
port = 8080
}
server.start()
In this example, WebServer
class provides a configureServer
method that takes a closure as an argument. Inside the closure, we set the properties of the WebServer
instance. By delegating the closure to the instance of WebServer
, we can directly access its properties within the closure.
When we call server.start()
, the configured server is started with the provided host and port, showcasing the power of DSLs in Groovy for simplifying complex configuration tasks.
Advanced DSL Techniques in Groovy
Groovy provides advanced features that can further enhance the capabilities of DSLs:
MethodMissing and PropertyMissing
Using methodMissing
and propertyMissing
in Groovy, we can dynamically intercept method and property calls that are not defined in the class. This enables us to create more flexible and dynamic DSLs.
class Configuration {
Map config = [:]
def methodMissing(String name, args) {
if (args.size() == 1) {
config[name] = args[0]
}
}
def propertyMissing(String name) {
config[name]
}
}
def conf = new Configuration()
conf {
serverName 'MyServer'
port 8080
maxConnections 100
}
In this example, the Configuration
class uses methodMissing
to intercept method calls and store the method name and its argument as a key-value pair in the config
map. Similarly, propertyMissing
is used to handle property access and retrieval from the config
map.
Groovy Builders
Groovy provides builders that allow for creating structured data or markup in a concise and expressive manner. These builders are commonly used for creating XML, HTML, or other structured documents, making them a powerful tool for creating DSLs.
def html = new groovy.xml.MarkupBuilder()
def page = html.html {
head {
title('DSLs in Groovy')
}
body {
h1('Mastering DSLs in Groovy')
p('Simplify Complex Tasks!')
}
}
println page
In this example, the MarkupBuilder
allows for creating HTML elements and content using a DSL-like syntax, making it easy to construct complex HTML structures in a clear and concise manner.
A Final Look
Mastering DSLs in Groovy opens up a world of possibilities for simplifying complex tasks, expressing domain-specific logic in a clear and intuitive manner, and improving overall code readability and maintainability.
By leveraging Groovy's language features such as closures, method delegation, and advanced techniques like methodMissing
and propertyMissing
, developers can create powerful internal DSLs that feel like a native part of the language.
In conclusion, DSLs in Groovy are a valuable tool for developers looking to streamline and simplify complex tasks within their domain-specific code. With the right use of DSLs, developers can make their code more expressive, maintainable, and accessible to stakeholders across different domains.
Start mastering DSLs in Groovy today and witness the transformative power of simplifying complex tasks with elegant and expressive domain-specific languages!
To delve deeper into a comprehensive guide on Groovy DSLs, check out Mastering Groovy Programming: A practical guide to building enterprise-grade web applications book by R. S. Sidhu, which provides detailed insights into creating advanced DSLs in Groovy.
For more insights into powerful DSL design patterns and best practices, explore Martin Fowler's seminal work on DSLs in his blog post Language Workbenches: The Killer-App for Domain Specific Languages?.