Streamlining Java Cold Starts on AWS Lambda: A Guide
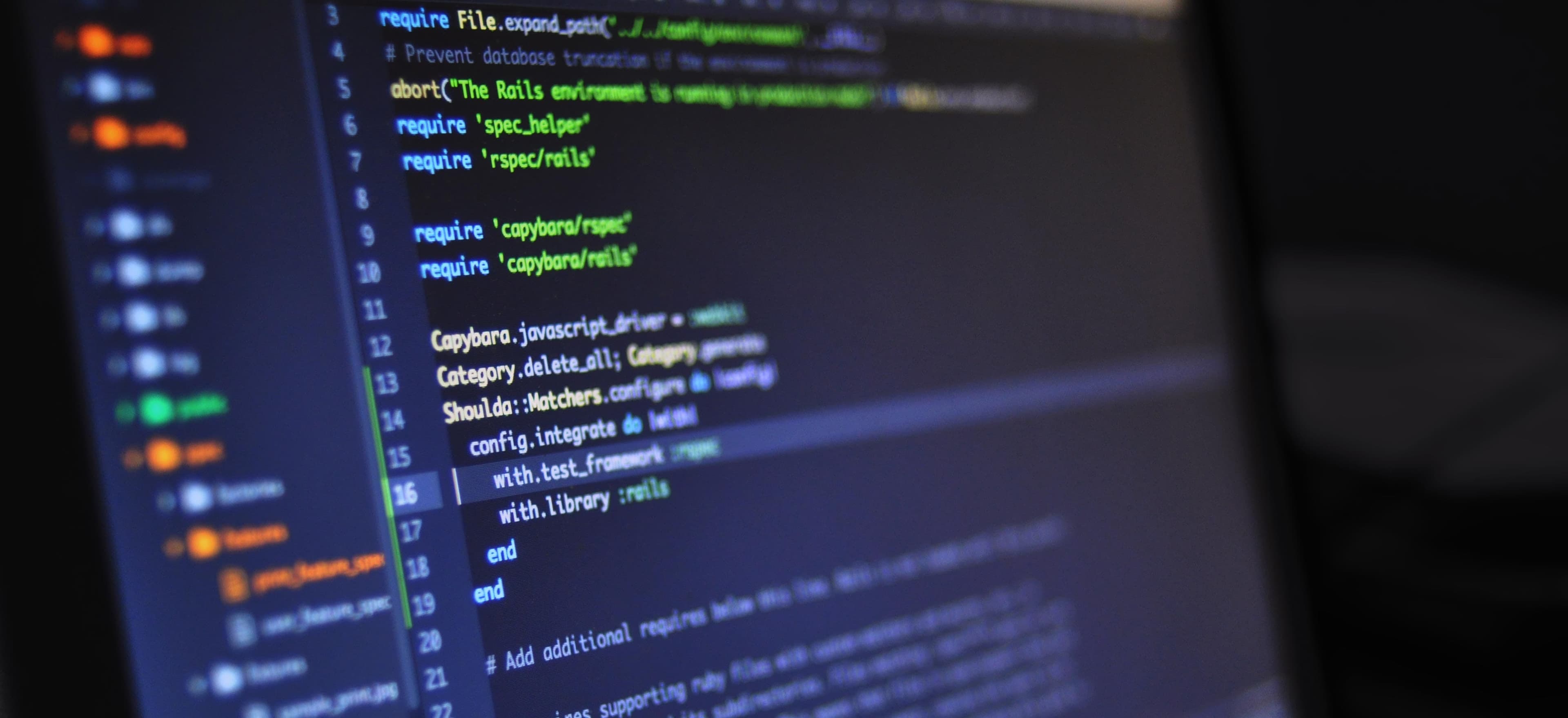
- Published on
Streamlining Java Cold Starts on AWS Lambda: A Guide
When it comes to serverless computing, AWS Lambda is a popular choice due to its flexibility, scalability, and cost-effectiveness. However, one common issue that developers encounter when using Java with AWS Lambda is the problem of cold starts. In this guide, we'll explore the concept of cold starts, how they affect Java applications on AWS Lambda, and techniques to streamline and optimize Java cold starts.
Understanding Cold Starts
What are Cold Starts?
Cold starts occur when an AWS Lambda function is invoked for the first time or after a significant period of inactivity. During a cold start, AWS Lambda initializes resources, such as creating a new container and loading the application code and dependencies. This process can result in increased latency, impacting the overall performance of the application.
Impact of Cold Starts on Java Applications
Java applications on AWS Lambda are particularly susceptible to cold starts due to the nature of the Java Virtual Machine (JVM). The JVM has an inherent startup overhead, including class loading, JIT compilation, and initialization, which can contribute to longer cold start times for Java-based Lambda functions.
Techniques for Streamlining Java Cold Starts
Reduce JAR Size
One effective way to mitigate cold starts for Java Lambda functions is by reducing the size of the application's JAR file. Smaller JAR files result in quicker download and initialization times, leading to reduced cold start latency. This can be achieved by eliminating unused dependencies, optimizing code, and employing techniques such as shading to package only the necessary dependencies.
// Example of shading plugin in Maven to create a fat JAR
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<configuration>
<createDependencyReducedPom>true</createDependencyReducedPom>
</configuration>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Use Custom Runtimes
AWS Lambda supports custom runtimes, allowing developers to use alternative runtimes for their functions. Leveraging custom runtimes tailored for Java applications can offer performance improvements, as they can be optimized specifically for AWS Lambda, reducing cold start times.
Pre-warming
Another approach to minimize the impact of cold starts is to proactively invoke Lambda functions at regular intervals to keep the containers warm. This can be achieved using scheduled tasks, event triggers, or external services to periodically invoke the Lambda functions, ensuring that they remain in a warm state and reducing the latency for subsequent invocations.
Optimize Initialization Logic
Optimizing the initialization logic of the Java application can contribute to faster cold start times. This includes minimizing static initializers, lazy-loading resources, and deferring any non-essential initialization tasks to be executed asynchronously after the function has been invoked.
Monitoring and Performance Tuning
Logging and Monitoring
Effective monitoring and logging are critical for identifying cold start issues and understanding the performance of Java Lambda functions. Utilize AWS CloudWatch logs, custom metrics, and tracing tools to gain insights into cold start times, resource utilization, and overall function performance.
Performance Tuning
Performance tuning of Java Lambda functions involves analyzing metrics, identifying bottlenecks, and implementing optimizations to improve the overall execution speed. Techniques such as memory tuning, parallelism, and concurrency management can be utilized to fine-tune the performance of Lambda functions and reduce cold start latency.
Closing the Chapter
Streamlining and optimizing Java cold starts on AWS Lambda is essential for delivering performant and responsive serverless applications. By employing techniques such as reducing JAR size, utilizing custom runtimes, pre-warming, and optimizing initialization logic, developers can significantly mitigate the impact of cold starts on Java applications running on AWS Lambda. Furthermore, continuous monitoring, logging, and performance tuning are imperative for maintaining and improving the efficiency of Java Lambda functions over time.
In conclusion, understanding the factors contributing to cold starts and implementing proactive strategies to address them is crucial for leveraging the full potential of Java applications on AWS Lambda. By combining best practices and optimization techniques, developers can ensure that their Java-based serverless applications deliver fast response times and optimal performance while minimizing the impact of cold starts.