Mastering Latency in Microservices with Netflix Hystrix
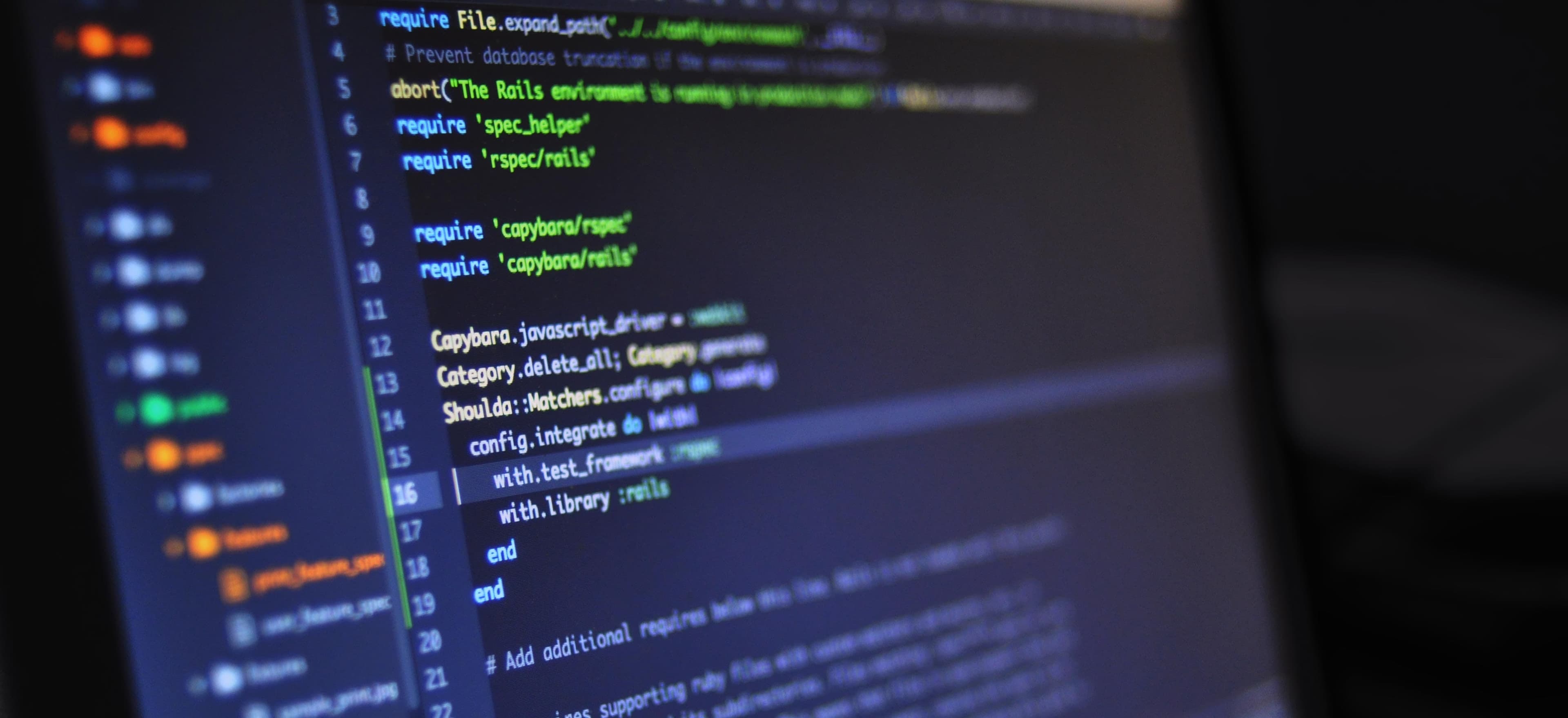
- Published on
Mastering Latency in Microservices with Netflix Hystrix
In today's fast-paced digital world, microservices architecture has become increasingly popular due to its flexibility and scalability. However, one of the challenges that microservices face is dealing with latency and failures. In a complex system with multiple interdependent services, the failure of one service can potentially cascade and impact other services, leading to degraded performance or complete system failures.
To address these challenges, Netflix open-sourced Hystrix, a library designed to control interactions between distributed services by adding latency tolerance and fault tolerance logic. In this article, we will explore how Netflix Hystrix can help us master latency in microservices and improve the overall resilience of our system.
Beginning Insights to Netflix Hystrix
Netflix Hystrix is a latency and fault tolerance library designed to isolate points of access between the services, stop cascading failures across them, and provide fallback options. The core idea behind Hystrix is to stop the failure of one service from bringing down an entire system, thereby improving system resiliency.
How Hystrix Works
At the heart of Hystrix is the concept of the circuit breaker pattern. When a service that Hystrix is monitoring reaches a predefined threshold of failures, Hystrix opens the circuit, meaning that subsequent calls to that service will automatically fail for a specified period. During this period, Hystrix allows some calls through to see if the service has recovered, and if so, the circuit is closed, and the calls are allowed to go through again.
Additionally, Hystrix provides fallback logic, which allows developers to define alternate behavior when a service call fails. This can be crucial in ensuring that the overall system remains functional even when individual services are experiencing issues.
Implementing Netflix Hystrix in Java
Let's dive into some code examples to see how we can implement Hystrix in a Java microservice. We'll use a simple Spring Boot application to demonstrate the integration.
Adding Hystrix Dependency
First, we need to add the Hystrix dependency to our pom.xml
:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
Creating a Hystrix Command
We can create a Hystrix command by extending the HystrixCommand
class:
import com.netflix.hystrix.HystrixCommand;
import com.netflix.hystrix.HystrixCommandGroupKey;
public class MyHystrixCommand extends HystrixCommand<String> {
public MyHystrixCommand() {
super(HystrixCommandGroupKey.Factory.asKey("ExampleGroup"));
}
@Override
protected String run() throws Exception {
// Perform the risky operation here
return "Result";
}
@Override
protected String getFallback() {
// Fallback logic in case of failure
return "Fallback Result";
}
}
Here, we define a class MyHystrixCommand
that extends HystrixCommand
and override the run
method to perform the actual operation we want to control. The getFallback
method provides the fallback logic in case of failure.
Using the Hystrix Command
We can then use the Hystrix command in our service:
public String performRiskyOperation() {
return new MyHystrixCommand().execute();
}
In this example, the performRiskyOperation
method uses the MyHystrixCommand
to execute the risky operation with built-in fault tolerance and fallback logic.
Monitoring Hystrix Metrics
Netflix Hystrix provides powerful metrics and monitoring capabilities out of the box. By monitoring these metrics, we can gain insights into the health and performance of our microservices.
Setting up Hystrix Dashboard
To visualize the Hystrix metrics, we can use the Hystrix Dashboard. We need to include the Hystrix Dashboard dependency in our pom.xml
:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix-dashboard</artifactId>
</dependency>
Configuring Hystrix Stream
We also need to expose the Hystrix metrics stream by adding the @EnableHystrix
annotation to our main Spring Boot application class:
import org.springframework.cloud.netflix.hystrix.EnableHystrix;
import org.springframework.cloud.netflix.hystrix.dashboard.EnableHystrixDashboard;
@SpringBootApplication
@EnableHystrix
@EnableHystrixDashboard
public class MicroserviceApplication {
public static void main(String[] args) {
SpringApplication.run(MicroserviceApplication.class, args);
}
}
Accessing Hystrix Dashboard
With the Hystrix Dashboard running, we can access the Hystrix stream endpoint, for example, http://localhost:8080/actuator/hystrix.stream
, and visualize the metrics in the Hystrix Dashboard UI.
Best Practices for Using Netflix Hystrix
While Netflix Hystrix provides powerful capabilities for managing latency and failures in microservices, it's crucial to follow best practices to ensure effective utilization:
-
Identify Critical Service Dependencies: Determine the most critical service dependencies in your system and apply Hystrix to those interactions to maximize its impact.
-
Set Meaningful Timeouts: Establish appropriate timeouts for service calls to prevent long-running operations from blocking resources.
-
Define Fallback Strategies: Always define meaningful fallback strategies to gracefully handle failures and provide a better user experience.
-
Monitor and Tune Hystrix Configurations: Regularly monitor Hystrix metrics and tune the configurations based on the observed behavior of your services.
By following these best practices, you can harness the full power of Netflix Hystrix to improve the resilience and performance of your microservices architecture.
The Bottom Line
In conclusion, Netflix Hystrix is a valuable tool for mastering latency and failures in microservices. By leveraging its circuit breaker pattern, fallback logic, and powerful monitoring capabilities, we can build more resilient and reliable microservices architectures. As the microservices landscape continues to evolve, tools like Hystrix play a vital role in ensuring the stability and performance of distributed systems.
With the growing complexity of microservices architectures, mastering latency and fault tolerance is essential, and Netflix Hystrix equips us with the necessary tools to do so effectively. By implementing Hystrix in our Java microservices, we can enhance our system's resilience and ensure a smoother, more reliable user experience.
So, embrace Netflix Hystrix and elevate your microservices to new levels of resilience!
Checkout our other articles