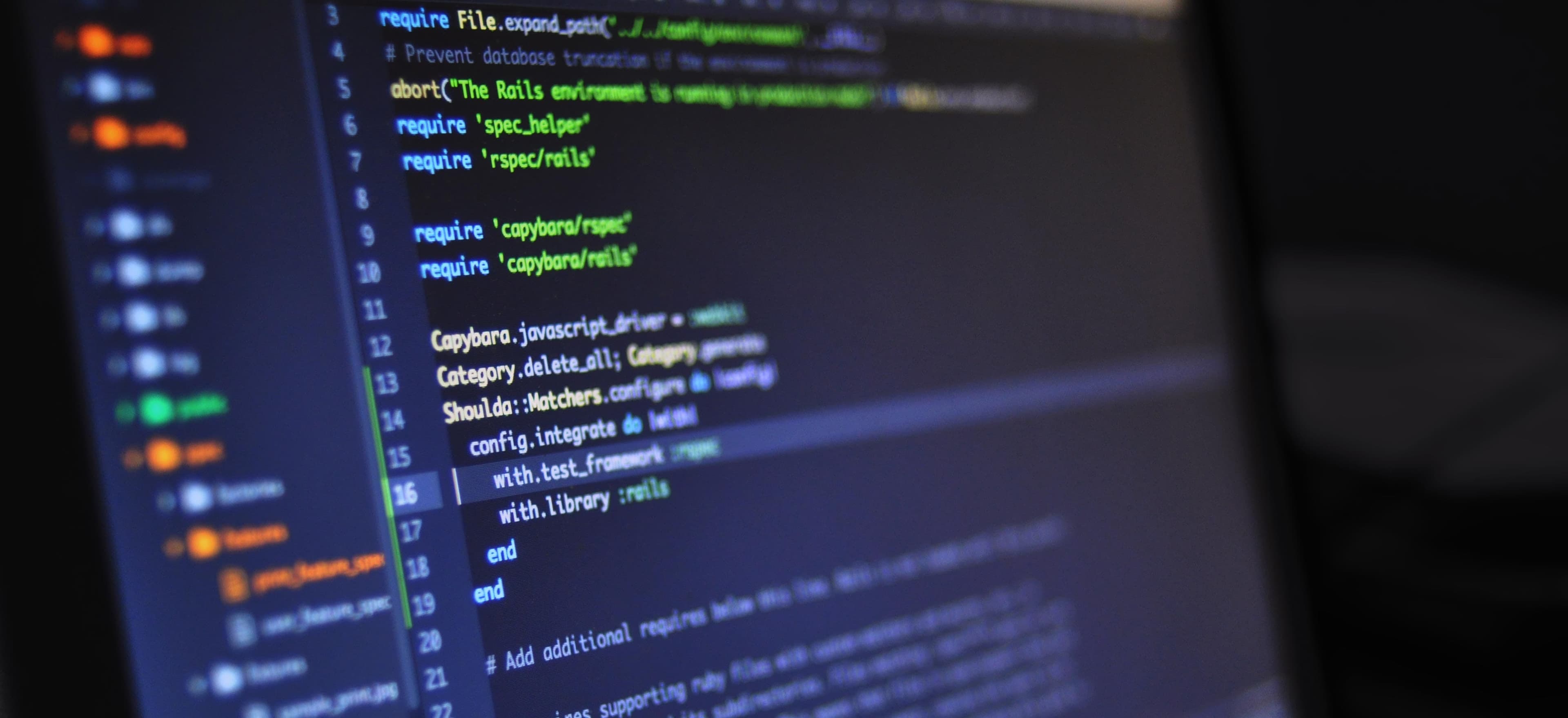
- Published on
Securing Tech Careers: Navigating Lifelong Employability in Java Development
In the ever-evolving landscape of technology, Java remains a cornerstone. Its robust framework, platform-independent nature, and widespread usage across industries make Java an ideal skill for developers seeking lifelong employability. However, with the tech industry's rapid growth and the constant emergence of new languages and frameworks, how can Java developers ensure they remain relevant and in demand? This guide will navigate you through the keys to securing a tech career with a focus on Java development, integrating both in-depth discussion and concise, actionable advice.
Mastering the Fundamentals
Before diving deep into the latest libraries or frameworks, establishing a rock-solid foundation in Java's core principles is crucial. This includes understanding object-oriented programming (OOP) concepts, such as encapsulation, inheritance, and polymorphism, which are central to Java. The official Java tutorials by Oracle provide an excellent starting point.
Consider this simple yet powerful example of encapsulation in Java:
public class Employee {
private String name;
private double salary;
public Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void raiseSalary(double byPercent) {
double raise = salary * byPercent / 100;
salary += raise;
}
}
In this code snippet, we encapsulate the Employee
's details, exposing only what's necessary through public methods. This approach enhances maintainability, a key to future-proofing your code in a rapidly changing tech landscape.
Stay Abreast of the Latest Trends
Java is constantly evolving, with new releases bringing enhancements and new features. Engaging with the Java community through forums, attending webinars, or contributing to open-source projects can keep you updated. Sites like Stack Overflow and GitHub provide invaluable resources for staying at the cutting edge.
Moreover, familiarizing yourself with the latest Java enhancements, such as the introduction of local variable type inference in Java 10, can simplify your code and make it more readable:
var list = new ArrayList<String>(); // infer ArrayList<String>
list.add("Hello");
list.add("Java 10");
System.out.println(list);
Understanding and adopting such features early on can set you apart in the job market.
Diversify Your Skill Set
While specializing in Java is beneficial, the most successful developers are often those who diversify their skill sets. Learning complementary languages such as Kotlin, which is fully interoperable with Java, or frameworks like Spring, which adds essential features for building robust applications, can significantly enhance your employability.
Additionally, familiarizing yourself with DevOps tools and practices, understanding cloud computing fundamentals, and gaining experience with containerization technologies like Docker can give you a competitive edge. A broad skill set not only makes you more versatile but also prepares you for leadership roles.
Understand the Ecosystem
Java is more than just a language; it's an ecosystem comprising various frameworks, tools, and libraries. For instance, mastering the Spring Framework (https://spring.io/) can enable you to develop enterprise-level applications efficiently. Spring simplifies Java development and promotes good design practices, making it a must-know framework for every Java developer. Here’s a basic Spring Boot application example:
@SpringBootApplication
public class SampleApplication {
public static void main(String[] args) {
SpringApplication.run(SampleApplication.class, args);
}
@RestController
class HelloController {
@GetMapping("/")
public String hello() {
return "Hello, World!";
}
}
}
By annotating our class with @SpringBootApplication
, we kickstart Spring’s auto-configuration magic, allowing us to focus on implementing functionality rather than boilerplate code.
Seek Out Mentoring and Networking Opportunities
Learning from experienced professionals can accelerate your growth exponentially. Whether it's through formal mentorship or informal networking, insights from seasoned developers can provide clarity on industry trends, best practices, and career advancement strategies. Participate actively in local meetups, online forums, and professional associations to build your network within the Java community.
Moreover, platforms like LinkedIn offer a wealth of opportunities for connecting with industry leaders and peers. Don’t hesitate to reach out for advice or discussion—most people are more than willing to share their experiences.
Embrace Continuous Learning
The tech industry doesn’t stand still, and neither should you. Continuous learning is the key to staying relevant. Luckily, there are myriad resources available, from online courses on platforms like Coursera and Udemy to certifications from Oracle that can not only boost your skills but also enhance your resume.
Here are some strategies to ensure continual growth:
- Set Learning Goals: Establish clear, achievable objectives. Whether it’s mastering a new framework every six months or contributing to an open-source project annually, goals give you direction.
- Schedule Regular Learning Sessions: Consistent, dedicated time for study and practice is more effective than sporadic, intensive periods.
- Learn by Doing: Apply what you learn through projects or contributions to real-world applications. Nothing solidifies understanding like practical experience.
Key Takeaways
Securing a tech career, particularly in Java development, requires more than just understanding syntax and basic programming concepts. It demands a commitment to mastering the fundamentals, staying updated with the latest advancements, diversifying skills, understanding the ecosystem, networking, and, most importantly, embracing continuous learning.
By following these steps, Java developers can navigate their way through the challenges and opportunities of the tech industry, ensuring lifelong employability in an ever-competitive field. Remember, in the realm of technology, stagnation is your biggest enemy. Keep moving, keep learning, and keep growing. Your future self will thank you.
Checkout our other articles