Resolving JAXB Exception Handling in Jersey REST APIs
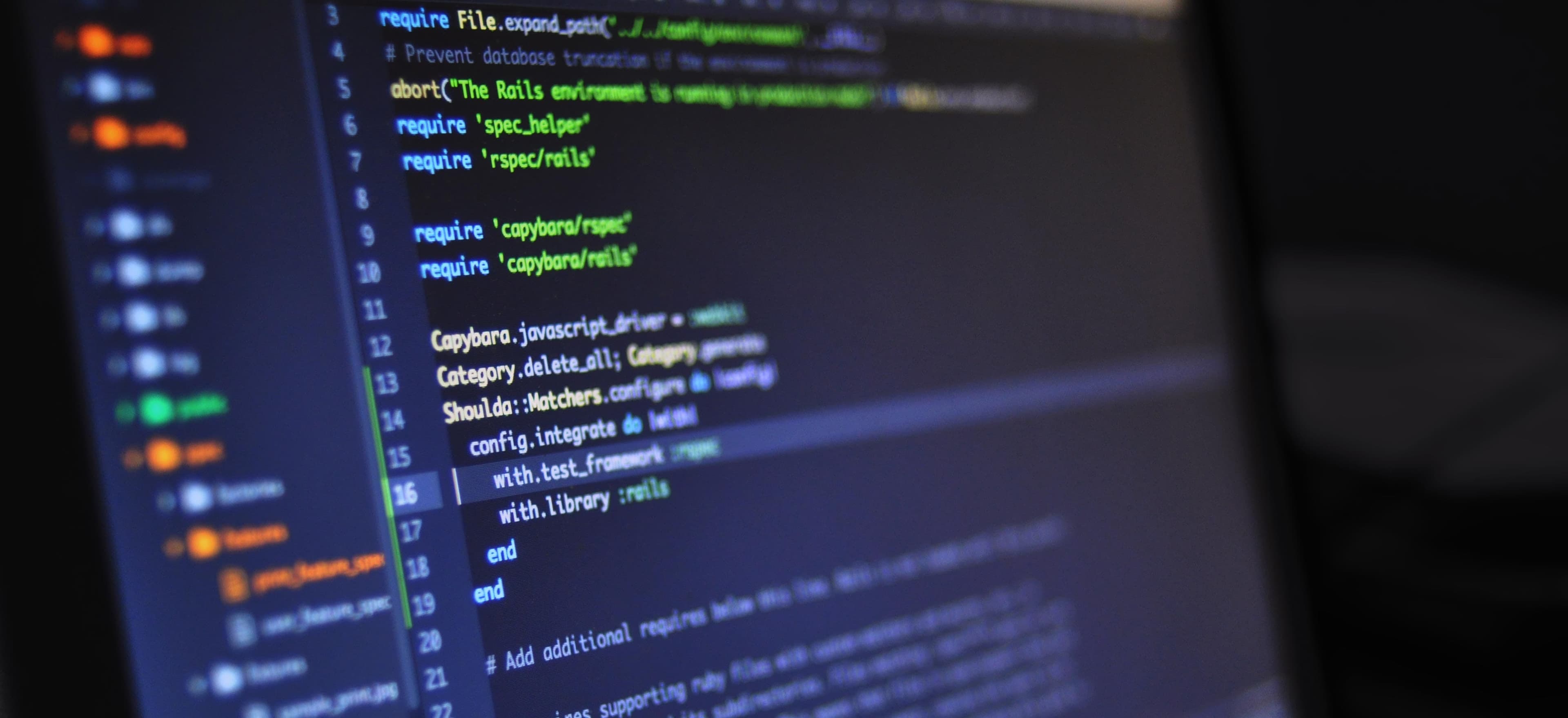
- Published on
Resolving JAXB Exception Handling in Jersey REST APIs
When developing RESTful APIs using the Jersey framework in Java, ensuring robust exception handling is crucial for maintaining the stability and reliability of your application. One common challenge developers encounter is dealing with JAXB (Java Architecture for XML Binding) exceptions when working with XML representations in their REST APIs.
In this article, we'll explore how to effectively handle JAXB exceptions in Jersey REST APIs. We'll discuss the underlying causes of these exceptions, provide practical solutions, and offer code examples to illustrate the implementation. By the end of this article, you'll have a clear understanding of how to manage JAXB exceptions in your Jersey-based RESTful applications.
Understanding JAXB Exceptions in Jersey
JAXB is used to convert Java objects to and from XML representations. When working with Jersey, which is a JAX-RS (Java API for RESTful Web Services) implementation, JAXB plays a significant role in processing XML payloads. However, during this process, various exceptions related to JAXB can occur, such as JAXBException
and its subclasses.
The most common causes of these exceptions include:
- Malformed XML input
- Inconsistent or incompatible XML schema
- Issues with XML serialization or deserialization
Dealing with these exceptions effectively is vital to ensure that your Jersey REST APIs can handle XML data gracefully and provide informative error responses when necessary.
Handling JAXB Exceptions in Jersey
To handle JAXB exceptions in your Jersey REST APIs, you can make use of exception mappers and global exception handling mechanisms provided by the framework. Exception mappers are used to map specific exception types to corresponding HTTP responses, allowing for custom error handling logic.
Let's start by creating a custom exception mapper to handle JAXBException
specifically. This mapper will catch instances of JAXBException
thrown during the processing of XML payloads and map them to appropriate HTTP responses with relevant error information.
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
import javax.xml.bind.JAXBException;
@Provider
public class JAXBExceptionMapper implements ExceptionMapper<JAXBException> {
@Override
public Response toResponse(JAXBException exception) {
// Construct a custom error response
String errorMessage = "Error processing XML payload: " + exception.getMessage();
return Response.status(Response.Status.BAD_REQUEST)
.entity(errorMessage)
.type("text/plain")
.build();
}
}
In the above code snippet, we've defined a class JAXBExceptionMapper
that implements the ExceptionMapper
interface for handling JAXBException
. The @Provider
annotation marks this class as an exception mapper, allowing Jersey to recognize and register it automatically.
Within the toResponse
method, we construct a custom error message using the details from the caught JAXBException
, and then build an HTTP response with an appropriate status code (in this case, 400 Bad Request
) and the error message as the response entity.
By implementing this custom exception mapper, any JAXBException
thrown within your Jersey resources will be intercepted and handled according to the logic defined in the JAXBExceptionMapper
.
Global Exception Handling with ExceptionMapper
In addition to creating specific exception mappers for individual exception types, Jersey also allows for global exception handling using a generic ExceptionMapper
. This approach enables you to define a single mapper to catch any unhandled exceptions thrown within your resources, including those related to JAXB processing.
Let's create a global exception mapper that covers all Exception
types, providing a generic error response for any unhandled exceptions within our Jersey application.
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
@Provider
public class GlobalExceptionMapper implements ExceptionMapper<Exception> {
@Override
public Response toResponse(Exception exception) {
// Construct a generic error response
String errorMessage = "An unexpected error occurred: " + exception.getMessage();
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity(errorMessage)
.type("text/plain")
.build();
}
}
In the code above, we've defined a global exception mapper GlobalExceptionMapper
that captures any Exception
not explicitly handled by other specific mappers. The @Provider
annotation indicates that this mapper should be globally registered and applied to all exceptions within the Jersey application.
Within the toResponse
method, we construct a generic error message for any unhandled exceptions and build an HTTP response with a status code of 500 Internal Server Error
.
By utilizing both specific and global exception mappers, you can establish comprehensive exception handling mechanisms for your Jersey REST APIs, ensuring that JAXB-related exceptions are managed effectively while also addressing unforeseen errors that may arise.
A Final Look
In this article, we've delved into the realm of JAXB exception handling within Jersey REST APIs. We've explored the causes of JAXB exceptions, discussed the importance of robust exception handling, and provided practical solutions using exception mappers in Jersey.
By implementing custom exception mappers for JAXB-related exceptions and establishing a global exception handler, you can fortify your Jersey-based RESTful applications against unexpected errors and ensure that XML payloads are processed gracefully, with informative error responses when necessary.
Handling JAXB exceptions effectively is essential for delivering a reliable and resilient API, and Jersey's exception handling capabilities provide the tools you need to achieve this goal seamlessly.
Now that you understand the intricacies of managing JAXB exceptions in Jersey, you can apply these techniques to enhance the exception handling capabilities of your own RESTful APIs built with Jersey.
Remember, mastering exception handling is a crucial aspect of becoming a proficient Java developer, especially when working with RESTful services. Continuously refining your skills in this area will undoubtedly contribute to the robustness and stability of your applications.
For more in-depth insights on Java exception handling, consider reading Java Exception Handling Best Practices for comprehensive guidance on effectively managing exceptions in Java applications.
Start fortifying your Jersey REST APIs today with superior JAXB exception handling, and elevate the reliability and resilience of your applications to new heights. Happy coding!
Checkout our other articles