Unlocking Java: Choosing the Right Lock Implementation
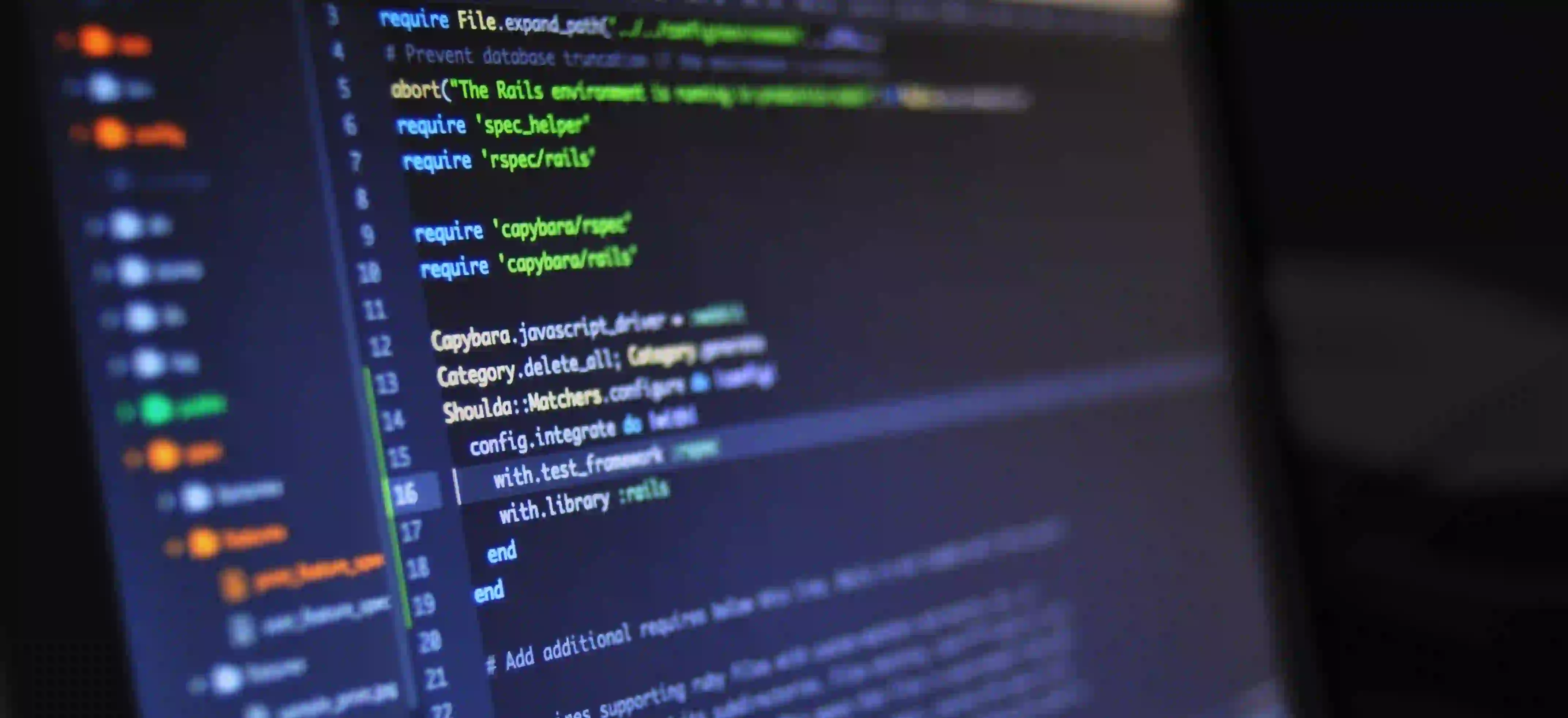
Unlocking Java: Choosing the Right Lock Implementation
In the realm of Java programming, concurrency is a fundamental concept that enables the execution of multiple threads simultaneously, improving the performance and scalability of applications. However, managing concurrency correctly is paramount to avoid issues such as thread interference and memory consistency errors. One of the key tools in the Java concurrency toolkit is the use of locks. Choosing the right lock implementation can significantly enhance the performance and reliability of your Java applications. In this blog post, we will delve deep into various lock implementations provided in Java, their use cases, and how to select the appropriate one for your specific needs.
Synchronized Blocks vs. Lock Interfaces
Before exploring the different lock implementations, it's crucial to understand the distinction between synchronized blocks and lock interfaces in Java. Synchronized blocks or methods are the most fundamental way to prevent concurrent access to a critical section of code:
public synchronized void accessResource() {
// critical section code
}
While synchronized blocks are straightforward and suitable for many situations, they lack flexibility. For instance, they do not allow attempts to acquire a lock without waiting indefinitely, nor do they support interruptible lock acquisitions or the implementation of non-block-structured locking disciplines.
Enter the java.util.concurrent.locks
package, introduced in Java 5, which provides a framework for lock implementations with advanced locking mechanisms and features. The two most commonly used interfaces from this package are Lock
and ReadWriteLock
.
The Lock Interface
The Lock
interface provides more extensive locking operations than synchronized blocks. It allows greater control over the lock acquisition and release process. The following is a simple example of using a ReentrantLock
, a popular implementation of the Lock
interface:
Lock lock = new ReentrantLock();
public void accessResource() {
lock.lock();
try {
// critical section code
} finally {
lock.unlock();
}
}
Why use ReentrantLock
? It offers several advantages, such as the ability to attempt to acquire a lock with a timeout (tryLock
method), the capability to interrupt the lock waiting thread (lockInterruptibly
method), and the provision to query the lock’s status (e.g., isLocked
method).
ReadWriteLock Interface
When the application frequently reads from a shared resource but rarely writes to it, using a ReadWriteLock
can improve efficiency. A ReadWriteLock
maintains a pair of associated locks, one for read-only operations and another for writing. This separation allows multiple threads to read data simultaneously, as long as there's no thread writing to it. The ReentrantReadWriteLock
class is an implementation that supports reentrant and therefore recursive read and write locks. Here's an example:
ReadWriteLock readWriteLock = new ReentrantReadWriteLock();
Lock readLock = readWriteLock.readLock();
Lock writeLock = readWriteLock.writeLock();
public void readResource() {
readLock.lock();
try {
// read-only code
} finally {
readLock.unlock();
}
}
public void writeResource() {
writeLock.lock();
try {
// writing code
} finally {
writeLock.unlock();
}
}
The beauty of this approach lies in its capacity to significantly reduce contention and improve throughput in data-reading scenarios, which are common in real-world applications.
Choosing the Right Lock
Selecting the correct lock implementation depends on various factors such as the nature of the resource, the expected contention level, and specific application requirements. Here's a quick guide:
- Use synchronized blocks/methods when you need simplicity and your critical section is brief and unlikely to cause significant contention.
- Opt for
ReentrantLock
when you require advanced features like timed lock waits, interruptible lock acquisition, or non-block-structured locking. - Choose
ReentrantReadWriteLock
when you have a high ratio of read operations compared to write operations, and you aim to improve performance through read concurrency.
It's essential to remember that while locks help manage concurrency, they can also introduce potential issues such as deadlocks and reduced scalability. Therefore, always aim to minimize lock contention and hold locks for as short a duration as possible.
Closing Remarks
Java provides a robust set of locking mechanisms through synchronized blocks and the java.util.concurrent.locks
package, which includes the Lock
and ReadWriteLock
interfaces. Each has its specific use cases and advantages. By understanding these tools and choosing the right lock implementation, you can effectively manage thread safety and concurrency in your Java applications, leading to improved performance and reliability.
For further reading and to deepen your understanding, the Oracle documentation provides an excellent overview of Java's Lock objects and the broader topic of Concurrency.
Concurrent programming in Java can be complex, but by mastering locks and understanding when and how to use them, you unlock the potential to create high-performing, thread-safe applications. Dive into these implementations, experiment with them, and watch your Java applications thrive in multi-threaded environments.