Boosting Quarkus Projects: Mastering Neo4j OGM Integration
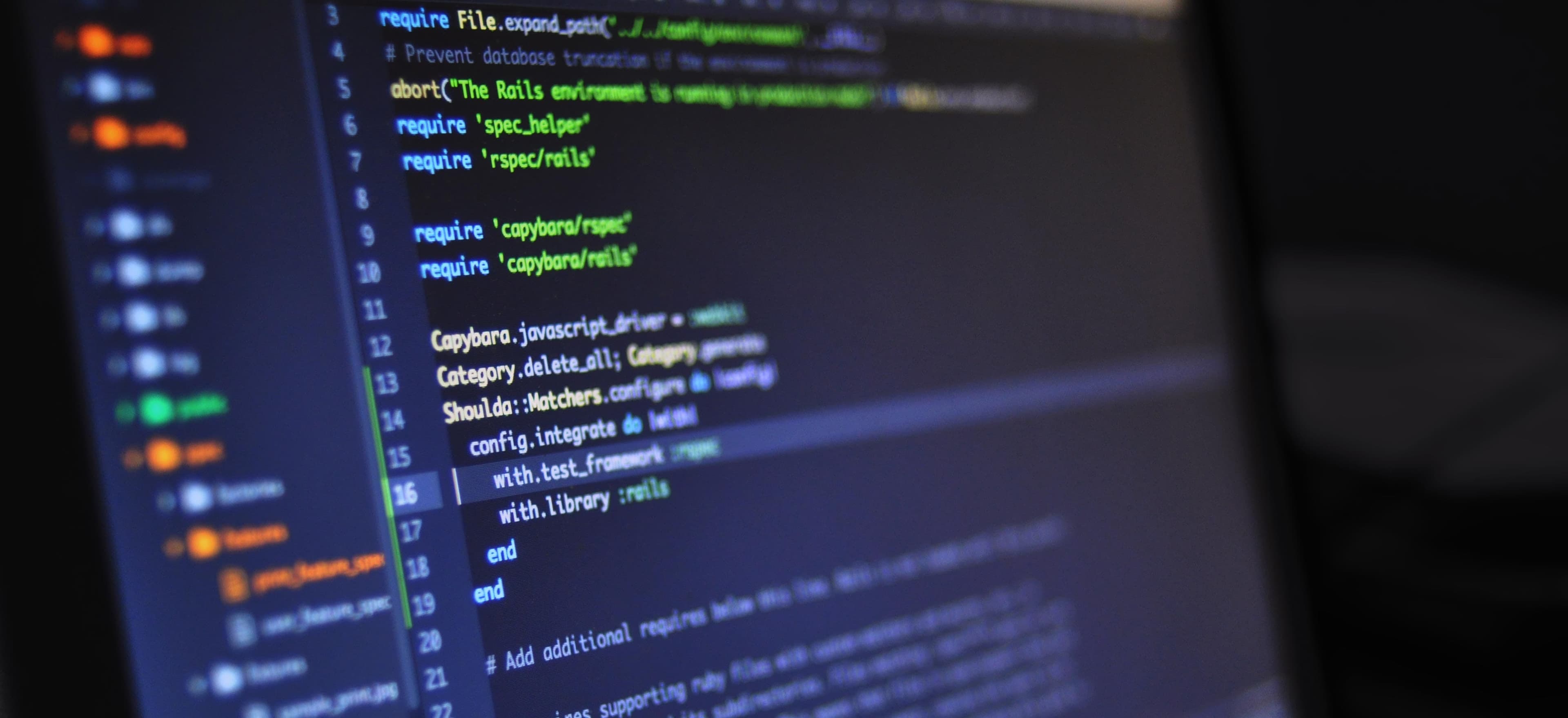
- Published on
Boosting Quarkus Projects: Mastering Neo4j OGM Integration
Java has long been a prominent language for developing robust and scalable web applications. However, the landscape of Java development is continually evolving, and modern requirements demand solutions that are optimized for cloud-native, serverless, and Kubernetes environments. This has led to the emergence of Quarkus, a Kubernetes Native Java stack tailored for GraalVM and HotSpot. Quarkus is designed to optimize Java specifically for containers, making it a lightweight and fast platform for modern Java applications.
In this article, we will explore the Quarkus framework and its distinctive advantages. We will then delve into Neo4j, a native graph database known for its flexibility and scalability, and discuss the concept of Object Graph Mapping (OGM). Finally, we will provide a step-by-step guide to effectively integrating Neo4j OGM with Quarkus projects and share best practices for maximizing performance.
Prerequisites
Before starting with Quarkus and Neo4j OGM integration, ensure you have the following prerequisites in place:
- Basic understanding of Java programming
- Familiarity with database concepts
- Knowledge about graph databases
- Installed software such as Java 11 or newer, Quarkus, and Neo4j (latest version)
With these prerequisites met, you are ready to dive into the integration process.
Quarkus and Its Edge in Modern Java Development
Quarkus has gained substantial traction in the Java development community due to its revolutionary features. These include:
Why Quarkus?
Developers are increasingly drawn to Quarkus due to its live coding feature, support for both imperative and reactive programming models, and its ability to bring together a wide range of Java standards and frameworks.
Key Advantages
Some of the key advantages of using Quarkus include its developer-centric approach, unified configuration system, vibrant community, and extensive documentation. Additionally, Quarkus offers seamless cloud and Kubernetes integration, which is essential for modern Java applications.
Deploying Quarkus applications to the cloud or orchestrating them in Kubernetes environments has become more straightforward, thanks to its container-first philosophy, reduced memory footprint, and fast boot time.
Neo4j: The Graph Database Revolution
Neo4j is a leading graph database known for its ability to harness data relationships as first-class entities. Its core features and the power of the Cypher query language make it a strong contender for handling complex, connected data.
Why Graph Database?
The rise of graph databases can be attributed to their unique ability to manage highly connected data scenarios that traditional databases struggle to address. Neo4j shines in use cases such as social networks, recommendation systems, and fraud detection, where relationships between data points play a crucial role.
Benefits of Neo4j
The flexibility and performance optimization offered by Neo4j for handling connected data, along with the powerful Cypher query language, make it a compelling choice for modern applications.
Step-by-Step Guide to Integrating Neo4j OGM in Quarkus Projects
Now, let's delve into the step-by-step process of integrating Neo4j Object Graph Mapping (OGM) in Quarkus projects:
Step 1: Setting Up Your Quarkus Project
You can begin by initializing a new Quarkus project using Maven or a preferred tool. To create a basic REST application template, use the following command:
mvn io.quarkus:quarkus-maven-plugin:1.13.7.Final:create \
-DprojectGroupId=com.example \
-DprojectArtifactId=my-quarkus-project \
-DclassName="com.example.GreetingResource" \
-Dpath="/hello"
Step 2: Configuring Neo4j OGM
Include the Neo4j dependencies in the pom.xml
file. You can add the necessary dependencies for Neo4j OGM and the driver as follows:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-neo4j</artifactId>
</dependency>
<dependency>
<groupId>org.neo4j.ogm</groupId>
<artifactId>neo4j-ogm-bolt-driver</artifactId>
<version>3.2.25</version>
</dependency>
Additionally, configure the connection to the Neo4j server in the application.properties
file.
Step 3: Defining Entity and Repository
Create the domain models and repositories, mapping entities to graph nodes and relationships using annotations. For example:
@Node
public class Person {
@Id
@GeneratedValue
private Long id;
@Property(name = "name")
private String name;
@Relationship(type = "FRIEND_OF", direction = Relationship.INCOMING)
private Set<Person> friends;
}
Step 4: Implementing Service Logic
Implement the service layer to handle business logic, leveraging the repository to interact with the database:
@ApplicationScoped
public class PersonService {
@Inject
Neo4jTemplate neo4jTemplate;
@Transactional
public Person createPerson(String name) {
Person person = new Person(name);
return neo4jTemplate.save(person);
}
}
Step 5: Creating REST Endpoints
Expose the service logic through RESTful APIs using Quarkus. Provide code snippets for a basic CRUD API, explaining each part of the code briefly:
@Path("/persons")
@Produces(MediaType.APPLICATION_JSON)
@Consumes(MediaType.APPLICATION_JSON)
public class PersonResource {
@Inject
PersonService personService;
@POST
public Response createPerson(Person person) {
Person createdPerson = personService.createPerson(person.getName());
URI createdUri = UriBuilder.fromResource(PersonResource.class)
.path(PersonResource.class, "getPerson")
.build(createdPerson.getId());
return Response.created(createdUri)
.entity(createdPerson)
.build();
}
}
Testing the Integration
Writing tests for the Quarkus application involves focusing on unit tests for the service layer and integration tests involving the Neo4j database. Use tools and annotations such as JUnit and @QuarkusTest
for running integration tests.
Best Practices for Optimal Performance
When using Neo4j OGM with Quarkus, it is crucial to prioritize performance optimization. Understanding and implementing query optimization, efficient graph modeling, and leveraging Quarkus’ reactive programming model for non-blocking database calls can significantly enhance the performance of your application.
Final Thoughts
The integration of Neo4j OGM with Quarkus projects unlocks the potential to handle complex data relationships efficiently. By combining the strengths of Quarkus and Neo4j, developers can build high-performance applications tailored for modern, cloud-native environments. With this step-by-step guide and best practices in mind, you can confidently explore the world of graph database integration in your Quarkus projects.
In the rapidly evolving world of Java development, the synergy between Quarkus and Neo4j offers an exciting opportunity for developers to build innovative and scalable applications.
References and Further Reading
For further information and learning resources, refer to the following:
- Quarkus Official Documentation
- Neo4j Official Documentation
- Quarkus GitHub Repository
- Neo4j GitHub Repository
- Blogs, tutorials, and courses on Quarkus and Neo4j available on platforms such as Baeldung, Medium, and Udemy.
Remember, continued experimentation and exploration are key to mastering the integration of Neo4j OGM with Quarkus projects.
With the comprehensive understanding of the Quarkus framework and Neo4j OGM integration, developers are equipped to elevate the capabilities and performance of their Java applications. This article provides a detailed guide for integrating Neo4j OGM with Quarkus projects, empowering developers to explore the potential of graph databases in modern Java development.