Unlocking Power: Enhanced Enums in Java Use Cases
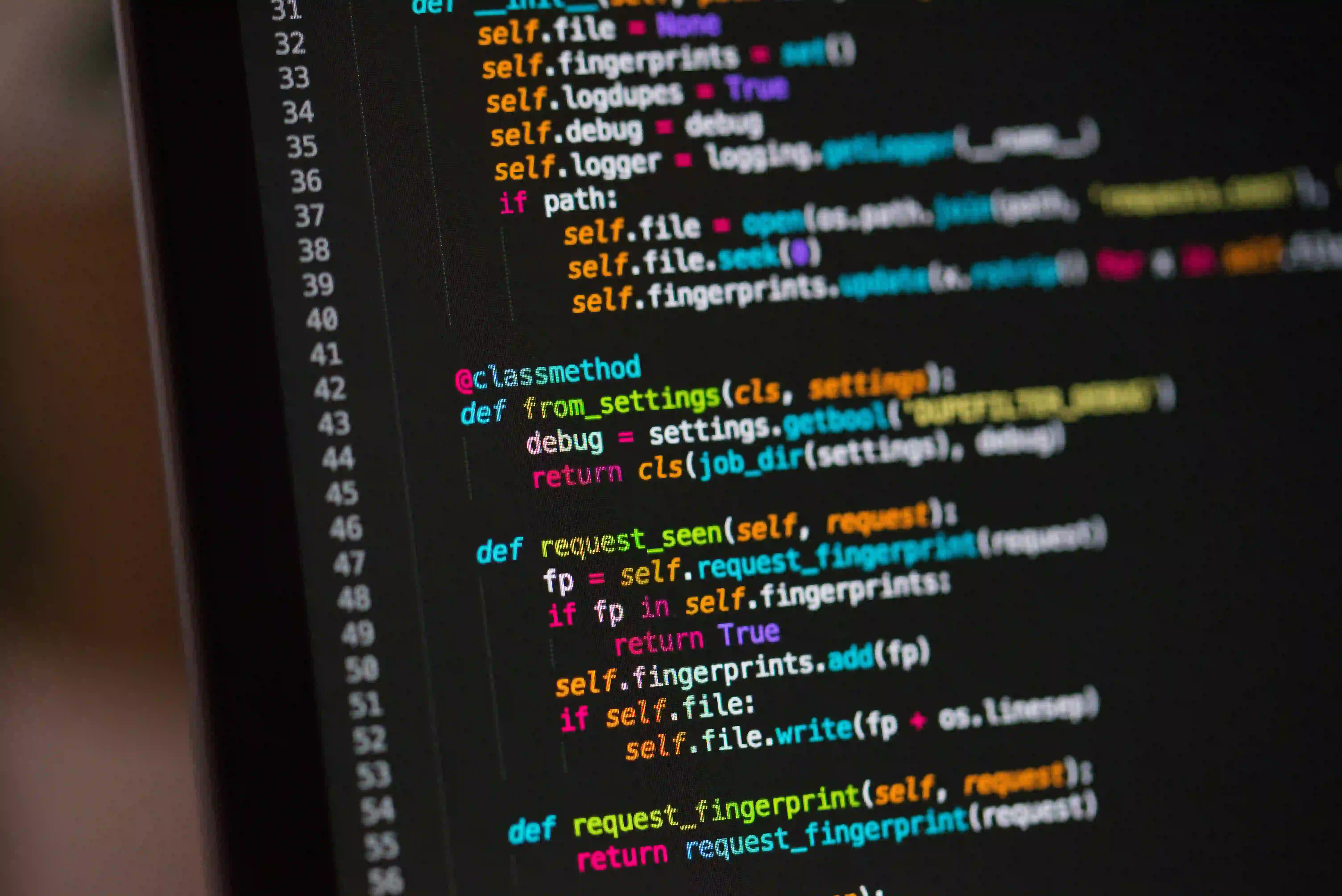
Unlocking Power: Enhanced Enums in Java Use Cases
Enums, short for enumerations, are a crucial part of Java programming. They enable the creation of a set of named constants and provide a more structured approach compared to primitive data types. While traditional enums are effective for creating a fixed set of constants, enhanced enums take this a step further by adding behaviors and properties to enums, providing a more versatile and powerful tool for Java developers.
In this article, we will explore enhanced enums in Java, their benefits, and various use cases where they can be leveraged to unlock their true potential.
Traditional Enums vs. Enhanced Enums
Traditional enums in Java are a way to define a fixed set of constants. For example, consider a simple enum representing the days of the week:
public enum Day {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY
}
With traditional enums, you can only represent the constant values. However, enhanced enums allow you to attach behaviors, methods, and properties to the enum constants, making them more powerful and flexible.
Benefits of Enhanced Enums
Enhanced enums offer several advantages over traditional enums, including:
-
Additional Behaviors: Enhanced enums can have methods, allowing each constant to exhibit specific behaviors.
-
Custom Data: They can contain custom data fields, providing more information associated with each enum constant.
-
Immutability: Enhanced enums are immutable by default, ensuring that once defined, the enum constants cannot be changed.
-
Inheritance: They can implement interfaces and extend classes, enabling enhanced enums to conform to a specific type or behavior.
Let's delve into some use cases where enhanced enums truly shine in Java development.
Use Case 1: Enum for Configuration Properties
Suppose we want to represent different environment configurations such as Development, Testing, and Production. Traditional enums alone wouldn't suffice as they cannot hold additional configuration-specific properties. With enhanced enums, we can attach properties to each environment configuration, like API endpoints, database connection details, etc., providing a more structured and organized approach.
public enum Environment {
DEVELOPMENT("http://localhost:8080", "dev-db-url"),
TESTING("http://test-server:8080", "test-db-url"),
PRODUCTION("http://prod-server:8080", "prod-db-url");
private final String apiUrl;
private final String dbUrl;
Environment(String apiUrl, String dbUrl) {
this.apiUrl = apiUrl;
this.dbUrl = dbUrl;
}
public String getApiUrl() {
return apiUrl;
}
public String getDbUrl() {
return dbUrl;
}
}
In this example, the Environment
enum not only represents the different environments but also includes properties for API URL and database URL for each environment.
Use Case 2: Enum for Command Line Options
When building command-line applications, parsing and handling options is a common requirement. Enhanced enums can be used to define and handle command line options in a concise and type-safe manner.
public enum CommandLineOption {
HELP("h", "Print help message"),
VERSION("v", "Print version"),
VERBOSE("V", "Enable verbose mode");
private final String option;
private final String description;
CommandLineOption(String option, String description) {
this.option = option;
this.description = description;
}
public String getOption() {
return option;
}
public String getDescription() {
return description;
}
}
In this case, the CommandLineOption
enum encapsulates the command line options and their descriptions, making it easier to manage and process them within the application.
Use Case 3: Enum for HTTP Methods
HTTP methods, such as GET, POST, PUT, and DELETE, are fundamental in web development. By using enhanced enums, we can encapsulate not only the method names but also associated behaviors for each HTTP method.
public enum HttpMethod {
GET {
@Override
public void performRequest(String url) {
// Perform GET request
}
},
POST {
@Override
public void performRequest(String url) {
// Perform POST request
}
},
PUT {
@Override
public void performRequest(String url) {
// Perform PUT request
}
},
DELETE {
@Override
public void performRequest(String url) {
// Perform DELETE request
}
};
public abstract void performRequest(String url);
}
In this example, each HTTP method enum constant has its behavior defined, allowing for easy invocation of the associated request handling logic.
Final Thoughts
Enhanced enums in Java unlock a new level of power and flexibility by allowing the attachment of behaviors, methods, and properties to enum constants. They find applications in various scenarios such as configuration management, command-line parsing, and encapsulating behaviors related to fundamental concepts like HTTP methods.
By leveraging enhanced enums, Java developers can write cleaner, more organized, and maintainable code, ultimately leading to more efficient and scalable applications.
In conclusion, enhanced enums are a valuable addition to Java's arsenal, empowering developers to encapsulate both data and behavior within a single, cohesive construct, thus enhancing the readability, maintainability, and usability of their code.
To explore more about enums in Java, you can delve into the official Oracle documentation and deepen your understanding of this powerful data type.
So, the next time you find yourself working with a fixed set of constants with associated behaviors and properties, consider harnessing the power of enhanced enums to elevate your Java programming skills and build more robust and scalable applications.