Master Design Patterns: Your Ultimate Cheat Sheet Guide!
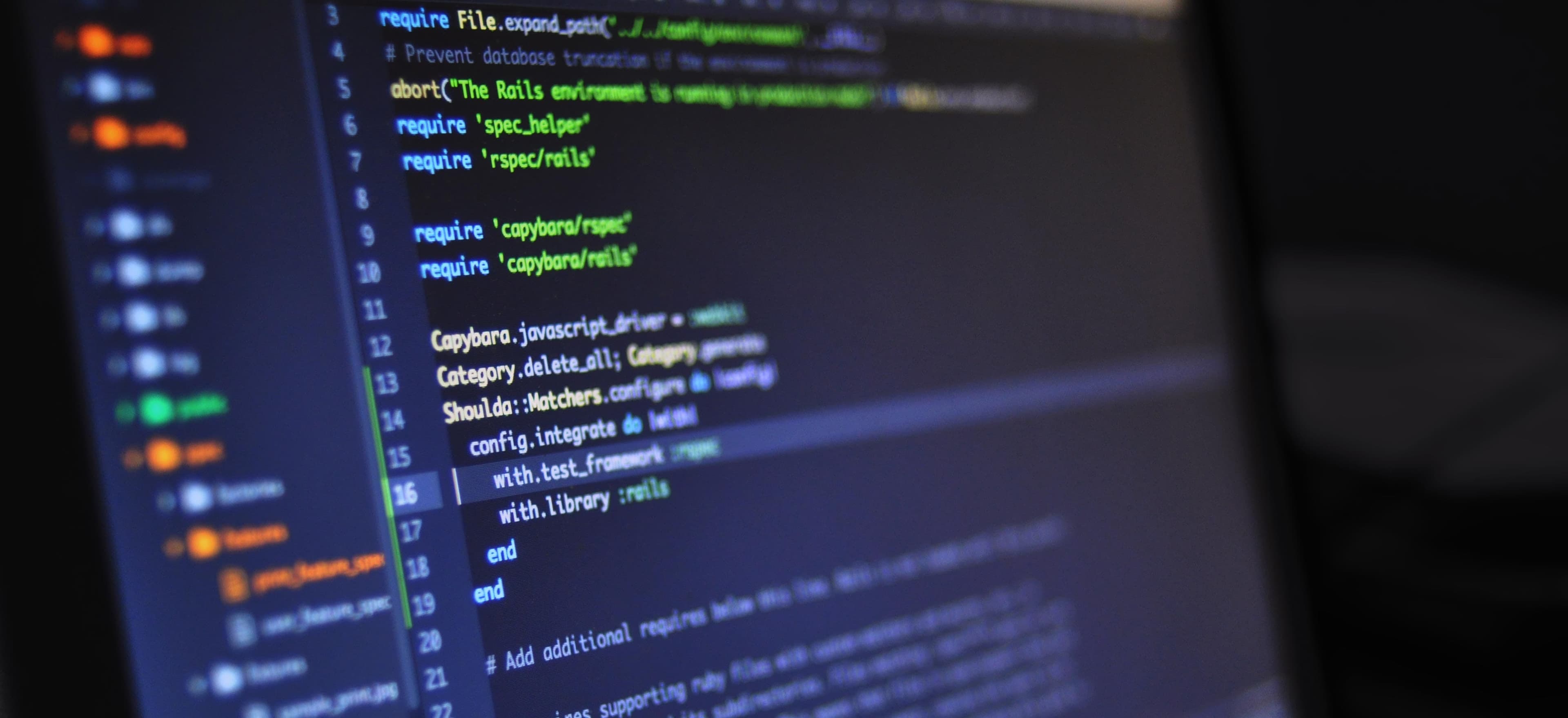
- Published on
Master Design Patterns: Your Ultimate Cheat Sheet Guide!
Design patterns are a lifesaver for software developers. They represent solutions to problems that crop up repeatedly in software design, allowing programmers to avoid reinventing the wheel. Java, with its robust features and strong community support, stands as one of the best languages to implement these patterns with elegance and ease. If you’re looking to sharpen your coding craft, you’ve come to the right place! Let's dive into the world of design patterns and how you can use them to craft maintainable, scalable, and clean Java code.
The Essence of Design Patterns in Java
Design patterns offer a high-level language of discourse for developers, a way to communicate architecture with standard terms. These patterns are categorized broadly under three heads:
- Creational: Concerned with the way objects are created.
- Structural: Focus on composing classes or objects to form larger structures.
- Behavioral: Deal with object interaction and responsibility.
As a Java developer, mastering these patterns will help you understand and solve complex architectural issues using proven methodologies. Let's take a closer took at some of the most common and useful patterns in each category.
Creational Patterns: The Art of Object Creation
Singleton Pattern
The Singleton pattern ensures a class has only one instance and provides a global point of access to that instance. It is particularly useful when exactly one object is needed to coordinate actions across the system.
public class Database {
private static Database instance;
private Database() {
// private constructor to prevent instantiation
}
public static Database getInstance() {
if (instance == null) {
instance = new Database();
}
return instance;
}
}
Here's why the code works: The constructor is private, which prevents instantiation from outside the class. The getInstance
method creates a new instance if it does not exist and returns it.
Factory Method Pattern
In contrast to Singleton, sometimes we want to create multiple instances but the process involved is complex. The Factory Method pattern offers an interface for creating objects but allows subclasses to alter the type of objects that will be created.
public abstract class Plan {
protected double rate;
abstract void getRate();
public void calculateBill(int units){
System.out.println(units*rate);
}
}
public class DomesticPlan extends Plan {
public void getRate() {
rate = 3.50;
}
}
The getRate
method is key here: each subclass of Plan
can define its own rate without altering the logic of calculateBill
.
Structural Patterns: Building Strong Relations
Adapter Pattern
Dealing with incompatible interfaces? The Adapter pattern acts as a bridge between two incompatible interfaces. This type of design pattern comes under structural pattern because this pattern combines the capability of two independent interfaces.
public interface MediaPlayer {
void play(String audioType, String fileName);
}
public class MediaAdapter implements MediaPlayer {
AdvancedMediaPlayer advancedMusicPlayer;
public MediaAdapter(String audioType) {
if(audioType.equalsIgnoreCase("vlc") ){
advancedMusicPlayer = new VlcPlayer();
}
}
@Override
public void play(String audioType, String fileName) {
if(audioType.equalsIgnoreCase("vlc")){
advancedMusicPlayer.playVlc(fileName);
}
}
}
This code allows a MediaPlayer
to use functionality of an AdvancedMediaPlayer
through composition—note MediaAdapter
implements MediaPlayer
but contains an AdvancedMediaPlayer
.
Decorator Pattern
Need to add functionality to objects without altering their structure? Decorator pattern lets you attach new behaviors to objects by placing them inside special wrapper objects that contain the behaviors.
public interface Car {
void assemble();
}
public class SportsCar extends CarDecorator {
public SportsCar(Car car) {
super(car);
}
@Override
public void assemble(){
super.assemble();
System.out.print(" Adding features of Sports Car.");
}
}
The SportsCar
class doesn't change the structure of Car
objects but adds new functionality. This is an excellent example of open/closed principle—one of the key principles of solid object-oriented design.
Behavioral Patterns: Smarter Interaction
Observer Pattern
The Observer pattern is used when there is one-to-many relationship between objects such as if one object is modified, its dependent objects are to be notified automatically. It encapsulates the core idea of reactive programming.
public class Subject {
private List<Observer> observers = new ArrayList<Observer>();
private int state;
public void setState(int state) {
this.state = state;
notifyAllObservers();
}
public void attach(Observer observer){
observers.add(observer);
}
public void notifyAllObservers(){
for (Observer observer : observers) {
observer.update();
}
}
}
public abstract class Observer {
protected Subject subject;
public abstract void update();
}
public class HexaObserver extends Observer {
public HexaObserver(Subject subject){
this.subject = subject;
this.subject.attach(this);
}
@Override
public void update() {
System.out.println( "Hex String: " + Integer.toHexString( subject.getState() ).toUpperCase() );
}
}
The Subject
keeps track of Observer
s and notifies them of any changes in its state. HexaObserver
merely receives these notifications and acts accordingly.
Design patterns empower you to leverage best practices and create robust, highly maintainable Java code. They serve as a shared vocabulary for designers and engineers.
For more in-depth examples and detailed explanations of these patterns and more, consider visiting Refactoring Guru, an excellent resource with a comprehensive list of design patterns and their use cases. Additionally, exploring the GoF’s seminal book, "Design Patterns: Elements of Reusable Object-Oriented Software", will provide a deeper conceptual background and a historical perspective on the subject.
In conclusion, while design patterns are powerful tools, remember that they're not silver bullets. They should only be applied when suitable, not forced into scenarios where they don’t fit. Strive for simplicity and don’t let your pursuit of pattern perfection cloud your judgement; sometimes, a straightforward solution is all that’s required.
Happy coding, fellow Java enthusiasts! May your code be clean and your architecture resilient!