Boost Java Speed: Mastering Garbage Collector Tuning
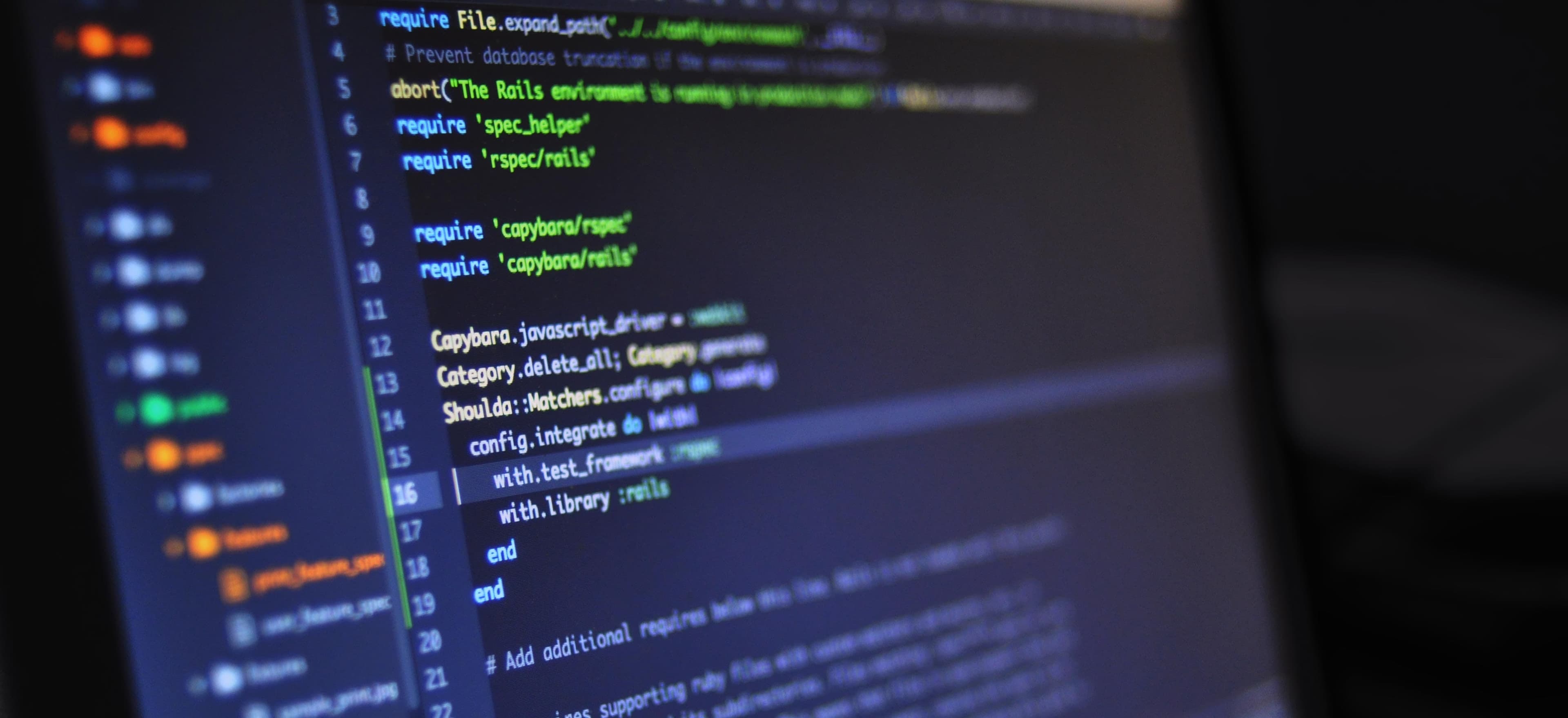
- Published on
Boost Java Speed: Mastering Garbage Collector Tuning
A Quick Look
Java memory management is a critical aspect of optimizing the performance of Java applications. The Java Garbage Collector (GC) plays a key role in memory management by automatically reclaiming memory that is no longer in use. Garbage collection significantly contributes to Java application performance by preventing memory leaks, reducing memory fragmentation, and ensuring efficient memory allocation.
In this article, we will focus on garbage collector tuning and how it can boost the speed of Java applications. Garbage collector tuning involves adjusting various parameters to optimize the performance of the garbage collector based on the specific requirements and characteristics of the application.
Understanding Java Garbage Collection
The Java Garbage Collector is responsible for automatically reclaiming memory that is no longer used by the application. It identifies objects that are no longer reachable through references and marks them for deletion. This automated memory management alleviates the need to manually allocate and deallocate memory, which greatly simplifies programming in Java.
Java provides several different types of garbage collectors, each designed to meet specific requirements and performance objectives. Some of the commonly used garbage collectors include:
- Serial: Suitable for simple applications with small heaps, where pause times are not a concern.
- Parallel: Ideal for multiprocessor systems, as it utilizes multiple threads to perform garbage collection.
- CMS (Concurrent Mark Sweep): Aimed at reducing latency by performing garbage collection concurrently with application execution.
- G1 (Garbage First): Offers consistent pause times by dividing the heap into smaller regions and performing collections incrementally.
- ZGC (Z Garbage Collector): Optimized for low latency and scalability by conducting concurrent garbage collection without stopping the application.
For more detailed information on each garbage collector, refer to the official Java documentation.
When and Why to Tune the Garbage Collector
There are various situations where tuning the garbage collector becomes necessary. Here are a few common scenarios:
-
Reducing Latency: In real-time or high-performance applications, reducing pause times caused by garbage collection is crucial. Tuning the garbage collector can help minimize these pauses and improve the overall responsiveness of the application.
-
Increasing Throughput: For applications that prioritize throughput, optimizing the garbage collector can help achieve higher execution rates and maximize processing capacity.
-
Managing Memory Footprint: Some applications require efficient memory utilization to handle large datasets or limited resources. By tuning the garbage collector, developers can control the memory footprint and prevent excessive memory usage.
The specific goals of garbage collector tuning may vary depending on the application requirements and performance objectives.
Basic Concepts of GC Tuning
To effectively tune the garbage collector, it is essential to understand some key concepts and terminology related to garbage collection.
Heap
The heap is a region of memory where objects are dynamically allocated. It consists of young and old generations. The young generation is further divided into Eden space and survivor spaces.
Generational Spaces
Generational spaces are used to manage objects based on their age. New objects are allocated in the young generation, and if they survive a certain number of garbage collections, they are promoted to the old generation.
Minor and Major Collections
Minor collections occur in the young generation and are responsible for reclaiming short-lived objects. These collections are usually fast and don't cause significant pauses. Major collections, on the other hand, occur in the old generation and involve scanning a larger portion of the heap. They typically take longer and result in longer pauses.
When tuning the garbage collector, various Java Virtual Machine (JVM) flags can be used. These flags control different aspects of garbage collection behavior. Let's take a look at a couple of examples:
-Xms1024m -Xmx1024m
-XX:NewRatio=3
In the above example, we have set the initial heap size (-Xms
) and maximum heap size (-Xmx
) to be 1024 MB. Specifying these values can prevent time-costly heap resizing operations during the application's execution.
The -XX:NewRatio
flag determines the ratio between the young and old generation in the heap. In this case, we have set it to 3, indicating that the old generation will be three times the size of the young generation.
By adjusting these flags, developers can control the memory allocation and improve the performance of the garbage collector.
Analyzing Garbage Collection Performance
To effectively tune the garbage collector, it is crucial to analyze its performance. Java provides various tools and techniques for monitoring and logging garbage collection activity.
One common approach is to enable garbage collection logging using the -Xloggc
flag. This flag directs the garbage collection logs to a file, which can be later analyzed. Additionally, multiple GC logging tools are available, such as VisualVM, JConsole, and Oracle's Mission Control, which offer rich insights into garbage collection behavior.
When analyzing the logs, it is important to pay attention to metrics such as pause times, frequency of collections, and memory usage patterns. Long pause times can negatively impact application responsiveness, while frequent collections can reduce throughput.
Here is an example of a GC log entry:
[GC pause(14.76897 secs), 0.0740108 secs]
[Times: user=0.25 sys=0.03, real=1.46 secs]
In this log entry, we can observe a garbage collection pause of approximately 14.77 seconds. The user
, sys
, and real
times provide insights into the CPU and wall-clock time spent during the pause.
Advanced Tuning Techniques
In addition to the basic concepts, there are advanced techniques that can further optimize garbage collection performance. Let's explore a couple of them.
Adjusting Parallel GC Threads
The -XX:ParallelGCThreads
flag allows developers to adjust the number of threads used by the parallel garbage collector. By setting this flag to match the number of processor cores, developers can maximize parallel garbage collection performance.
Here is an example of setting the parallel GC threads to match the number of cores:
-XX:ParallelGCThreads=8
Setting the threads to match the number of cores ensures efficient utilization of CPU resources during garbage collection.
Tweaking Survivor Spaces
Survivor spaces are regions within the young generation where objects that have survived minor collections are promoted. The -XX:SurvivorRatio
flag can be used to adjust the ratio between the Eden space and the survivor spaces.
For example:
-XX:SurvivorRatio=6
In this example, the survivor spaces will be six times smaller than the Eden space.
By carefully adjusting the survivor space ratio, developers can influence the behavior of minor collections and optimize memory management.
Case Studies: Real-World GC Tuning
To illustrate the impact of garbage collector tuning, let's consider a couple of real-world case studies.
Case Study 1: Web Application
In a web application with a large number of concurrent users, the default garbage collector was causing frequent pauses, affecting the overall response time. By tuning the garbage collector to prioritize low-latency collections with shorter pauses, the application's responsiveness improved significantly. The average pause time reduced by 75%, resulting in a noticeable improvement in user experience.
Case Study 2: Big Data Processing
A data processing application that dealt with large datasets was experiencing frequent heap exhaustion due to inefficient memory utilization. By tuning the garbage collector to better manage memory footprint and prioritize memory reclamation, the application's memory usage reduced by 50%. This optimization enabled the application to handle larger datasets and run more efficiently within the available resources.
For more detailed case studies and in-depth analysis of garbage collector tuning, refer to the following resources:
- Case Study: Garbage Collection Tuning in a Real-Time System
- Tuning Java Garbage Collection for Low Latency at Twitter
Closing the Chapter
Garbage collector tuning is a valuable technique for optimizing Java application speed. By understanding the concepts and techniques of garbage collection tuning, developers can significantly improve the performance of their Java applications.
Throughout this article, we have explored the importance of garbage collector tuning, the different types of garbage collectors available in Java, and the basic and advanced techniques for optimizing garbage collection performance. We have also discussed real-world case studies that highlight the benefits of garbage collector tuning in improving Java application speed.
To become proficient in garbage collector tuning, developers are encouraged to experiment with the provided tips and further expand their knowledge through practice and additional resources. By actively tuning the garbage collector, developers can unlock the full potential of their Java applications.
Further Resources
- Java SE 14 Garbage Collection Tuning: Official Java documentation on garbage collection tuning.
- Java Performance: The Definitive Guide: A comprehensive guide to Java performance tuning, including garbage collection optimization.
- Garbage Collection Handbook: The Art of Automatic Memory Management: A comprehensive reference on garbage collection algorithms and techniques.
- Java Garbage Collection and Performance Optimization: An online course on garbage collection and performance optimization in Java applications.