Boost Your Spring MVC: Mastering Advanced Session Tricks
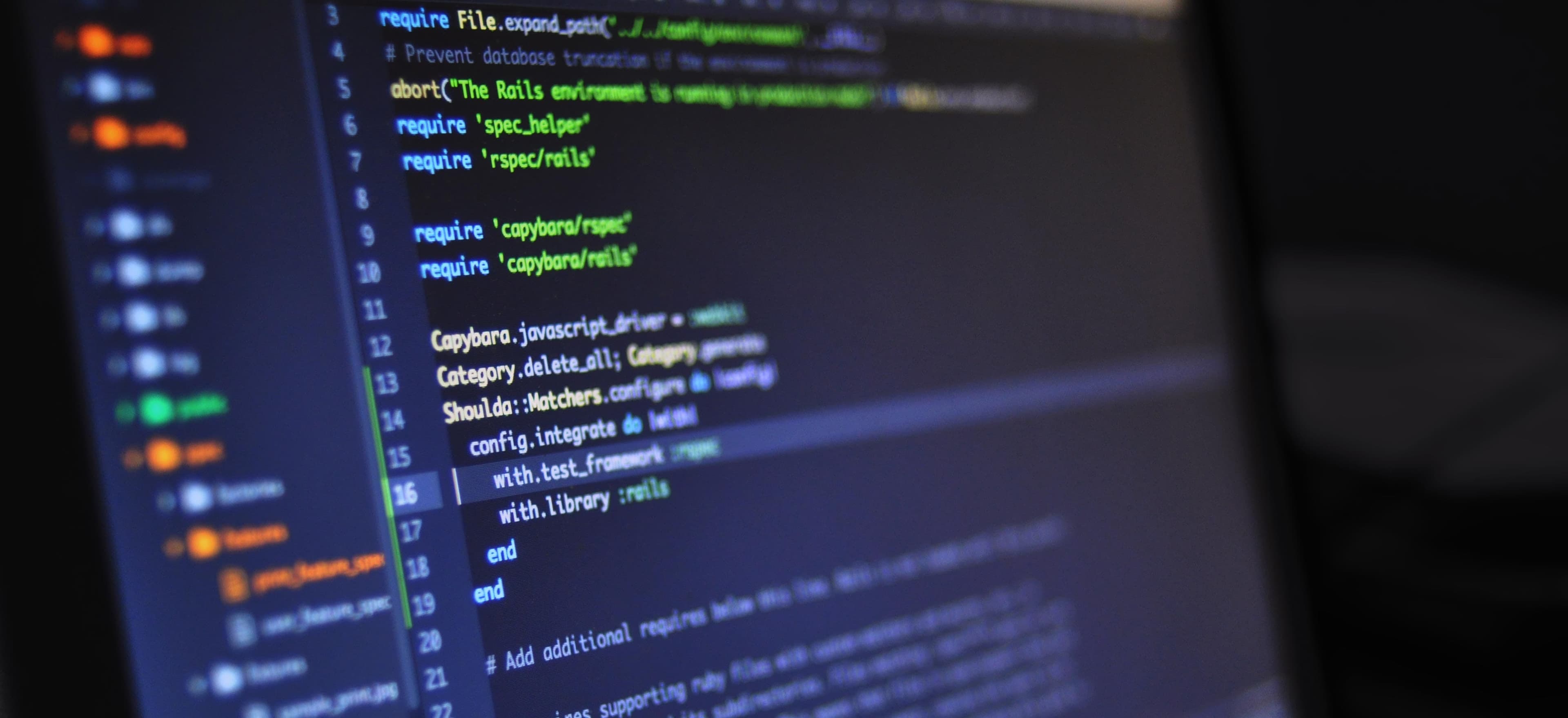
- Published on
Boost Your Spring MVC: Mastering Advanced Session Tricks
Spring MVC is a powerful framework that allows developers to build robust and scalable web applications with ease. One of its key features is session management, which enables the storage and retrieval of user-specific data across multiple requests. In this blog post, we will explore some advanced session tricks in Spring MVC that will help you take your application to the next level.
Understanding Spring MVC Session Management
Before diving into the advanced techniques, let's quickly recap how session management works in Spring MVC. When a user accesses your application, a session is created to store their data. This session is identified by a unique session ID, which is typically stored in a cookie or appended to the URL.
By default, Spring MVC uses an implementation of the HttpSession
interface provided by the underlying servlet container (e.g., Tomcat or Jetty) to manage sessions. This interface provides methods for storing and retrieving data in the session.
1. Storing Objects in the Session
In many cases, you may need to store complex objects in the session instead of simple data types like strings or numbers. To do this, you can use the @SessionAttribute
annotation in your controller methods.
@GetMapping("/user")
public String getUser(@SessionAttribute("loggedInUser") User user) {
// Your logic here
}
In the example above, the @SessionAttribute
annotation retrieves the loggedInUser
attribute from the session and binds it to the user
parameter of the getUser
method. This allows you to access the user object throughout the request handling process.
2. Controlling Session Timeout
Session timeout is an important aspect of session management, as it determines how long a session will remain active after a user becomes inactive. By default, Spring MVC uses the timeout value specified in the servlet container configuration.
However, you can override this value using the HttpSession.setMaxInactiveInterval()
method. This method takes the session timeout value in seconds. For example, to set the session timeout to 30 minutes, you can use the following code:
@GetMapping("/setSessionTimeout")
public String setSessionTimeout(HttpSession session) {
session.setMaxInactiveInterval(1800);
// Your logic here
}
3. Invalidating Sessions
There may be scenarios where you need to manually invalidate a session, such as when a user logs out or after a certain period of inactivity. To do this, you can use the HttpSession.invalidate()
method.
@GetMapping("/logout")
public String logout(HttpSession session) {
session.invalidate();
// Your logic here
}
By calling session.invalidate()
, the session associated with the current user will be destroyed, and a new session will be created when they access your application again.
4. Using Session Scoped Beans
Spring MVC allows you to define beans with session scope, which means that a new instance of the bean will be created for each user session. This can be useful when you need to maintain stateful data specific to each user.
To define a session-scoped bean, you can use the @Scope(value = "session")
annotation on your bean class.
@Scope(value = "session")
@Component
public class ShoppingCart {
// Your logic here
}
In the example above, an instance of the ShoppingCart
class will be created for each user session. You can then inject this bean into your controllers or other beans as needed.
5. Cross-Site Request Forgery (CSRF) Protection
Cross-site request forgery (CSRF) is a type of attack where an unauthorized user tricks a victim into performing actions on a website without their consent. To protect against CSRF attacks, Spring MVC provides built-in support through the CsrfToken
class.
In your controller, you can inject the CsrfToken
object and include it in your response to ensure that subsequent requests are properly authenticated.
@GetMapping("/user")
public String getUser(CsrfToken csrfToken) {
// Your logic here
}
By including the CsrfToken
in your response, Spring MVC will automatically validate it on subsequent requests, preventing CSRF attacks.
The Closing Argument
Session management is a crucial aspect of any web application, and Spring MVC provides a rich set of features to handle it effectively. In this blog post, we explored some advanced session tricks that will help you enhance the security and functionality of your Spring MVC application.
By leveraging features like storing objects in the session, controlling session timeouts, invalidating sessions, using session-scoped beans, and implementing CSRF protection, you can take your Spring MVC application to the next level.
Get started with these advanced session tricks and let us know how they work for you and if you have any other tricks up your sleeve.
To learn more about Spring MVC and session management, check out the official Spring Framework documentation. Additionally, the Spring Security project provides comprehensive security features for Spring applications, including session management.