Java Streams: Mastering Incremental CSV File Reads
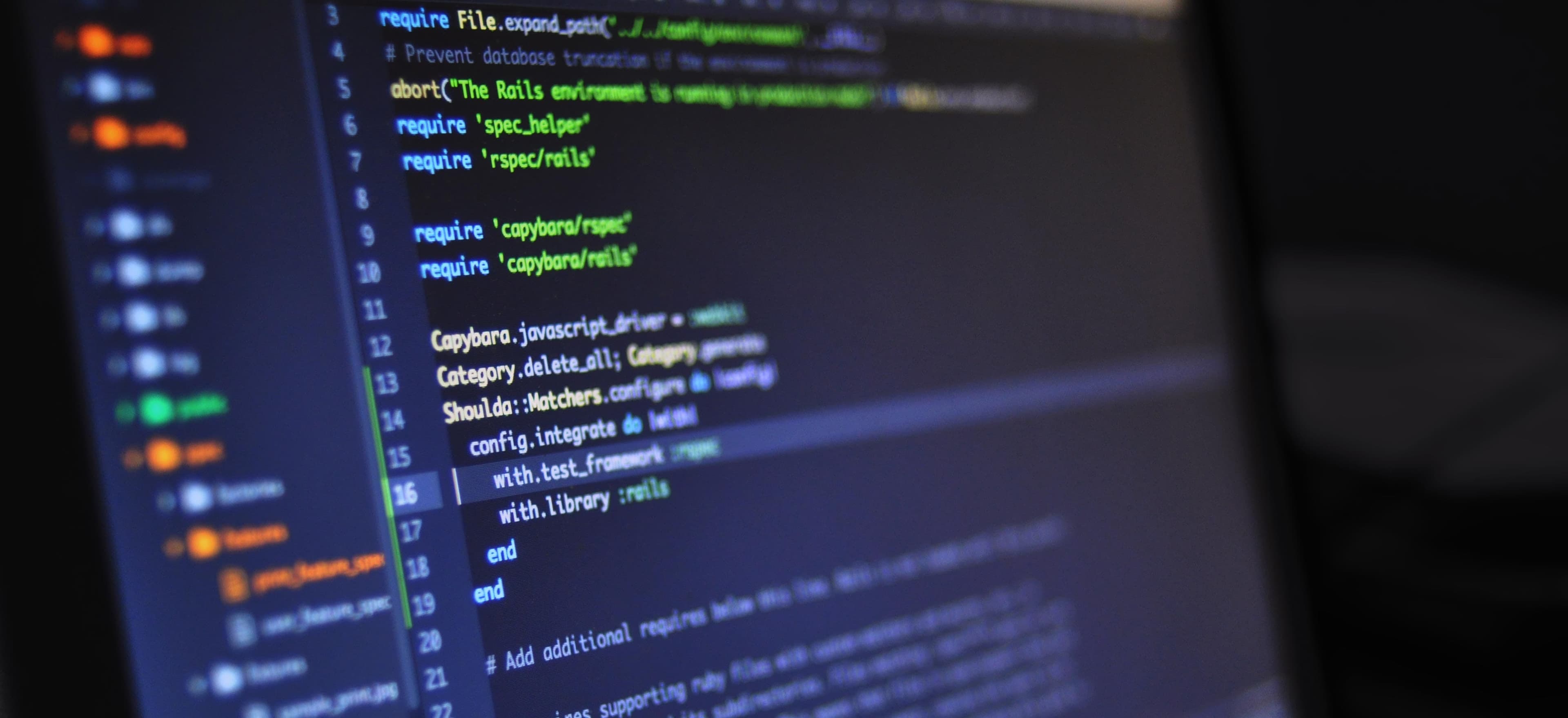
- Published on
Java Streams: Mastering Incremental CSV File Reads
CSV files are a staple in the world of programming – a simple, succinct format for data representation. Whether you're dealing with configuration data, exporting reports, or handling bulk data imports, understanding how to process CSV files efficiently in Java can make or break your application's performance.
In this expert guide, we’ll take a deep dive into the world of incremental CSV file reading using Java's Stream API to ensure optimum memory management and speed. We aim to strike a perfect balance between detailed explanations and actionable code snippets, a must-read for the savvy Java developer.
Understanding Streams and Their Potential
Java 8 introduced the Stream API, a powerful tool that revolutionized how we process collections of data. Streams provide a high-level abstraction for sequential and parallel data operations using functional style programming. A critical aspect of streams is their ability to facilitate incremental processing – reading and processing one element at a time, rather than holding an entire collection in memory.
This characteristic is especially beneficial when dealing with large CSV files. Instead of loading the entire file into memory, we can read and process entries one row at a time, dramatically reducing our application's memory footprint.
Reading CSV Files Incrementally
Reading a CSV file incrementally in Java can be accomplished by combining the Files.lines
method with the Stream API. The Files.lines
method reads the file line by line lazily, which means that the line is read and processed only when it's needed.
Here's a basic outline of what we're going to do:
- Open a CSV file as a
Stream
of strings, each representing a row. - Parse each row into a more usable form (like a custom object).
- Process the data incrementally to reduce memory usage.
Let's start by creating our model. Imagine we have a CSV file representing user data with columns: id
, name
, and email
.
public class User {
private Long id;
private String name;
private String email;
// Constructors, getters, setters, and toString
}
The Incremental Read Code
Here's a step-by-step example of how to read a CSV file incrementally:
import java.nio.file.*;
import java.util.stream.Stream;
public class CsvReader {
public void readCsvIncrementally(Path pathToCsv) {
try (Stream<String> lines = Files.lines(pathToCsv)) {
lines
.skip(1) // skip the header row
.map(this::parseCsvRow)
.forEach(System.out::println); // process each row
} catch (Exception e) {
e.printStackTrace();
}
}
private User parseCsvRow(String row) {
String[] columns = row.split(",");
return new User(
Long.parseLong(columns[0]),
columns[1],
columns[2]
);
}
}
Breaking Down the Code:
- We open the CSV file using
Files.lines
, which returns aStream<String>
with each element being one line in the file. - The
skip(1)
method is employed to ignore the header row of the CSV file. - We transform each
String
line into aUser
object through themap
operation. - Finally, we perform an action on each
User
object, which in this case is printing it to the console.
The Power of Laziness
The beauty of this approach lies in the laziness of the Stream API. Each line is read and processed one by one, which means you can read files far larger than your available memory without running into OutOfMemoryError
.
Let's say we only want to process users with an email from a specific domain. Streams make this incredibly simple with the filter
method:
lines
.skip(1)
.map(this::parseCsvRow)
.filter(user -> user.getEmail().endsWith("@example.com"))
.forEach(System.out::println);
Just by adding the filter
line, we narrow the processed data to exactly what we need.
Advanced Processing with Java Streams
The Stream API provides numerous methods for more complex operations, such as reduce
, collect
, and flatMap
. With collect
, you can gather your processed data into collections like Lists or Maps. Let's see an example:
import java.util.List;
import java.util.stream.Collectors;
public List<User> filterAndCollectUsers(Path pathToCsv, String domain) {
try (Stream<String> lines = Files.lines(pathToCsv)) {
return lines
.skip(1)
.map(this::parseCsvRow)
.filter(user -> user.getEmail().endsWith(domain))
.collect(Collectors.toList());
} catch (Exception e) {
e.printStackTrace();
}
return Collections.emptyList();
}
In this snippet, collect
gathers the filtered users into a List
, which we can use further in the application.
When to Use Parallel Streams
Parallel streams can expedite the processing of large CSV files by utilizing multiple threads. However, this comes with overheads and doesn't always speed up the operation. It's best for computationally expensive tasks where the cost of processing each element is high compared to the cost of splitting the data among threads.
For simple tasks like parsing CSV rows, parallel streams may not provide a performance benefit and could even complicate things because of thread management and synchronization costs.
Handling Exceptions Gracefully
Working with files means anticipating I/O errors. When using Streams, you can handle exceptions with a try-catch block as we've done in the examples. For more granular control, consider implementing custom exception handling within the stream operations.
Best Practices and Optimization
When dealing with large CSV files, you'll want to adhere to some best practices to maximize performance:
- Always use buffered reads (
Files.newBufferedReader
, combined withlines()
) to minimize I/O operations. - Reuse expensive resources like
Pattern
instances for regular expressions. - Carefully consider when to use parallel streams.
- Profile your application to identify bottlenecks.
- Close resources properly to avoid leaks – the try-with-resources statement in the examples ensures this.
Key Takeaways
Incremental CSV file reading using Java's Stream API is a powerful technique that every Java developer should have in their toolkit. Leveraging the power of laziness, streams enable you to work with large datasets in a memory-efficient manner, transforming and filtering data on the fly.
By understanding and utilizing the principles and code examples provided in this post, you’ll be well-equipped to handle CSV files gracefully in your Java applications.
For further reading, the official Java documentation provides great insights into the Stream API. Also, consider diving into more advanced topics like Java's new Date and Time API or exploring concurrency in Java.
Happy coding, and may your data always be in the right stream!