Securing RESTful APIs: Spring Security Auth Pitfalls
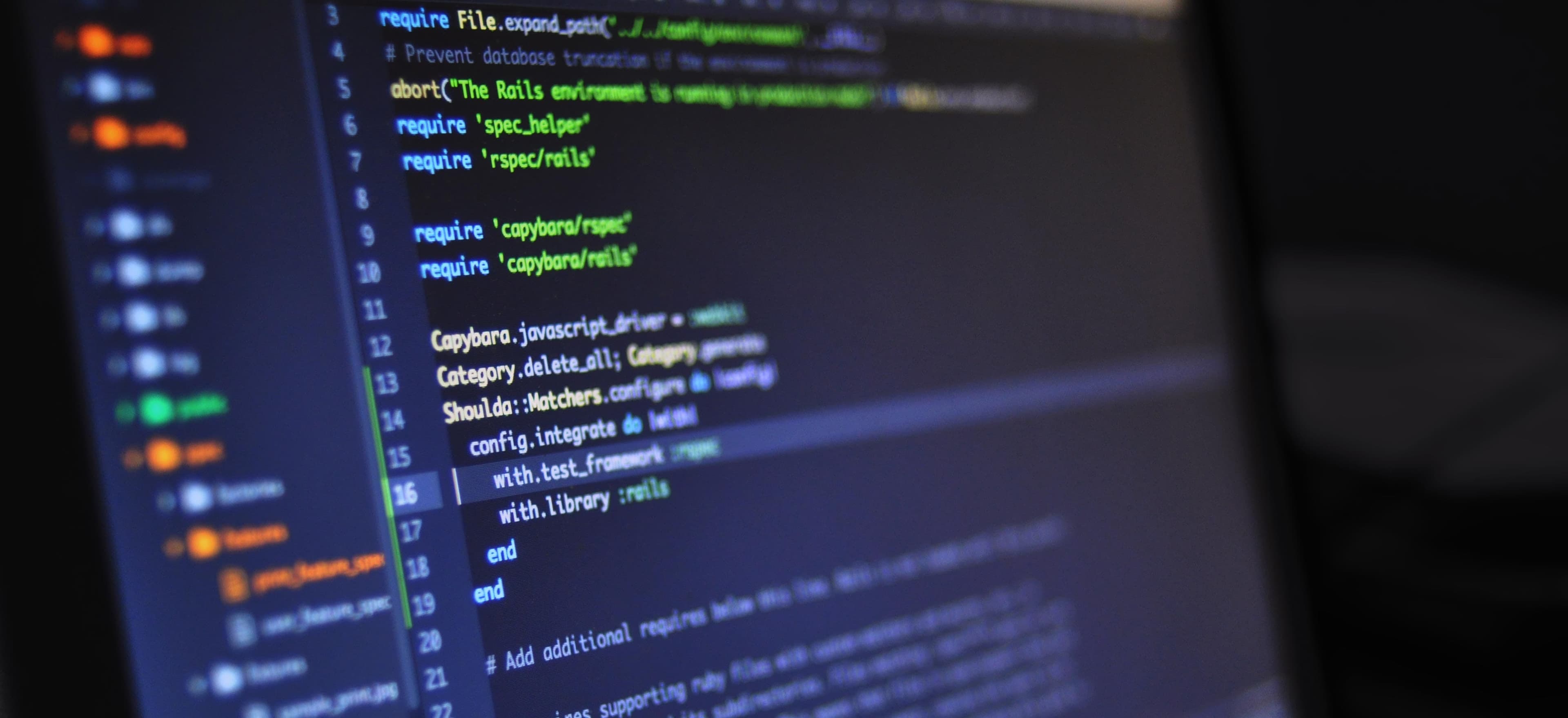
- Published on
Securing RESTful APIs: Spring Security Auth Pitfalls
Since the rise of web services and APIs, securing these endpoints has become a critical concern. RESTful APIs are increasingly being used by applications to exchange data, and it is essential to protect them from unauthorized access and potential attacks. Spring Security is a popular framework in the Java ecosystem for securing web applications and API endpoints. In this blog post, we will discuss some common authentication pitfalls in Spring Security when securing RESTful APIs and explore ways to overcome them.
1. Lack of Authentication
One of the most common pitfalls is not implementing authentication at all. It is crucial to verify the identity of the user or client requesting access to the API. Without authentication, any user or attacker can make requests and potentially access or modify sensitive data.
To implement authentication in Spring Security, we can use various mechanisms such as Basic Authentication, OAuth2, JWT (JSON Web Tokens), or even custom authentication providers. The choice of authentication mechanism depends on the specific requirements of your application.
2. Weak Passwords
Another common pitfall is allowing weak passwords, which can be easily guessed or cracked by attackers. Weak passwords are inherently insecure and can lead to unauthorized access to the API.
To address this issue, Spring Security provides password encoding and hashing mechanisms. When storing user passwords, it is crucial to hash them using a strong cryptographic algorithm, such as bcrypt or Argon2. This way, even if the password hashes are compromised, it will be challenging to reverse engineer the original password.
Here's an example of how to configure password encoding in Spring Security:
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
In this example, we are using the BCryptPasswordEncoder
to hash passwords. This bean can then be used by Spring Security to encode and match passwords during the authentication process.
3. Insecure Communication
Securing the communication channel between the client and the API server is crucial to prevent eavesdropping and data tampering. Using HTTPS (HTTP over SSL/TLS) encrypts the communication and ensures data integrity.
To enable HTTPS in Spring Boot, we need to configure an SSL certificate and configure the server to use HTTPS. This can be done by providing the SSL certificate and private key files, or by using a self-signed certificate for development purposes.
Here's an example of how to configure HTTPS in a Spring Boot application:
server:
port: 8443
ssl:
enabled: true
key-store: classpath:keystore.p12
key-store-password: mypassword
key-store-type: PKCS12
key-alias: myalias
In this example, we are configuring the server to use HTTPS on port 8443. We provide the path to the keystore file containing the SSL certificate and the necessary password to access it. Finally, we specify the alias for the certificate.
4. Lack of CSRF Protection
Cross-Site Request Forgery (CSRF) is an attack where an attacker tricks a user into performing unintended actions on a website or API. To prevent CSRF attacks, Spring Security provides built-in support for CSRF protection.
CSRF protection in Spring Security is enabled by default for most HTTP methods. It adds a state-changing token to each request, and the client must include this token in subsequent requests to prove its authenticity.
To enable CSRF protection in Spring Security, we can add the following configuration:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse());
}
}
In this example, we configure the HttpSecurity
object to use the CookieCsrfTokenRepository
. This repository stores the CSRF token in a cookie, and the client must send this token in subsequent requests.
5. Missing Authorization
Authentication is about verifying the identity of a user or client, while authorization is about determining what actions and resources a particular user is allowed to access. It is essential to enforce fine-grained access control to protect sensitive data and resources.
Spring Security provides a flexible and powerful authorization mechanism based on roles, permissions, and expression-based access control. We can annotate controller methods or configure URL patterns to restrict access based on roles or custom access expressions.
Here's an example of how to apply method-level security in Spring Security:
@RestController
@RequestMapping("/api")
public class ApiController {
@PreAuthorize("hasRole('ADMIN')")
@GetMapping("/admin")
public String adminOnly() {
return "Only admins can access this resource";
}
@PreAuthorize("hasRole('USER')")
@GetMapping("/user")
public String userOnly() {
return "This resource is accessible to authenticated users";
}
}
In this example, we use the @PreAuthorize
annotation to restrict access to specific methods based on user roles.
To Wrap Things Up
Securing RESTful APIs is a crucial aspect of building modern web applications. In this blog post, we discussed some common pitfalls in authentication when using Spring Security to secure API endpoints. We explored topics such as lack of authentication, weak passwords, insecure communication, lack of CSRF protection, and missing authorization.
By addressing these pitfalls and implementing appropriate security measures, we can ensure the integrity, confidentiality, and availability of our RESTful APIs. Spring Security provides a robust set of features and configurations to build secure and resilient applications. Remember to stay vigilant and regularly review and update your security measures as new threats and vulnerabilities emerge.
If you are looking for more information on Spring Security, I recommend checking out the official Spring Security documentation and the Baeldung Spring Security tutorials. These resources provide in-depth explanations, examples, and best practices for securing your applications.