Dockerfile 101: Solving Common Syntax Hang-ups!
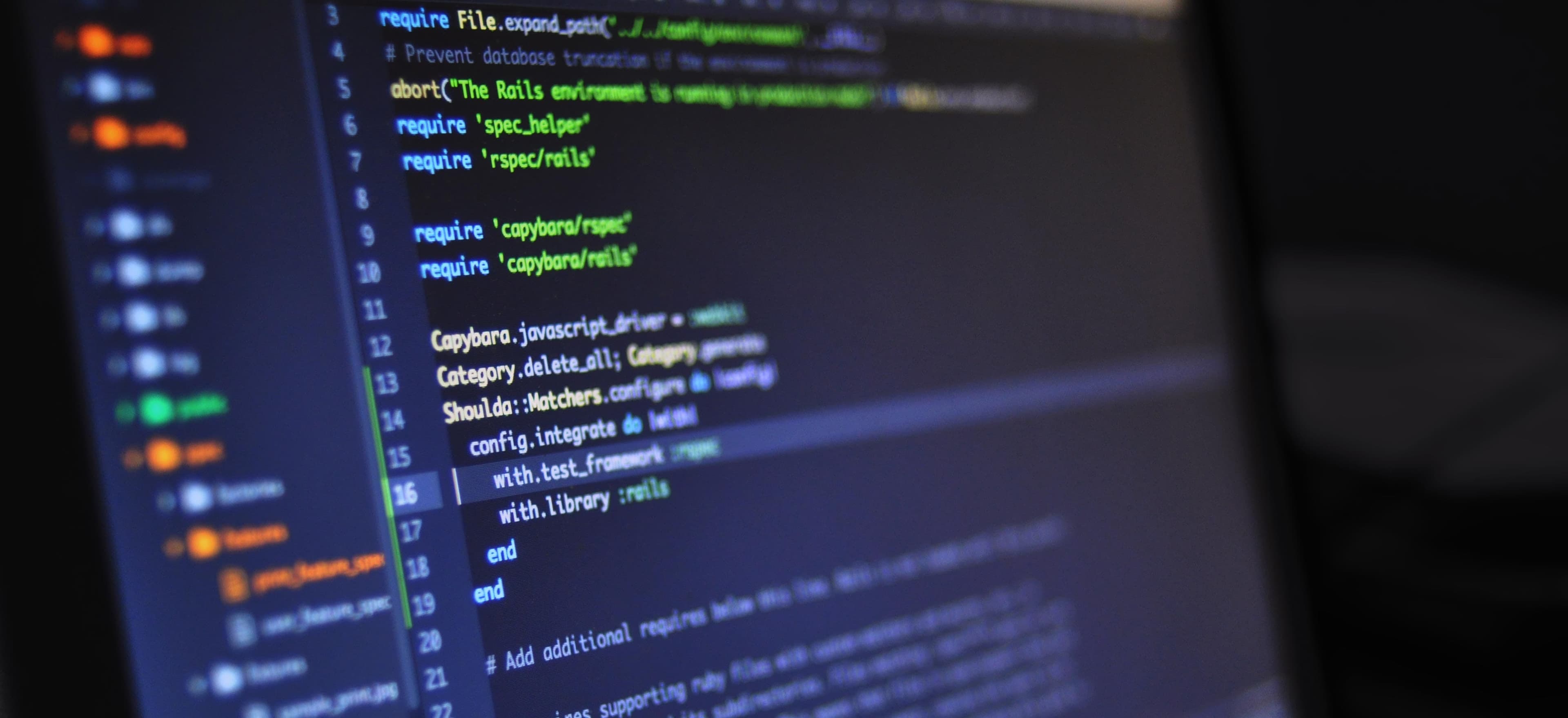
- Published on
Dockerfile 101: Solving Common Syntax Hang-ups!
Have you ever found yourself stuck while writing a Dockerfile? Don't worry, you're not alone. Dockerfiles can be a bit tricky to get right, especially for beginners. But fear not, in this article, we will discuss some common syntax hang-ups and how to overcome them. By the end, you will have a better understanding of Dockerfile syntax and be able to confidently write your own Dockerfiles.
What is a Dockerfile?
A Dockerfile is a text file that contains a set of instructions that Docker uses to build a Docker image. It provides a simple and declarative way to define the environment and dependencies required by your application. With a Dockerfile, you can automate the process of building and deploying your applications in a consistent and reproducible manner.
Understanding the Syntax
Before we dive into the common syntax hang-ups, let's quickly go over the basic structure of a Dockerfile. A Dockerfile consists of a series of instructions, each starting with a keyword followed by arguments. Here's a simple example:
# Use an existing base image
FROM ubuntu:latest
# Run a command inside the container
RUN apt-get update && apt-get install -y curl
# Set the working directory
WORKDIR /app
# Copy files from the host to the container
COPY . .
# Expose a port
EXPOSE 8080
# Define the command to run when the container starts
CMD ["python", "app.py"]
In this example, we start with a base image ubuntu:latest
, update the package lists, install curl
, set the working directory to /app
, copy the files from the host to the container, expose port 8080
, and finally define the command to run when the container starts.
Common Syntax Hang-ups
Now that we have a basic understanding of the Dockerfile syntax, let's discuss some common hang-ups that beginners often encounter.
1. Missing or Incorrect Keywords
One of the most common mistakes is missing or using incorrect keywords. The keywords in a Dockerfile are case-insensitive, but it's best practice to use uppercase letters to make them stand out. For example, instead of using from
or FroM
, use FROM
. Similarly, run
should be RUN
, copy
should be COPY
, and so on.
2. Misplaced Instructions
The order of instructions in a Dockerfile matters. For example, if you try to set the working directory before copying the files, you will get an error because the directory doesn't exist yet. Make sure to define the instructions in the correct order based on their dependencies. For instance, if you want to install dependencies using RUN
, it should come before COPY
.
3. Incorrect Arguments
Each instruction in a Dockerfile has its own set of arguments. It's important to use the correct arguments for each instruction. For example, COPY
requires two arguments - the source and destination. Similarly, EXPOSE
requires one argument - the port number. Always refer to the Docker documentation for the correct arguments for each instruction.
4. Forgetting to End Instructions
Each instruction in a Dockerfile needs to be properly terminated. Most instructions end with a newline character, but some instructions like RUN
can span multiple lines using the backslash \
at the end of each line. Make sure to properly terminate all instructions to avoid syntax errors.
5. Incorrect Use of Environment Variables
Environment variables are a powerful feature in Docker, but they can be a source of confusion if not used correctly. To use environment variables in a Dockerfile, you can use the ENV
instruction. However, keep in mind that environment variables set with ENV
are only available during the build process and not at runtime. To use environment variables at runtime, you need to use the -e
flag when running the container or use a .env
file with docker-compose
.
6. Not Cleaning Up After Each Step
Docker build caches each instruction in the Dockerfile. If you make a change to an instruction and rebuild the image, Docker will use the cached result of the previous instruction. This can lead to unexpected behavior and make debugging difficult. To avoid this, it's a good practice to clean up after each step to ensure that each instruction is executed from scratch. You can use the --no-cache
flag with docker build
to force a clean build.
Best Practices
To avoid syntax hang-ups and write maintainable Dockerfiles, here are some best practices to follow:
- Use descriptive comments to explain what each instruction does.
- Pin the version of the base image to ensure reproducibility.
- Use multi-stage builds to minimize the size of the final image.
- Minimize the number of layers in your image by combining multiple instructions into a single
RUN
statement. - Use
.dockerignore
to exclude unnecessary files and directories from the build context. - Avoid running commands as root in the container. Instead, create a non-root user and use it for running your application.
By following these best practices, you can write clean and manageable Dockerfiles that are easy to understand and maintain.
Lessons Learned
Writing Dockerfiles can sometimes be challenging, especially for beginners. However, with a solid understanding of the syntax and by following best practices, you can avoid common hang-ups and write Dockerfiles like a pro. Remember to pay attention to keywords, instructions order, arguments, and properly terminate instructions. Additionally, keep in mind the correct usage of environment variables and ways to clean up after each step. By adhering to these guidelines, you can build Docker images efficiently and consistently.
Now that you have learned how to overcome common syntax hang-ups in Dockerfiles, go ahead and put your newfound knowledge into practice. Happy Dockerizing!
Further reading: